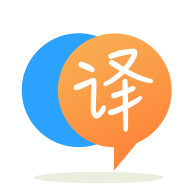
[英]ConcurrentModificationException while iterating through HashMap
[英]Iterating through a HashMap while removing AND updating the collection
我有一個計數圖,我在其中跟蹤字符串中的字符數。 我想遍歷該地圖,減少當前訪問的字符數,如果達到零則將其刪除。
在 Java 中如何做到這一點?
HashMap<Character, Integer> characterCount = new HashMap<>();
characterCount.put('a', 2);
characterCount.put('b', 1);
characterCount.put('c', 1);
Iterator<Map.Entry<Character, Integer>> iterator = characterCount.entrySet().iterator();
while (iterator.hasNext()) {
Map.Entry<Character, Integer> entry = iterator.next();
// Decrement the chosen character from the map
if (entry.getValue() == 1) {
iterator.remove();
} else {
characterCount.put(entry.getKey(), entry.getValue() - 1);
}
// Call some logic the relies on the map with the remaining character count.
// I want the characterCount.size() to return zero when there is no character with count > 0
doSomeLogic(characterCount);
// Restore the character to the map
characterCount.put(entry.getKey(), entry.getValue());
}
上面的代碼導致ConcurrentModificationException
。
由於Map#entrySet
返回映射中映射的視圖,因此直接設置Entry
的值以對其進行更新。
if (entry.getValue() == 1) {
iterator.remove();
} else {
entry.setValue(entry.getValue() - 1);
}
這是一種方法。 Map.computeIfPresent
將在條目變為null
時刪除它。 使用三元運算符要么遞減值,要么在當前為1
(即將遞減)時用null
替換。
Map<Character, Integer> map = new HashMap<>();
map.put('a', 2);
map.put('b', 1);
map.put('c', 1);
map.computeIfPresent('c', (k,v)-> v == 1 ? null : v-1);
map.computeIfPresent('a', (k,v)-> v == 1 ? null : v-1);
System.out.println(map);
印刷
{a=1, b=1}
所以,這就是它如何為你工作,替換你的迭代器和 while 循環。
for (char ch : characterCount.keySet()) {
characterCount.computeIfPresent(ch, (k,v)-> v == 1 ? null : v-1);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.