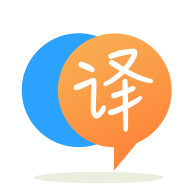
[英]Flutter Firestore: How to retrieve map data from Firestore and display in Flutter Widget
[英]How to retrieve data from firestore to display in multi select form field
您好,我是 flutter 的新人。我正在嘗試從 firestore 獲取症狀列表並將其顯示在“MultiSelectFormField”中。 我怎樣才能做到? 我應該添加什么?
MultiSelectFormField(
autovalidate: AutovalidateMode.disabled,
chipBackGroundColor: Colors.blue[900],
chipLabelStyle: TextStyle(fontWeight: FontWeight.bold, color: Colors.white),
dialogTextStyle: TextStyle(fontWeight: FontWeight.bold),
checkBoxActiveColor: Colors.blue[900],
checkBoxCheckColor: Colors.white,
dialogShapeBorder: RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(12.0))),
title: Text(
"Symptoms",
style: TextStyle(fontSize:20),
),
validator: (value) {
if (value == null || value.length == 0) {
return 'Please select one or more symptoms';
}
return null;
},
dataSource: ['value': symptomsList],
textField: 'value',
valueField: 'value',
okButtonLabel: 'OK',
cancelButtonLabel: 'CANCEL',
hintWidget: Text('Please choose one or more symptoms'),
initialValue: _symptoms,
onSaved: (value) {
if (value == null) return;
setState(() {
_symptoms = value;
});
},
),
重要的是,您已在 firebase 項目中注冊了您的應用程序並將包: Firebase Core和Cloud Firestore添加到您的pubspec.yaml文件中
要從雲 firestore 檢索數據,請確保您已導入雲 firestore package
import 'package:cloud_firestore/cloud_firestore.dart';
完成后創建一個名為getDataFromFirestore()
的 function
getDataFromFirestore(var collection, var data) async {
await FirebaseFirestore.instance
.collection(collection)
.get()
.then((value) {
// here we set the data to the data
data = value.docs;
});
}
完成后,我們可以在 auer initstate 中使用 function:
List symptoms = [];
...
@override
void initState() {
super.initState();
asyncTasks() async {
var data;
await getDataFromFirestore("symptoms", data);
// here we fill up the list symptoms
for(var item in data) {
symptoms.add(item.data()["name"]);
}
setState(() {});
}
asyncTasks();
}
要從雲 firestore 檢索數據,請確保您已導入雲 firestore package
import 'package:cloud_firestore/cloud_firestore.dart';
完成后創建一個名為getDataFromFirestore()
的 function
// the document Id is the id from the document where the symptoms list is in
getDataFromFirestore(var collection, var data, var documentId) async {
await FirebaseFirestore.instance
.collection(collection)
.doc(documentId)
.get()
.then((value) {
// here we set the data to the data
data = value.data();
});
}
完成后,我們可以在 auer initstate 中使用 function:
List symptoms = [];
...
@override
void initState() {
super.initState();
asyncTasks() async {
var data;
await getDataFromFirestore("symptoms", data);
// here we fill up the list symptoms
for(var item in data) {
symptoms.add(item["name"]);
}
setState(() {});
}
asyncTasks();
}
MultiSelectFormField(
autovalidate: AutovalidateMode.disabled,
chipBackGroundColor: Colors.blue[900],
chipLabelStyle: TextStyle(fontWeight: FontWeight.bold, color: Colors.white),
dialogTextStyle: TextStyle(fontWeight: FontWeight.bold),
checkBoxActiveColor: Colors.blue[900],
checkBoxCheckColor: Colors.white,
dialogShapeBorder: RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(12.0))),
title: Text(
"Symptoms",
style: TextStyle(fontSize:20),
),
validator: (value) {
if (value == null || value.length == 0) {
return 'Please select one or more symptoms';
}
return null;
},
// you can simply use the list
dataSource: ['value': symptoms[0]],
textField: 'value',
valueField: 'value',
okButtonLabel: 'OK',
cancelButtonLabel: 'CANCEL',
hintWidget: Text('Please choose one or more symptoms'),
initialValue: _symptoms,
onSaved: (value) {
if (value == null) return;
setState(() {
_symptoms = value;
});
},
),
希望能幫助到你!
首先,申報stream
final Stream<QuerySnapshot> symptomsStream = FirebaseFirestore.instance.collection('symptoms').snapshots();
然后使用stream構建器查詢症狀stream。聲明症狀列表並填寫列表症狀。 在datasource
中,使用for
來逐一顯示列表項。
StreamBuilder(
stream: symptomsStream,
builder: (BuildContext context, AsyncSnapshot<QuerySnapshot> snapshot){
if(snapshot.hasError){
print('Something went wrong');
}
if(snapshot.connectionState == ConnectionState.waiting){
return const Center(
child: CircularProgressIndicator(),
);
}
final List symptomsList = [];
//fill up the list symptoms
snapshot.data!.docs.map((DocumentSnapshot document){
Map a = document.data() as Map<String, dynamic>;
symptomsList.add(a['name']);
a['id'] = document.id;
}).toList();
return MultiSelectFormField(
autovalidate: AutovalidateMode.disabled,
chipBackGroundColor: Colors.blue[900],
chipLabelStyle: TextStyle(fontWeight: FontWeight.bold, color: Colors.white),
dialogTextStyle: TextStyle(fontWeight: FontWeight.bold),
checkBoxActiveColor: Colors.blue[900],
checkBoxCheckColor: Colors.white,
dialogShapeBorder: RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(12.0))),
title: Text(
"Symptoms",
style: TextStyle(fontSize:20),
),
validator: (value) {
if (value == null || value.length == 0) {
return 'Please select one or more symptoms';
}
return null;
},
dataSource: [
for (String i in symptomsList) {'value' : i}
],
textField: 'value',
valueField: 'value',
okButtonLabel: 'OK',
cancelButtonLabel: 'CANCEL',
hintWidget: Text('Please choose one or more symptoms'),
initialValue: _symptoms,
onSaved: (value) {
if (value == null) return;
setState(() {
_symptoms = value;
}
);
},
);
}
),
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.