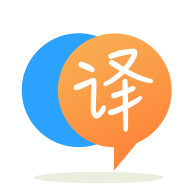
[英]BMI calculator :ValueError: could not convert string to float: X
[英]Cannot Use Float in BMI Calculator
我構建了以下代碼:
# function to calculate bmi and return a result based on user input
def calculatebmi(weight, height):
bmivalue = weight // (height ** 2)
if bmivalue < 18.5:
print("Underweight "), print(bmivalue)
elif bmivalue >= 18.5 and bmivalue <= 24.9:
print("Healthy "), print(bmivalue)
elif bmivalue <= 25.0 and bmivalue >= 29.9:
print("Overweight "), print(bmivalue)
elif bmivalue >= 30.0:
print("Obese "), print(bmivalue)
# establish usable variables based on user input
user_weight_kg1, user_height_m1 = input("What is your weight in kilograms? "), input("What is your height in meters? ")
# convert user input to float
user_weight_kg2, user_height_m2 = float(user_weight_kg1), float(user_height_m1)
# run the function
calculatebmi(user_weight_kg2, user_height_m2)
無論出於何種原因,當我輸入“75”作為重量值和“1.7”作為高度值時,它只會返回:
What is your weight in kilograms? 75
What is your height in meters? 1.7
Process finished with exit code 0
如果我使用整數,它工作正常:
What is your weight in kilograms? 80
What is your height in meters? 2
Healthy
20.0
Process finished with exit code 0
我需要能夠讓我的用戶輸入一個字符串,然后將其轉換為浮點數。 我在這里做錯了什么? 感謝您的幫助!
盡量避免在bmivalue
計算時進行整數除法
bmivalue = weight / (height ** 2)
代替
bmivalue = weight // (height ** 2)
並確保在以下位置更正聲明
elif bmivalue <= 25.0 and bmivalue >= 29.9:
改成
elif (bmivalue >= 25.0) and (bmivalue <= 29.9):
好吧,您的第二個 elif 存在問題,您應該將其更改為 >=25 和 <=29.9:
# function to calculate bmi and return a result based on user input
def calculatebmi(weight, height):
bmivalue = weight // (height ** 2)
if bmivalue < 18.5:
print("Underweight "), print(bmivalue)
elif (bmivalue >= 18.5) and (bmivalue <= 24.9):
print("Healthy "), print(bmivalue)
elif (bmivalue <= 29.9) and (bmivalue >= 25.0):
print("Overweight "), print(bmivalue)
elif bmivalue >= 30.0:
print("Obese "), print(bmivalue)
# establish usable variables based on user input
user_weight_kg1, user_height_m1 = input(
"What is your weight in kilograms? "), input("What is your height in meters? ")
# convert user input to float
user_weight_kg2, user_height_m2 = float(user_weight_kg1), float(user_height_m1)
# run the function
calculatebmi(user_weight_kg2, user_height_m2)
您的代碼包含不必要的重復比較。 它也不包含任何類型的驗證。 試試這個(輸出修改):
def get_bmi(weight, height):
try:
if (BMI := float(weight) / (float(height) ** 2)) < 18.5:
c = 'Underweight'
elif BMI < 25.0:
c = 'Healthy'
elif BMI < 30.0:
c = 'Overweight'
else:
c = 'Obese'
return f'{BMI=:.1f} -> {c}'
except ValueError as e:
return str(e)
weight = input('Input weight in kg: ')
height = input('Input your height in metres: ')
print(get_bmi(weight, height))
例子:
Input weight in kg: 72
Input height in metres: 1.8
BMI=22.2 -> Healthy
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.