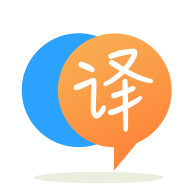
[英]Appium : how to check device name using automation test cases in java
[英]How to automation testing with a Console Application with already test cases set
我剛剛完成了一個用於學生管理的Java CONSOLE 應用程序。
我收到了基於標准程序(來自我的講師)構建的測試用例集( pdf 文件包含根據應用程序要求遵循的行) 。 您可以概述我的應用程序的功能以及在下面的附圖中設置的測試用例圖像描述格式是什么。
問題是我想使用測試用例來測試我的應用程序,而不是在控制台 IO 和 pdf 文件之間手動輸入和逐行匹配=> 我想編寫一個程序來自動導入和匹配我的 jar/ 之間的數據程序來測試用例。
但是,我不確定如何以及從哪里開始。 我已經嘗試過使用谷歌,但單元測試/白色測試仍然是我所有搜索的內容。 希望在繼續嘗試用google搜索的過程中,有人能給我一些對我有用的建議或方向。 非常感謝。
我這樣做的方法是將您的應用程序與控制台分離,以便您可以在測試中使用假實現從控制台打印和讀取。 “假”是一個技術術語——你可以查找“測試替身”來了解這些和其他相關的想法。 這個想法被稱為依賴注入,或依賴倒置原則。
我們這樣做的方式是使用接口。 下面是一個打印一些項目的應用程序示例:
import java.util.List;
public class ItemPrinterApplication {
public ItemPrinterApplication(OutputWriter outputWriter, List<Item> items) {
this.outputWriter = outputWriter;
this.items = items;
}
public void run() {
outputWriter.writeLine("Name, Price");
items.forEach(item -> outputWriter.writeLine(item.name + ", " + item.price));
}
private OutputWriter outputWriter;
private List<Item> items;
}
OutputWriter
是負責打印的東西。 它只是一個接口,所以應用程序不知道它是寫入控制台還是其他地方:
public interface OutputWriter {
void writeLine(String line);
}
為了完整起見, Item
類只包含一些數據:
public class Item {
public Item(String name, Integer price) {
this.name = name;
this.price = price;
}
public final String name;
public final Integer price;
}
然后我可以使用JUnit編寫一個測試,檢查當我運行這個應用程序時,我得到了我想要的輸出。 我通過使用只寫入字符串的OutputWriter
實現來做到這一點。 這樣就很容易在測試中檢查:
import org.junit.jupiter.api.Assertions;
import org.junit.jupiter.api.Test;
import java.util.List;
public class ItemPrinterTest {
@Test
public void itPrintsAListOfItems() {
List<Item> items =
List.of(
new Item("Apple", 50),
new Item("Carrot", 25),
new Item("Milk", 120)
);
FakeOutputWriter fakeOutputWriter = new FakeOutputWriter();
ItemPrinterApplication app = new ItemPrinterApplication(fakeOutputWriter, items);
app.run();
Assertions.assertEquals(
"Name, Price\n" +
"Apple, 50\n" +
"Carrot, 25\n" +
"Milk, 120\n",
fakeOutputWriter.written
);
}
}
和FakeOutputWriter
看起來像
public class FakeOutputWriter implements OutputWriter {
public String written = "";
@Override
public void writeLine(String line) {
written += line;
written += "\n";
}
}
這讓我有信心正確地編寫輸出。 不過,在main
中,我想實際打印到控制台:
import java.util.List;
public class Main {
public static void main(String[] args) {
OutputWriter outputWriter = new ConsoleOutputWriter();
List<Item> items =
List.of(
new Item("Apple", 50),
new Item("Carrot", 25),
new Item("Milk", 120)
);
new ItemPrinterApplication(outputWriter, items).run();
}
}
而ConsoleOutputWriter
正是這樣做的:
public class ConsoleOutputWriter implements OutputWriter{
@Override
public void writeLine(String line) {
System.out.println(line);
}
}
您可以采用相同的方法來偽造閱讀輸入。 您的接口將有一個不帶參數並讀取字符串的函數:
interface InputReader {
String readLine()
}
所以在測試中你可以偽造它,在main
中,使用Scanner
或其他東西閱讀。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.