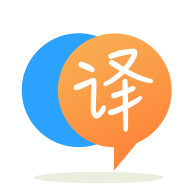
[英]What is the best way to represent all numbers in a given range? (some restrictions)
[英]What is the best way to ensure that all inputs are all unique Numbers
這是一個示例輸入:
字符串測試 = "1 2 3 4 5 42"; 字符串 test2 = test.replaceAll(" ","");
public static boolean uniqueNumbers(String test2) {
char[] testEntries= test2.toCharArray();
Set<Character> set = new HashSet<>();
for (int i = 0 ; i < testEntries.length ; i++ ) {
if(!set.add(testEntries[i])) {
return false;
}
}
return true;
}
盡管都是唯一的數字,它會返回為假。 有沒有辦法來解決這個問題?
您應該首先使用 split() 拆分輸入參數。
String test = "1 2 3 4 5 42";
String[] origin = test.split(" ");
Set<String> check = new HashSet<>(Arrays.asList(origin));
for (String el : check) {
System.out.println(el);
}
Set.of
捕獲IllegalArgumentException
Java 9+ 中的Set.of
便捷方法在傳遞數組時創建了一個未指定實現的Set
。 如果在構建此Set
時遇到任何重復項,則會引發異常。 您可以捕獲該IllegalArgumentException
以了解您的字符串的各個部分是否不同。
我們可以通過調用String#split
來獲取字符串部分的數組。
Boolean isDistinct;
try
{
Set.of( "1 3 3 4 5 42".split( " " ) );
isDistinct = Boolean.TRUE;
}
catch ( IllegalArgumentException e )
{
isDistinct = Boolean.FALSE;
}
System.out.println( "Input is distinct: " + Objects.requireNonNull( isDistinct ) );
輸入不同:假
當您將 String 參數傳遞給 uniqueNumbers() 時,它看起來像
1234542
將其拆分為 char 數組將每個單個字符作為一個單獨的元素,立即打印輸出,例如:
char[] testEntries = test2.toCharArray();
System.out.println(Arrays.toString(testEntries));
//output: [1, 2, 3, 4, 5, 4, 2]
因此,當將每個元素傳遞給集合時,它會發現 2 在索引 1 和 6 處重復。
在這種情況下,一種解決方案是使用 String 的實例 split() 通過原始拆分測試。
String test = "1 2 3 4 5 42";
String[] test2=test.split(" ");
public static boolean uniqueNumbers(String testEntries[]) {
Set<String> set = new HashSet<>();
for (int i = 0; i < testEntries.length; i++) {
if (!set.add(testEntries[i])) {
return false;
}
}
return true;
}
//output: true
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.