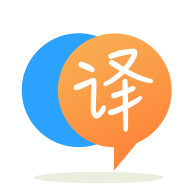
[英]Redux-Toolkit extraReducers listed after reducers causes issues (TS2322 Error)
[英]Hook Error After Implementing Redux Toolkit into Authentication
當我運行我的應用程序時,我不斷收到錯誤消息,並且屏幕是白色的。
ERROR Error: Invalid hook call. Hooks can only be called inside of the body of a function component. This could happen for one of the following reasons:
1. You might have mismatching versions of React and the renderer (such as React DOM)
2. You might be breaking the Rules of Hooks
3. You might have more than one copy of React in the same app
See https://reactjs.org/link/invalid-hook-call for tips about how to debug and fix this problem., js engine: hermes
ERROR Invariant Violation: Module AppRegistry is not a registered callable module (calling runApplication). A frequent cause of the error is that the application entry file path is incorrect.
This can also happen when the JS bundle is corrupt or there is an early initialization error when loading React Native., js engine: hermes
ERROR Invariant Violation: Module AppRegistry is not a registered callable module (calling runApplication). A frequent cause of the error is that the application entry file path is incorrect.
This can also happen when the JS bundle is corrupt or there is an early initialization error when loading React Native., js engine: hermes
完整代碼可在我的 GitHub 上找到,但讓我跳過我正在擺弄的代碼區域: https://github.com/astuertz/hashtagValues/commits/master
首先,這是我的 store.js:
import { configureStore } from '@reduxjs/toolkit';
import activeImgReducer from '../features/counter/activeImgSlice';
import userAuthReducer from '../features/counter/userAuthSlice';
export default configureStore({
reducer: {
activeImg: activeImgReducer,
user: userAuthReducer
}
})
這是我的 index.js:
/**
* @format
*/
import React from 'react';
import {AppRegistry} from 'react-native';
import App from './App';
import {name as appName} from './app.json';
import store from './src/app/store';
import { Provider } from 'react-redux';
const reduxApp = () => (
<Provider store={store}>
<App />
</Provider>
);
AppRegistry.registerComponent(appName, () => reduxApp);
還有我的 App.js(沒有樣式):
import React from 'react';
import {View, Text, StyleSheet} from 'react-native';
import Navigation from './src/components/routes/navigation';
import Amplify from 'aws-amplify';
import config from './src/aws-exports';
import { withAuthenticator } from 'aws-amplify-react-native';
Amplify.configure({
...config,
Analytics: {
disabled: true,
},
});
const App = () => {
return (
<View style={styles.root}>
<Navigation />
</View>
);
};
這是我的 navigation.js(從根堆棧向下的所有內容——根堆棧中嵌套了其他幾個堆棧):
const RootStack = createNativeStackNavigator();
const RootStackScreen = () => {
const [isLoading, setIsLoading] = useState(true);
const [user, setUser] = useState(null);
useEffect(() => {
setTimeout(() => {
setIsLoading(!isLoading);
setUser('user');
}, 2500)
}, []);
return (
<RootStack.Navigator screenOptions={{
animationEnabled: false,
headerShown: false,
presentation: 'modal',
}}>
{isLoading ? (
<RootStack.Screen name="LoadingScreen" component={LoadingScreen} />
) : user ? (
<RootStack.Screen name="AppTabs" component={AppTabsScreen} />
) : (
<RootStack.Screen name="AuthStackScreen" component={AuthStackScreen} />
)}
<RootStack.Screen name="Gallery" component={GalleryScreen} options={{
animationEnabled: true,
cardStyle: {
backgroundColor: 'black',
},
}}/>
</RootStack.Navigator>
);
};
export default () => {
return (
<NavigationContainer>
<RootStackScreen />
</NavigationContainer>
);
};
我現在已經恢復到錯誤開始發生之前的狀態。
我搞砸的唯一另一件事是登錄屏幕和配置文件屏幕(帶注銷)。 這是登錄屏幕:
import React, {useState} from 'react';
import {
View,
Text,
StyleSheet,
Image,
Dimensions,
TextInput,
Button,
TouchableWithoutFeedback,
Keyboard,
TouchableOpacity,
Alert,
} from 'react-native';
import logo from '../../graphics/Values_logo.png';
import { useNavigation } from '@react-navigation/native';
import { Auth } from 'aws-amplify';
import { useSelector, useDispatch, } from 'react-redux';
import { validateUser } from '../../features/counter/userAuthSlice';
const WIDTH = Dimensions.get("window").width;
const HEIGHT = Dimensions.get("window").height;
const SignIn = () => {
const navigation = useNavigation();
const dispatch = useDispatch();
const [email, setEmail] = useState('');
const [password, setPassword] = useState('');
const onPressSignIn = async () => {
if (email.length < 1 || password.length < 1) return Alert.alert('no input', 'please input an email and password to log in.');
try {
const u = await Auth.signIn(email, password);
dispatch(validateUser(u));
} catch(e) {
Alert.alert('Login Failed!', e.message);
return;
}
Alert.alert('Login Successful!');
return;
}
const renderLogo = (
<Image
source={logo}
style={styles.logoSize}
resizeMode='contain'
/>
);
const inputElements = (
<>
<TextInput
placeholder='email'
value={email}
onChangeText={setEmail}
style={styles.textInput}
/>
<TextInput
placeholder='password'
value={password}
onChangeText={setPassword}
style={styles.textInput}
secureTextEntry={true}
/>
<TouchableOpacity
onPress={onPressSignIn}
style={styles.button}
>
<Text style={styles.buttonText}>Sign In</Text>
</TouchableOpacity>
<Text
style={styles.hyperlink}
>Forgot Password?</Text>
<Text
style={styles.hyperlink}
onPress={() => navigation.push("SignUp")}
>Sign Up</Text>
</>
);
return (
<TouchableWithoutFeedback
onPress={() => Keyboard.dismiss()}>
<View style={styles.pageContainer}>
<View style={styles.logo}>
{renderLogo}
</View>
<View style={styles.inputContainer} >
{inputElements}
</View>
</View>
</TouchableWithoutFeedback>
);
}
和個人資料屏幕:
import React from 'react';
import {View, Text, StyleSheet, Button, Alert,} from 'react-native';
import { SafeAreaView } from 'react-native-safe-area-context';
import { Auth } from 'aws-amplify';
import { useSelector, useDispatch, } from 'react-redux';
import { signOutUser } from '../../features/counter/userAuthSlice';
const dispatch = useDispatch();
const onSignOut = async () => {
try {
await Auth.signOut();
dispatch(signOutUser());
} catch (error) {
Alert.alert('error signing out: ', error.message);
return;
}
Alert.alert('Sign Out Successful!');
}
const ProfileScreen = () => {
return (
<SafeAreaView style={styles.pageContainer}>
<Text>ProfileScreen</Text>
<Button title="Sign Out" onPress={onSignOut} />
</SafeAreaView>
);
};
const styles = StyleSheet.create({
root: {
flex: 1
},
pageContainer: {
justifyContent: 'center',
alignItems: 'center',
flex: 1,
width: '100%'
}
});
export default ProfileScreen;
我真的不確定我做了什么來破壞我的應用程序或如何修復它。
在配置文件屏幕中,您正在調用const dispatch = useDispatch();
它在組件之外並且是無效調用。 它必須在 ProfileScreen 內部調用。 當您不確定問題出在哪里時,請嘗試注釋您的代碼,看看沒有它們是否能正常工作。 就像一個一個地評論你的屏幕會幫助你找到錯誤是由哪個屏幕引起的等等。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.