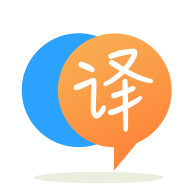
[英]Why is my code only counting the frequency of lower case letters from my string
[英]Why is my program counting letters and digits not running?
我編寫了這個函數來檢查字符串中的大寫、小寫和數字,但是當我嘗試運行代碼時,它會彈出並且似乎無法理解問題。
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <ctype.h>
#define size 50
void statistics(char str[], int *lower, int *upper, int *digits) {
for (int i = 0; i < size; i++) {
if (islower(str[i]) != 0) {
*lower = *lower + 1;
} else
if (isupper(str[i]) != 0) {
*upper = *upper + 1;
} else
if (isalpha(str[i])) {
*digits = *digits + 1;
}
}
}
int main() {
char str[size] = { " " };
int upper = 0, lower = 0, digits = 0;
printf("Enter a string:\n");
gets_s(str);
statistics(&str[size], &lower, &upper, &digits);
printf("Lower: %d\nUpper: %d\nDigits %d", lower, upper, digits);
return 0;
}
您的代碼中有多個問題:
gets_s()
函數不可移植:它是可選的,在許多系統上不受支持。 您忘記傳遞數組大小,從而導致未定義的行為。 編譯器應該輸出一個你不應該忽略的診斷。 您應該改用fgets()
。
您不應將char
值傳遞給isupper()
和類似函數,因為它們僅針對unsigned char
類型的值和特殊的負值EOF
定義。 使用unsigned char
變量或將str[i]
參數轉換為(unsigned char)str[i]
。
您傳遞 char 數組末尾的地址而不是開頭。 只需將str
作為參數傳遞給statistics
。 statistics
函數讀取數組末尾以外的字符,調用未定義的行為,其中一個字節恰好是小於-1
的負char
值,從而觸發 Visual C++ 編譯器運行時中的診斷。 錯誤消息很難解釋,IDE 應該將您指向調用代碼。
您迭代整個數組,超出空終止符。 數組的內容在gets_s()
或fgets()
設置的空終止符之外未定義。 只需停在空終止符處。
你測試if (isalpha(ch))
你可能打算使用if (isdigit(ch))
isxxx
函數為真返回非零值,為假返回零。 在 C 中,只寫if (isdigit(c))
而不是if (isdigit(c) != 0)
似乎是多余的,這是慣用的。
將size
定義為宏很容易出錯。 使用大寫和更明確的名稱。
這是修改后的版本:
#include <ctype.h>
#include <stdio.h>
#define LINE_SIZE 50
void statistics(const char *str, int *lower, int *upper, int *digits) {
while (*str != '\0') {
unsigned char ch = *str++;
if (islower(ch)) {
*lower += 1;
} else
if (isupper(ch)) {
*upper += 1;
} else
if (isdigit(ch) {
*digits += 1;
}
}
}
int main() {
char str[LINE_SIZE];
int upper = 0, lower = 0, digits = 0;
printf("Enter a string:\n");
if (fgets(str, sizeof str, stdin)) {
statistics(str, &lower, &upper, &digits);
printf("Lower: %d\nUpper: %d\nDigits %d", lower, upper, digits);
}
return 0;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.