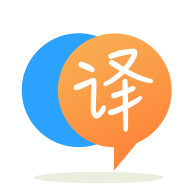
[英]JSON how find another value at the same index from a value in Javascript Object
[英]How can I find the value from another value in the same line with JavaScript (JSON)?
我正在嘗試從一個數字中獲取“正確答案”。
這是 JSON 的示例:
{
"questions": [
{
"number": 3,
"question": "☀️ ➕ 🌼 = ?",
"answers": [
"🌻sunflower",
"BIRTHDAY",
"iPhone",
"MOON CAKE"
],
"correctAnswers": [
"🌻sunflower"
],
"random": true,
"timeLimit": "24"
},
{
"number": 1,
"question": "⭐️ ➕ 🐟 =❓ ",
"answers": [
"STAR FISH",
"iPhone",
"MOON CAKE",
"HOT DOG"
],
"correctAnswers": [
"STAR FISH"
],
"random": true,
"timeLimit": "20"
},
{
"number": 7,
"question": "👁 ➕ ☎ = ",
"answers": [
"SUNGLASSES",
"SUNFLOWER",
"HOUSEPAINT",
"IPHONE"
],
"correctAnswers": [
"IPHONE"
],
"random": true,
"timeLimit": 15
},
]
}
我試圖找到“數字”:1,或“問題”中的隨機數,然后從該數字中找到“正確答案”。
const array = {
"questions": [
{
"number": 3,
"question": "☀️ ➕ 🌼 = ?",
"answers": [
"🌻sunflower",
"BIRTHDAY",
"iPhone",
"MOON CAKE"
],
"correctAnswers": [
"🌻sunflower"
],
"random": true,
"timeLimit": "24"
},
{
"number": 1,
"question": "⭐️ ➕ 🐟 =❓ ",
"answers": [
"STAR FISH",
"iPhone",
"MOON CAKE",
"HOT DOG"
],
"correctAnswers": [
"STAR FISH"
],
"random": true,
"timeLimit": "20"
},
{
"number": 7,
"question": "👁 ➕ ☎ = ",
"answers": [
"SUNGLASSES",
"SUNFLOWER",
"HOUSEPAINT",
"IPHONE"
],
"correctAnswers": [
"IPHONE"
],
"random": true,
"timeLimit": 15
},
]
};
const { questions } = array;
// Find by number
const correctAnswersByNumber = qNumber => {
const q = questions.find(({ number }) => number === qNumber);
if (!q) {
return false;
}
return q.correctAnswers;
};
console.log(correctAnswersByNumber(3)); // Question by number === 3
// Random question
const randomCorrectAnswers = questions[Math.floor(Math.random() * questions.length)].correctAnswers;
console.log(randomCorrectAnswers);
使用隨機選擇一個隨機問題。 然后顯示正確的屬性。
var data = { "questions": [ { "number": 3, "question": "☀️ ➕ 🌼 = ?", "answers": [ "🌻sunflower", "BIRTHDAY", "iPhone", "MOON CAKE" ], "correctAnswers": [ "🌻sunflower" ], "random": true, "timeLimit": "24" }, { "number": 1, "question": "⭐️ ➕ 🐟 =❓ ", "answers": [ "STAR FISH", "iPhone", "MOON CAKE", "HOT DOG" ], "correctAnswers": [ "STAR FISH" ], "random": true, "timeLimit": "20" }, { "number": 7, "question": "👁 ➕ ☎ = ", "answers": [ "SUNGLASSES", "SUNFLOWER", "HOUSEPAINT", "IPHONE" ], "correctAnswers": [ "IPHONE" ], "random": true, "timeLimit": 15 }, ] }; // Let's choose a random question let question = data['questions'][Math.floor(Math.random() * data['questions'].length)]; console.log(question.question, question.correctAnswers)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.