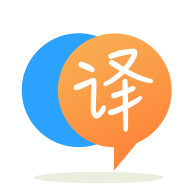
[英]How to stop restarting for loop when dependency change in useEffect (React js)
[英]How to stop a React App breaking on refreshing when using UseEffect
import {useState, useEffect } from 'react'
import axios from 'axios'
const Singlecountry = ({searchedCountries, setWeather, weather}) => {
const weatherName = searchedCountries[0].capital
const iconname = () => {
if (weather === undefined) {
return null
}
weather.map(w => w.weather[0].icon)
}
console.log(iconname)
useEffect(() => {
axios.get(`https://api.openweathermap.org/data/2.5/weather?q=${weatherName}&appid=${process.env.REACT_APP_API_KEY}`)
.then(response => {
const apiResponse = response.data;
console.log(apiResponse)
console.log(`Current temperature in ${apiResponse.name} is ${apiResponse.main.temp - 273.15}℃`);
setWeather([apiResponse])
}).catch(error => {
console.log(error);
})
}, [])
return(
<div>
capital: {searchedCountries.map(c => <p>{c.capital}</p>)}
area: {searchedCountries.map(c => <p>{c.area}</p>)}
<h2>Languages</h2>
<ul>
{
searchedCountries.map(c =>
<ul>
{Object.values(c.languages).map(l => <li>{l}</li>)}
</ul>
)
}
</ul>
{searchedCountries.map(c => <img src={Object.values(c.flags)[0]} alt="" /> )}
<h3>Weather</h3>
<p>temperature is {weather.map(w => w.main.temp - 273.15)} degrees Celsius</p>
<p>wind is {weather.map(w => w.wind.speed)} miles per hour</p>
<img src={`http://openweathermap.org/img/wn/${iconname}.png`} alt="" />
</div>
)
}
const Countries = ({ searchedCountries, handleClick, show, setWeather, setCountries, weather}) => {
if (weather === undefined) {
return null
}
if (searchedCountries.length >= 10) {
return (
<div>
<p>too many countries to list, please narrow your search</p>
</div>
)
}
if (searchedCountries.length === 1) {
return (
<Singlecountry searchedCountries={searchedCountries} setWeather={setWeather} weather={weather}/>
)
}
if (show === true) {
return (
<Singlecountry searchedCountries={searchedCountries} setWeather={setWeather} />
)
}
return (
<ul>
{searchedCountries.map(c => <li>{c.name.common}<button onClick={handleClick} >show</button></li>)}
</ul>
)
}
const App = () => {
const [countries, setCountries] = useState([])
const [newSearch, setNewSearch] = useState('')
const [show, setShow] = useState(false)
const [weather, setWeather] = useState('')
const handleSearchChange = (event) => {
setNewSearch(event.target.value)
}
const handleClick = () => {
setShow(!show)
}
const searchedCountries =
countries.filter(c => c.name.common.includes(newSearch))
useEffect(() => {
axios
.get('https://restcountries.com/v3.1/all')
.then(response => {
setCountries(response.data)
})
}, [])
return (
<div>
<div><p>find countries</p><input value={newSearch} onChange={handleSearchChange} /></div>
<div>
<h2>countries</h2>
<Countries searchedCountries={searchedCountries} handleClick={handleClick} show={show} setCountries={setCountries} setWeather={setWeather} weather={weather}/>
</div>
</div>
)
}
export default App
下面的代碼旨在當用戶在搜索欄中輸入國家名稱時顯示國家信息,包括首都、溫度和天氣。
該應用程序從國家 API 獲取國家數據,當用戶搜索特定國家時,它會從天氣 API 獲取天氣。
但是,當應用程序刷新時,應用程序會在搜索單個國家/地區的天氣時中斷。
有誰知道這是為什么以及如何解決?
謝謝
看起來您在 useEffect 中使用 axios,這可能會導致無限循環並使您的應用程序崩潰。 我建議為您的數據獲取創建一個單獨的 function,然后像這樣在useEffect
中調用 function:
const fetchCountries = useCallback(() => {
axios
.get('https://restcountries.com/v3.1/all')
.then(response => {
setCountries(response.data)
})
}, [])
useEffect(() => {
fetchCountries()
}, [fetchCountries])
關鍵是useEffect
中的依賴數組,只有當來自fetchCountries
function 的國家列表發生變化時才會更新,從而防止無限循環。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.