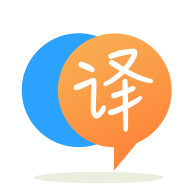
[英]How to repeat the question after the user chose the wrong answer on my quiz program in java
[英]How to add the option for the user to repeat this Java program AFTER using it?
我整個晚上都在參加這個節目,我只需要添加一件事,但我正在摸索如何去做。 我 go 如何在用戶已經完成一次程序后添加重復該程序的選項? 我有一個選項供用戶輸入他們是否想首先使用它,Y 運行程序,然后 N 結束它。 如果用戶已經運行過它,我只需要一些幫助將程序返回到 go 回到最開始,或者如果他們只想退出則再次退出。 謝謝你。 很抱歉我的代碼不是最漂亮的。 我是一個初學者。
import java.util.Scanner;
public class BarChart
{
public static void main(String[] args)
{
int store1, store2, store3, store4, store5;
String answer;
Scanner keyboard = new Scanner(System.in);
System.out.println("This program will display a bar chart " +
" comprised of astericks based on five different stores' " +
"sales today. 1 asterick = $100 in sales.");
System.out.println("Would you like to use the program? "
+ "type 'Y' for yes or 'N' to exit the program.");
answer = keyboard.nextLine();
if(answer.equalsIgnoreCase("Y"))
{
System.out.print("Enter today's sales for store 1: ");
store1 = keyboard.nextInt();
while(store1 < 0)
{
System.out.println("Error! Sales cannot be less than 0.");
System.out.print("Enter today's sales for store 1: ");
store1 = keyboard.nextInt();
}
System.out.print("Enter today's sales for store 2: ");
store2 = keyboard.nextInt();
while(store2 < 0)
{
System.out.println("Error! Sales cannot be less than 0.");
System.out.print("Enter today's sales for store 2: ");
store2 = keyboard.nextInt();
}
System.out.print("Enter today's sales for store 3: ");
store3 = keyboard.nextInt();
while(store3 < 0)
{
System.out.println("Error! Sales cannot be less than 0.");
System.out.print("Enter today's sales for store 3: ");
store3 = keyboard.nextInt();
}
System.out.print("Enter today's sales for store 4: ");
store4 = keyboard.nextInt();
while(store4 < 0)
{
System.out.println("Error! Sales cannot be less than 0.");
System.out.print("Enter today's sales for store 4: ");
store4 = keyboard.nextInt();
}
System.out.print("Enter today's sales for store 5: ");
store5 = keyboard.nextInt();
while(store5 < 0)
{
System.out.println("Error! Sales cannot be less than 0.");
System.out.print("Enter today's sales for store 5: ");
store5 = keyboard.nextInt();
}
System.out.println("Sales \t Bar Chart");
System.out.println("----- \t --------------------------");
System.out.print("Store 1: ");
for (int num = 0; num < store1; num += 100)
{
System.out.print("*");
}
System.out.print("\nStore 2: ");
for (int num = 0; num < store2; num += 100)
{
System.out.print("*");
}
System.out.print("\nStore 3: ");
for (int num = 0; num < store3; num += 100)
{
System.out.print("*");
}
System.out.print("\nStore 4: ");
for (int num = 0; num < store4; num += 100)
{
System.out.print("*");
}
System.out.print("\nStore 5: ");
for (int num = 0; num < store5; num += 100)
{
System.out.print("*");
}
}
if(answer.equalsIgnoreCase("N"))
System.out.print("Program terminated. Have a nice day!");
}
}
我試過重新定向循環,但這只會破壞我的程序。
您目前擁有:
System.out.println("Would you like to use the program? "
+ "type 'Y' for yes or 'N' to exit the program.");
answer = keyboard.nextLine();
if(answer.equalsIgnoreCase("Y")) {
// "the program"
}
if(answer.equalsIgnoreCase("N"))
System.out.print("Program terminated. Have a nice day!");
將其更改為:
while (true) {
// "the program"
System.out.println("Would you like to use the program again? "
+ "type 'Y' for yes or anything else to exit the program.");
answer = keyboard.nextLine();
if (!answer.equalsIgnoreCase("Y")) {
System.out.print("Program terminated. Have a nice day!");
break;
}
}
while(true)
永遠循環直到執行break
。
當啟動程序的答案為“是”時,您應該使用循環遍歷代碼。 如果答案是否定的,我們應該跳出循環。 這是一個工作代碼:
public static void main(String[] args) {
int store1, store2, store3, store4, store5;
String answer;
Scanner keyboard = new Scanner(System.in);
System.out.println("This program will display a bar chart " +
" comprised of astericks based on five different stores' " +
"sales today. 1 asterick = $100 in sales.");
System.out.println("Would you like to use the program? "
+ "type 'Y' for yes or 'N' to exit the program.");
answer = keyboard.nextLine();
while (answer.equalsIgnoreCase("Y")) {
System.out.print("Enter today's sales for store 1: ");
store1 = keyboard.nextInt();
while (store1 < 0) {
System.out.println("Error! Sales cannot be less than 0.");
System.out.print("Enter today's sales for store 1: ");
store1 = keyboard.nextInt();
}
System.out.print("Enter today's sales for store 2: ");
store2 = keyboard.nextInt();
while (store2 < 0) {
System.out.println("Error! Sales cannot be less than 0.");
System.out.print("Enter today's sales for store 2: ");
store2 = keyboard.nextInt();
}
System.out.print("Enter today's sales for store 3: ");
store3 = keyboard.nextInt();
while (store3 < 0) {
System.out.println("Error! Sales cannot be less than 0.");
System.out.print("Enter today's sales for store 3: ");
store3 = keyboard.nextInt();
}
System.out.print("Enter today's sales for store 4: ");
store4 = keyboard.nextInt();
while (store4 < 0) {
System.out.println("Error! Sales cannot be less than 0.");
System.out.print("Enter today's sales for store 4: ");
store4 = keyboard.nextInt();
}
System.out.print("Enter today's sales for store 5: ");
store5 = keyboard.nextInt();
while (store5 < 0) {
System.out.println("Error! Sales cannot be less than 0.");
System.out.print("Enter today's sales for store 5: ");
store5 = keyboard.nextInt();
}
System.out.println("Sales \t Bar Chart");
System.out.println("----- \t --------------------------");
System.out.print("Store 1: ");
for (int num = 0; num < store1; num += 100) {
System.out.print("*");
}
System.out.print("\nStore 2: ");
for (int num = 0; num < store2; num += 100) {
System.out.print("*");
}
System.out.print("\nStore 3: ");
for (int num = 0; num < store3; num += 100) {
System.out.print("*");
}
System.out.print("\nStore 4: ");
for (int num = 0; num < store4; num += 100) {
System.out.print("*");
}
System.out.print("\nStore 5: ");
for (int num = 0; num < store5; num += 100) {
System.out.print("*");
}
keyboard.nextLine();
System.out.println("");
System.out.println("Would you like to reuse the program? "
+ "type 'Y' for yes or 'N' to exit the program.");
answer = keyboard.nextLine();
}
if (answer.equalsIgnoreCase("N"))
System.out.print("Program terminated. Have a nice day!");
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.