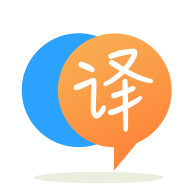
[英]cannot convert 'std::__cxx11::string {aka std::__cxx11::basic_string<char>}' to 'LPCSTR {aka const char*}'
[英]c++ cannot convert ‘std::string’ {aka ‘std::__cxx11::basic_string’} to ‘std::string (*)[3]’ {aka ‘std::__cxx11::basic_string (*)[3]’}
我目前正在嘗試在 c++ 中制作一個 tic tac toe AI。我目前正在制作一個 function 來檢查游戲的 winstate 是否正常,但是當我嘗試運行它時出現此錯誤:
61:34:錯誤:無法將“std::string”{又名“std::__cxx11::basic_string”}轉換為“std::string ( )[3]”{又名“std::__cxx11::basic_string ( )” [3]'} 61 | cout << HasWon(棋盤[3][3], 長度); | ~~~~~~~~~~^ | | | std::string {aka std::__cxx11::basic_string} main.cpp:6:19: 注意:初始化'int HasWon(std::string (*)[3], int)' 6 | 的參數 1 | int HasWon(string GameBoard[3][3], int boardLength); | ~~~~~~~^~~~~~~~~~~~~~~
我知道它與 char 和 strings 有關,但我不知道如何在我當前的程序中實現它。 繼承人的代碼:
#include <iostream>
using namespace std;
int AIMove(int PlayerMove);
int HasWon(string GameBoard[3][3], int boardLength);
int main () {
int Length = 3;
string Board[Length][Length] = {
{" ", " ", " "},
{" ", " ", " "},
{" ", " ", " "},
};
int NodeMap[Length][Length] = {
{0,0,0},
{0,0,0},
{0,0,0},
};
int NodeNumber = 1;
for(int h = Length - 1; h >= 0;h--) {
for(int d = 0; d < Length;d++) {
NodeMap[h][d] = NodeNumber;
NodeNumber++;
}
}
int Player;
bool HasMoved = false;
bool run = true;
while(run) {
//Prints Tic Tac Toe board to the screen
for(int h = 0; h < Length;h++) {
cout << " ";
for(int d = 0; d < Length;d++) {
cout << Board[h][d];
if(d < Length - 1) { cout <<"||"; }
}
if(h < Length - 1) { cout << "\n---------\n"; }
}
//Gets player input
cout << "\n choose a number 1-9 to place an X: ";
cin >> Player;
//Adds it to the board
HasMoved = false;
while(!HasMoved) {
for(int h = 0; h < Length; h++) {
for(int d = 0; d < Length; d++) {
if(NodeMap[h][d] == Player && Board[h][d] == " ") {
Board[h][d] = "X";
HasMoved = true;
}
}
}
if(!HasMoved) {
cout << "\n choose a number 1-9 to place an X: ";
cin >> Player;
}
}
cout << HasWon(Board[3][3], Length);
}
}
int AIMove(int PlayerMove, int boardLength);
//Checks if anyone has won yet. 0: nothing has happend, 1: tie, 2: X win, 3: O win.
int HasWon(string GameBoard[3][3], int boardLength) {
int xNumber = 0;
int oNumber = 0;
//Checks for vertical wins
for(int d = 0;d < boardLength;d++) {
xNumber = 0;
oNumber = 0;
for(int h = 0;h < boardLength;h++) {
if(GameBoard[h][d] == "X") { xNumber++; }
if(GameBoard[h][d] == "O") { oNumber++; }
if(xNumber == boardLength ) { return 2; }
if(oNumber == boardLength ) { return 3; }
}
}
//Checks for horizontial wins
for(int h = 0;h < boardLength;h++) {
xNumber = 0;
oNumber = 0;
for(int d = 0;d < boardLength;d++) {
if(GameBoard[h][d] == "X") { xNumber++; }
if(GameBoard[h][d] == "O") { oNumber++; }
if(xNumber == boardLength ) { return 2; }
if(oNumber == boardLength ) { return 3; }
}
}
//Checks for diagonal wins
xNumber = 0;
oNumber = 0;
for(int i = boardLength - 1;i >= 0;i--) {
if(GameBoard[i][i] == "X") { xNumber++; }
if(GameBoard[i][i] == "O") { oNumber++; }
if(xNumber == boardLength ) { return 2; }
if(oNumber == boardLength ) { return 3; }
}
xNumber = 0;
oNumber = 0;
for(int i = 0;i < boardLength;i++) {
if(GameBoard[i][i] == "X") { xNumber++; }
if(GameBoard[i][i] == "O") { oNumber++; }
if(xNumber == boardLength ) { return 2; }
if(oNumber == boardLength ) { return 3; }
}
//Checks for a tie
xNumber = 0;
oNumber = 0;
for(int h = 0;h < boardLength;h++) {
for(int d = 0;d < boardLength;d++) {
if(GameBoard[h][d] == "X") { xNumber++; }
if(GameBoard[h][d] == "O") { oNumber++; }
if(xNumber+oNumber == boardLength*boardLength) { return 1; }
}
}
return 0;
}
問題是當作為調用參數傳遞時,表達式Board[3][3]
的類型是std::string
而 function 參數GameBoard
的類型實際上是'std::string (*)[3]
。 這是因為string [3][3]
3] 由於類型衰減而衰減為string (*)[3]
。
因此,function HasWon
的參數類型與傳遞的參數不匹配。
另請注意,在標准 c++ 中,數組的大小必須是編譯時常量。 所以在你的代碼中:
int Length = 3;
string Board[Length][Length]; //this is not standard C++
聲明string Board[Length][Length];
不是標准的 C++ 因為Length
不是常量表達式。
要解決這個問題,您應該向Length
添加一個頂級const
並傳遞參數Board
而不是Board[3][3]
,如下所示:
//-vvvvv---------------------------> const added here
const int Length = 3;
//----------------vvvvv------------> changed to Board instead of Board[3][3]
cout << HasWon(Board, Length);
演示。
另一種選擇(更好)是使用std::vector
。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.