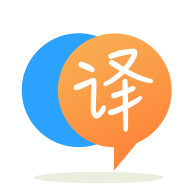
[英]Redux-Form and React-RTE - Save RTE editorValue to form state?
[英]react-rte giving TypeError: r.getEditorState is not a function in next js
我有一個 nextjs 項目,它有一個 react-rte 組件,react-rte 組件顯示正確,但是當我轉到其他組件並單擊瀏覽器后退按鈕時,我收到以下錯誤:
Unhandled Runtime Error TypeError: r.getEditorState is not a function
當我注釋掉 react-rte 組件時,錯誤不再發生
反應組件
import React, { useState, useEffect } from "react";
import dynamic from "next/dynamic";
import PropTypes from "prop-types";
//import the component
const RichTextEditor = dynamic(() => import("react-rte"), { ssr: false });
const MyStatefulEditor = ({ onChange }) => {
const [value, setValue] = useState([]);
console.log(value.toString("html"));
useEffect(() => {
const importModule = async () => {
//import module on the client-side to get `createEmptyValue` instead of a component
const module = await import("react-rte");
console.log(module);
setValue(module.createEmptyValue());
};
importModule();
}, []);
const handleOnChange = (value) => {
setValue(value);
if (onChange) {
onChange(value.toString("html"));
}
};
return <RichTextEditor value={value} onChange={handleOnChange} />;
};
MyStatefulEditor.propTypes = {
onChange: PropTypes.func,
};
export default MyStatefulEditor;
嘗試這個
import React, { useState, useEffect } from "react";
import dynamic from "next/dynamic";
import PropTypes from "prop-types";
const RichTextEditor = dynamic(() => import("react-rte"), { ssr: false });
const MyStatefulEditor = ({ onChange }) => {
const [value, setValue] = useState([]);
useEffect(() => {
// set the state value using the package available method
setValue(RichTextEditor.createEmptyValue())
}, []);
const handleOnChange = (value) => {
setValue(value);
if (onChange) {
onChange(value.toString("html"));
}
};
return <RichTextEditor value={value} onChange={handleOnChange} />;
};
MyStatefulEditor.propTypes = {
onChange: PropTypes.func,
};
export default MyStatefulEditor;
就像我在之前的評論中提到的那樣,我注意到您正在導入react-rte
包兩次。
在useEffect
鈎子中,您可以通過查看此處找到的示例代碼進行導入以初始化value
狀態
您可以使用來自已導入包的RichTextEditor.createEmptyValue()
來實現。
您會注意到我更改了導入,不是動態的,嘗試一下,如果它有效,那么如果這是您需要的,請嘗試進行動態導入。
您可以在渲染RichTextEditor
之前添加條件來檢查value
import React, { useState, useEffect } from "react";
import dynamic from "next/dynamic";
import PropTypes from "prop-types";
import { useRouter } from "next/router";
//import the component
const RichTextEditor = dynamic(() => import("react-rte"), { ssr: false });
const MyStatefulEditor = ({ onChange }) => {
const [value, setValue] = useState();
const router = useRouter();
useEffect(() => {
const importModule = async () => {
//import module on the client-side to get `createEmptyValue` instead of a component
const module = await import("react-rte");
setValue(module.createEmptyValue());
};
importModule();
}, [router.pathname]);
const handleOnChange = (value) => {
setValue(value);
if (onChange) {
onChange(value.toString("html"));
}
};
//if `value` from react-rte is not generated yet, you should not render `RichTextEditor`
if (!value) {
return null;
}
return <RichTextEditor value={value} onChange={handleOnChange} />;
};
MyStatefulEditor.propTypes = {
onChange: PropTypes.func
};
export default MyStatefulEditor;
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.