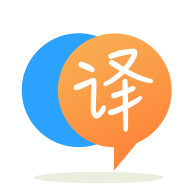
[英]Error: 'django.db.utils.IntegrityError: NOT NULL constraint failed: base_user.is_superuser'
[英]django.db.utils.IntegrityError: NOT NULL constraint failed: bankapp_userregistermodel.date_of_birth i m getting this error when i create the superuser
請幫我解決這個問題\
模型.py
from django.db import models
from django.utils import timezone
from django.contrib.auth.models import BaseUserManager, AbstractBaseUser
from django.contrib.auth.models import PermissionsMixin
class MyuserManager(BaseUserManager):
def create_user(self, mobile_number, password=None):
if not mobile_number:
raise ValueError("user must have an mobile number")
user = self.model(mobile_number = mobile_number)
user.set_password(password)
user.save(using=self._db)
return user
def create_superuser(self, mobile_number, password=None):
user = self.create_user(mobile_number, password=password)
user.is_admin = True
user.save(using=self._db)
return user
class UserRegisterModel(AbstractBaseUser, PermissionsMixin):
mobile_number = models.IntegerField(verbose_name="Mobile Number", unique=True)
bank_ac = models.CharField(max_length=64)
first_name = models.CharField(max_length=64)
last_name = models.CharField(max_length=64)
user_name = models.CharField(max_length=64)
email = models.EmailField()
date_of_birth = models.DateField(null=False)
address = models.CharField(max_length=160)
pin_code = models.IntegerField()
is_active = models.BooleanField(default=True)
is_staff = models.BooleanField(default=True)
is_admin = models.BooleanField(default=True)
date_joined = models.DateTimeField(default=timezone.now)
objects = MyuserManager()
USERNAME_FIELD = "mobile_number"
REQUIRED_FIELDS = []
def __str__(self):
return self.mobile_number
def has_perm(self, perm, obj=None):
return True
def has_module_perm(self, app_label):
return True
@property
def is_superuser(self):
return self.is_admin
admin.py
from django.contrib import admin
from bankapp.models import UserRegisterModel
from django.contrib.auth.admin import UserAdmin as BaseUserAdmin
class UserAdmin(BaseUserAdmin):
list_display = ['bank_ac','first_name','last_name','user_name','mobile_number','email','password','date_of_birth','address','pin_code']
list_filter = ['bank_ac']
search_fields = ['mobile_number']
ordering = ['mobile_number']
filter_horizontal = []
fieldsets = []
add_fieldssets = [
[None, {
'classes' : ['wide',],
'fields' : ['mobile_number','email','password'],
}],
]
admin.site.register(UserRegisterModel, UserAdmin)
forms.py
from django import forms
from .models import UserRegisterModel
import datetime
from django.core.exceptions import ValidationError
class LoginForm(forms.Form):
mobile_number = forms.CharField(label='Mobile Number',
widget=forms.TextInput(
attrs={'placeholder':'Enter Your Mobile Number',
'class':'form-control'}
)
)
password = forms.CharField(label='Password',
widget=forms.PasswordInput(
attrs={'placeholder':"Enter Your Password",
'class':'form-control'}
)
)
class RegisterForm(forms.ModelForm):
repassword = forms.CharField(label='Re Enter Password',
widget=forms.PasswordInput(attrs={'placeholder':'Re Enter Password', 'class':'form-control'})
# forms.DateField(widget=NumberInput(attrs={'type': 'date'}))
)
class Meta:
model = UserRegisterModel
fields = ['bank_ac','first_name','last_name','user_name','mobile_number','email','date_of_birth','address','pin_code','password','date_joined']
widgets = {
'bank_ac': forms.NumberInput(attrs={'placeholder': 'First Name', 'class':'form-control'}),
'first_name': forms.TextInput(attrs={'placeholder': 'First Name', 'class':'form-control'}),
'last_name': forms.TextInput(attrs={'placeholder': 'Last Name', 'class':'form-control'}),
'user_name': forms.TextInput(attrs={'placeholder': 'User Name', 'class':'form-control'}),
'mobile_number': forms.NumberInput(attrs={'placeholder': 'Name', 'class':'form-control'}),
'email': forms.EmailInput(attrs={'placeholder': 'Email', 'class':'form-control'}),
'date_of_birth': forms.NumberInput(attrs={'type': 'date'}),
'address': forms.TextInput(attrs={'placeholder': 'Addeess', 'class':'form-control'}),
'pin_code': forms.NumberInput(attrs={'placeholder': 'Pincode', 'class':'form-control'}),
'password': forms.PasswordInput(attrs={'placeholder': 'Password','class':'form-control'}),
}
def clean(self):
cleaned_data = super().clean()
pwd = self.cleaned_data["password"]
rpwd = self.cleaned_data["repassword"]
if pwd != rpwd:
raise forms.ValidationError('Both Passwords must be same')
views.py
from django.shortcuts import render, redirect
from bankapp.forms import RegisterForm, LoginForm
from bankapp.models import UserRegisterModel
from django.contrib.auth import login, authenticate, logout
from django.contrib import messages
def Home(request):
return render(request, 'home.html')
def RegistrationView(request):
if request.method == 'POST':
form = RegisterForm(data=request.POST)
if form.is_valid():
user = form.save()
login(request, user)
messages.success(request, 'Registration Successful')
return redirect('/')
else:
messages.error(request, 'Unsuccessful Registration. Invalid Informtion')
form = RegisterForm()
return render(request, template_name='bankapp/Registration.html', context={'register_form':form})
def LoginView(request):
if request.method == 'POST':
form = LoginForm(request.POST)
if form.is_valid():
username = form.cleaned_data.get('mobile_number')
password = form.cleaned_data.get('password')
user = authenticate(username=username, password=password)
if user is not None:
login(request, user)
messages.info(request, f'You are now logged in as {username}.')
return redirect('/')
else:
messages.error(request, 'invalid username or password.')
else:
messages.error(request, 'invalid username or password.')
form = LoginForm()
return render(request=request, template_name='accounts/login.html', context={'login_form':form})
def logoutView(request):
logout(request)
messages.info(request, "You have succesfully Logged out.")
return redirect("/")
# HttpResponse ("Logout Successfully")
_execute 中的文件“C:\Users\sms14\AppData\Local\Programs\Python\Python310\lib\site-packages\django\db\backends\utils.py”,第 85 行 return self.cursor.execute(sql, params) 文件“C:\Users\sms14\AppData\Local\Programs\Python\Python310\lib\site-packages\django\db\backends\sqlite3\base.py”,第 416 行,執行返回 Database.Cursor。執行(自我,查詢,參數) sqlite3.IntegrityError:NOT NULL 約束失敗:bankapp_userregistermodel.date_of_birth
上述異常是以下異常的直接原因:
回溯(最后一次調用):文件“C:\Users\sms14\Projects\MyProjects\omkarbank\manage.py”,第 22 行,在 main() 文件“C:\Users\sms14\Projects\MyProjects\omkarbank\ manage.py”,第 18 行,在主 execute_from_command_line(sys.argv) 文件“C:\Users\sms14\AppData\Local\Programs\Python\Python310\lib\site-packages\django\core\management_init _.py ",第 425 行,在 execute_from_command_line 實用程序.execute() 文件中 "C:\Users\sms14\AppData\Local\Programs\Python\Python310\lib\site-packages\django\core\management_init _.py ",第 419 行, 在執行 self.fetch_command(subcommand).run_from_argv(self.argv) 文件 "C:\Users\sms14\AppData\Local\Programs\Python\Python310\lib\site-packages\django\core\management\base.py ",第 373 行,run_from_argv self.execute(*args, **cmd_options) 文件“C:\Users\sms14\AppData\Local\Programs\Python\Python310\lib\site-packages\django\contrib\auth\management \commands\createsuperuser.py”,第 79 行,在執行中返回 super().execute(*args, **options) 文件“C:\Users\sms14\AppData \Local\Programs\Python\Python310\lib\site-packages\django\core\management\base.py",第 417 行,在執行輸出 = self.handle(*args, **options) 文件“C:\Users \sms14\AppData\Local\Programs\Python\Python310\lib\site-packages\django\contrib\auth\management\commands\createsuperuser.py”,第 195 行,在句柄 self.UserModel._default_manager.db_manager(數據庫)中。 create_superuser(**user_data) 文件“C:\Users\sms14\Projects\MyProjects\omkarbank\bankapp\models.py”,第 21 行,在 create_superuser
user = self.create_user(mobile_number, password=password) 文件“C:\Users\sms14\Projects\MyProjects\omkarbank\bankapp\models.py”,第 17 行,在 create_user user.save(using=self._db) 文件中“C:\Users\sms14\AppData\Local\Programs\Python\Python310\lib\site-packages\django\contrib\auth\base_user.py”,第 66 行,保存 super().save(*args, * *kwargs) 文件“C:\Users\sms14\AppData\Local\Programs\Python\Python310\lib\site-packages\django\db\models\base.py”,第 743 行,保存 self.save_base(using=使用,force_insert=force_insert,文件“C:\Users\sms14\AppData\Local\Programs\Python\Python310\lib\site-packages\django\db\models\base.py”,第 780 行,在 save_base 中更新 = self ._save_table(文件“C:\Users\sms14\AppData\Local\Programs\Python\Python310\lib\site-packages\django\db\models\base.py”,第 885 行,在 _save_table 結果 = self._do_insert( cls._base_manager, using, fields, returned_fields, raw) 文件“C:\Users\sms14\AppData\Local\Programs\Python\Python310\lib\site-packages\django\db\models\base.py”,行 923,在 _do_insert 返回 manager._insert( 文件 "C:\Users\sms14\AppData\Local\Programs\Python\Python310\lib\site-packages\django\db\models\manager.py",第 85 行,在 manager_method返回 getattr(self.get_queryset(), name)(*args, **kwargs) 文件 "C:\Users\sms14\AppData\Local\Programs\Python\Python310\lib\site-packages\django\db\models\ query.py”,第 1301 行,在 _insert 返回 query.get_compiler(using=using).execute_sql(returning_fields) 文件“C:\Users\sms14\AppData\Local\Programs\Python\Python310\lib\site-packages\django \db\models\sql\compiler.py”,第 1441 行,在 execute_sql cursor.execute(sql, params) 文件“C:\Users\sms14\AppData\Local\Programs\Python\Python310\lib\site-packages\ django\db\backends\utils.py”,第 99 行,在執行中返回 super().execute(sql, params) 文件“C:\Users\sms14\AppData\Local\Programs\Python\Python310\lib\site- packages\django\db\backends\utils.py”,第 67 行,在執行中返回 self._execute_with_wrappers(sql, params, many=False, executor=self._execute) 文件“C:\Users\sm s14\AppData\Local\Programs\Python\Python310\lib\site-packages\django\db\backends\utils.py”,第 76 行,在 _execute_with_wrappers 返回執行程序(sql,params,many,context)文件“C:\ Users\sms14\AppData\Local\Programs\Python\Python310\lib\site-packages\django\db\backends\utils.py”,第 80 行,在 _execute 中使用 self.db.wrap_database_errors:文件“C:\Users\ sms14\AppData\Local\Programs\Python\Python310\lib\site-packages\django\db\utils.py”,第 90 行,退出從 exc_value 文件“C:\Users\sms14\ AppData\Local\Programs\Python\Python310\lib\site-packages\django\db\backends\utils.py”,第 85 行,在 _execute 中返回 self.cursor.execute(sql, params) 文件“C:\Users\ sms14\AppData\Local\Programs\Python\Python310\lib\site-packages\django\db\backends\sqlite3\base.py",第 416 行,在執行中返回 Database.Cursor.execute(self, query, params) django .db.utils.IntegrityError:NOT NULL 約束失敗:bankapp_userregistermodel.date_of_birth
謝謝你的回答我已經通過你的回答改變了一些代碼它正在工作非常感謝你兄弟
模型.py
date_of_birth = models.DateField(null=True)
pin_code = models.IntegerField(null=True)
表格.py
def save(self, commit=True):
user = super().save(commit=False)
user.set_password(self.cleaned_data['password1'])
if commit:
user.save()
return user
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.