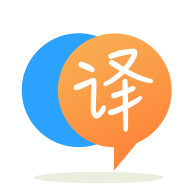
[英]Shorter way to mapDispatchToProps using React, Redux and TypeScript?
[英]Is it possible to write this a shorter way in React using onClick?
現在,我的代碼正在按預期工作,但我想知道是否有更短的方法來編寫它。 我在 React 中使用了 speaktts,它由我希望通過文本到語音的文本說出的內容組成。 我有一個函數來處理點擊,然后用戶必須點擊按鈕才能聽到這個詞。 如果用戶點擊聲音表情符號,他們將聽到蘋果、香蕉和胡蘿卜的聲音。 有什么辦法可以縮短這個時間嗎?
import React from "react";
import Speech from "speak-tts";
function Alphabet() {
const [say1] = React.useState("apple");
const [say2] = React.useState("banana");
const [say3] = React.useState("carrot");
const speech = new Speech();
speech
.init({
lang: "en-US",
rate: 0.4,
})
.then((data) => {
console.log("Speech is ready, voices are available", data);
})
.catch((e) => {
console.error("An error occured while initializing : ", e);
});
const handleClick1 = () => {
speech.speak({
text: say1,
});
};
const handleClick2 = () => {
speech.speak({
text: say2,
});
};
const handleClick3 = () => {
speech.speak({
text: say3,
});
};
return (
<>
<h2>Apple</h2>
<button
value="Apple"
onClick={handleClick1}
alt="click here for pronounciation"
className="buttonSound"
>
🔊
</button>
<h2>Banana</h2>
<button
value="Banana"
onClick={handleClick2}
alt="click here for pronounciation"
className="buttonSound"
>
🔊
</button>
<h2>Carrot</h2>
<button
value="Carrot"
onClick={handleClick3}
alt="click here for pronounciation"
className="buttonSound"
>
🔊
</button>
</>
);
}
export default Alphabet;
將<h2>
和<button>
變成它們自己的組件。 看起來唯一改變的值是標題文本,這也是按鈕的value
,也是單擊時傳遞給.speak
的文本。 文本不需要狀態,因為文本不會改變。
因此,將語音對象和文本作為道具傳遞下去。
您也不應該在每次組件呈現時都調用new Speech
。 而是只創建一次,使用 ref 或 state。
const Word = ({ text, speech }) => (
<>
<h2>{text}</h2>
<button
value={text}
onClick={() => speech.speak({ text })}
alt="click here for pronounciation"
className="buttonSound"
>
🔊
</button>
</>
);
function Alphabet() {
const [speech] = useState(() => new Speech());
useEffect(() => {
speech
.init({
lang: "en-US",
rate: 0.4,
})
.then((data) => {
console.log("Speech is ready, voices are available", data);
})
.catch((e) => {
console.error("An error occured while initializing : ", e);
});
}, []);
return (
<>
<Word text="Apple" speech={speech} />
<Word text="Banana" speech={speech} />
<Word text="Carrot" speech={speech} />
</>
);
}
我希望這段代碼對你有所幫助,你將從這段代碼中學到一些東西。 謝謝
import React from "react";
import Speech from "speak-tts";
function Alphabet() {
const data = [
{
name: "Apple"
},
{
name: "Banana"
},
{
name: "Carrot"
}
]
const speech = new Speech();
speech
.init({
lang: "en-US",
rate: 0.4,
})
.then((data) => {
console.log("Speech is ready, voices are available", data);
})
.catch((e) => {
console.error("An error occured while initializing : ", e);
});
const handlePlay = (text) => {
speech.speak({
text: text,
});
}
return (
<>
<h2>Apple</h2>
{data.map(d => (
<button
value={d.name}
onClick={() => handlePlay(d.name.toLowerCase())}
alt="click here for pronounciation"
className="buttonSound"
>
🔊
</button>
))}
</>
);
}
export default Alphabet;
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.