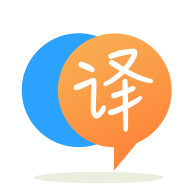
[英]A simple hashing-array-loop, I get error warnings even though it works, why is that?
[英]Why do I get these warnings even though the headers are included?
GCC 發出警告,這對我來說毫無意義。 我已經包含了所需的stdio
庫。 該程序的執行按預期工作,我在 EDX 上也獲得了 100% 的分數。 還有關於size 6
和size 5
的這些警告是什么?
到目前為止的代碼:
#include <stdio.h>
#include <stdlib.h>
struct student {
char name[50];
int age;
struct student *next;
};
struct student *createStudent(char studentName[50], int studentAge);
struct student * append(struct student * end, struct student * newStudptr);
void printStudents(struct student *start);
void copyStr(char [], char []);
/* add other prototypes here if needed*/
int main(void) {
struct student *start, *newStudptr, *end, *tmp;
int ageP, ageR, ageM;
scanf("%d %d %d", &ageP, &ageR, &ageM);
start = createStudent("Petra", ageP);
end = start;
newStudptr = createStudent("Remi", ageR);
end = append(end, newStudptr);
newStudptr = createStudent("Mike", ageM);
end = append(end, newStudptr);
printStudents(start);
tmp = start->next;
free(start);
start = tmp;
tmp = start->next;
free(start);
free(tmp);
return 0;
}
struct student* createStudent(char studentName[50], int studentAge) {
struct student* newStud;
newStud = (struct student*) malloc(sizeof(struct student));
copyStr(studentName, newStud->name);
newStud->age = studentAge;
newStud->next = NULL;
return newStud;
}
struct student* append(struct student* end, struct student* newStudPtr) {
end->next = newStudPtr;
end = newStudPtr;
return end;
}
void copyStr(char source[], char target[]) {
int i = 0;
while(source[i] != '\0') {
target[i] = source[i];
i++;
}
target[i] = '\0';
}
void printStudents(struct student* start) {
struct student* ptr = start;
while(ptr != NULL) {
printf("%s is %d years old.\n", ptr->name, ptr->age);
ptr = ptr->next;
}
}
/* Place your function definitions here. Be sure to include the definitions for
createStudent() and append() as well as any other functions you created for
the previous tasks. */
警告:
:!gcc -Wall -std=c17 edx_PrintLinkedList.c -o bin/edx_PrintLinkedList
edx_PrintLinkedList.c: In function ‘main’:
edx_PrintLinkedList.c:22:13: warning: ‘createStudent’ accessing 50 bytes in a region of size 6 [-Wstringop-overflow=]
22 | start = createStudent("Petra", ageP);
| ^~~~~~~~~~~~~~~~~~~~~~~~~~~~
edx_PrintLinkedList.c:22:13: note: referencing argument 1 of type ‘char *’
edx_PrintLinkedList.c:45:17: note: in a call to function ‘createStudent’
45 | struct student* createStudent(char studentName[50], int studentAge) {
| ^~~~~~~~~~~~~
edx_PrintLinkedList.c:25:18: warning: ‘createStudent’ accessing 50 bytes in a region of size 5 [-Wstringop-overflow=]
25 | newStudptr = createStudent("Remi", ageR);
| ^~~~~~~~~~~~~~~~~~~~~~~~~~~
edx_PrintLinkedList.c:25:18: note: referencing argument 1 of type ‘char *’
edx_PrintLinkedList.c:45:17: note: in a call to function ‘createStudent’
45 | struct student* createStudent(char studentName[50], int studentAge) {
| ^~~~~~~~~~~~~
edx_PrintLinkedList.c:28:18: warning: ‘createStudent’ accessing 50 bytes in a region of size 5 [-Wstringop-overflow=]
28 | newStudptr = createStudent("Mike", ageM);
| ^~~~~~~~~~~~~~~~~~~~~~~~~~~
edx_PrintLinkedList.c:28:18: note: referencing argument 1 of type ‘char *’
edx_PrintLinkedList.c:45:17: note: in a call to function ‘createStudent’
45 | struct student* createStudent(char studentName[50], int studentAge) {
我們不知道您可以更改哪些線路
但是 gcc 正在抱怨這個
你說
struct student* createStudent(char studentName[50], int studentAge)
但后來你做了
start = createStudent("Petra", ageP);
它試圖調用它作為
struct student* createStudent(char studentName[6], int studentAge)
function
你可以做
struct student* createStudent(char studentName[], int studentAge)
那可行
編輯
你也可以這樣修
char name[50];
....
strcpy(name, "Petra");
start = createStudent(name, ageP);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.