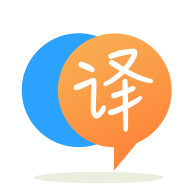
[英]How to dynamically add items and attributes to HTML with javascript or jQuery referencing items from a csv or json file
[英]How to load into HTML table just first 5 items from json file with JavaScript and JQuery?
我想首先使用 JavaScript/JQuery 顯示來自 json 文件的 5 個項目。
我有以下名為 draws.json 的 .json 文件,如下所示:
{"history": [["2022-07-04", "22:50", [5, 8, 10, 15, 17, 19, 21, 24, 28, 31, 36, 40, 47, 57, 58, 60, 61, 62, 78, 79], 756, 37.8], ["2022-07-04", "15:00", [5, 7, 9, 11, 15, 16, 17, 22, 25, 26, 31, 34, 40, 42, 45, 46, 59, 60, 65, 78], 653, 32.65], ["2022-07-03", "22:50", [1, 2, 10, 15, 16, 22, 24, 27, 29, 37, 38, 48, 51, 53, 66, 69, 71, 73, 74, 77], 803, 40.15], ["2022-07-03", "15:00", [4, 8, 11, 13, 18, 19, 24, 36, 42, 46, 48, 51, 57, 60, 63, 67, 68, 72, 73, 76], 856, 42.8], ["2022-07-02", "22:50", [2, 3, 5, 6, 8, 9, 10, 13, 24, 34, 37, 39, 44, 45, 48, 54, 55, 56, 64, 67], 623, 31.15], ["2022-07-02", "15:00", [4, 5, 9, 11, 12, 13, 19, 20, 31, 35, 39, 43, 45, 49, 52, 56, 59, 60, 72, 75], 709, 35.45]}
這只是其中的一小部分。 我只想顯示其中的前 5 個項目。
例如,一個項目是:
["2022-07-04", "22:50", [5, 8, 10, 15, 17, 19, 21, 24, 28, 31, 36, 40, 47, 57, 58, 60, 61, 62, 78, 79], 756, 37.8]
我想將日期、小時和沒有最后兩項的數字的數組加載到我的 HTML 表中,在本例中為 756 和 37.8。
為此,我創建了以下代碼,由於某種原因,即使我選擇得很好並且讀取了 json 文件,它也不會將任何行插入到表體中。
索引.html:
<!DOCTYPE html>
<html>
<head>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<style src="style/main.css"></style>
<script src="scripts/show_json.js"></script>
</head>
<body>
<div id="error_message" style="display:none">
<br>
<br>
<p align="center">The following errors occurred:</p>
<br>
<p id="xhr" align="center"></p>
<p id="msg" align="center"></p>
</div>
<div class="container">
<div class="table-responsive">
<h1 align="center">CSV File to HTML Table Using AJAX jQuery</h1>
<br />
<br />
<table id="draws_table">
<thead>
<tr>
<th>Date</th>
<th>Hour</th>
<th>N1</th>
<th>N2</th>
<th>N3</th>
<th>N4</th>
<th>N5</th>
<th>N6</th>
<th>N7</th>
<th>N8</th>
<th>N9</th>
<th>N10</th>
<th>N11</th>
<th>N12</th>
<th>N13</th>
<th>N14</th>
<th>N15</th>
<th>N16</th>
<th>N17</th>
<th>N18</th>
<th>N19</th>
<th>N20</th>
</tr>
</thead>
<tbody></tbody>
</table>
</div>
</div>
</body>
</html>
show_json.js:
function ajaxGetJson() {
var hr = new XMLHttpRequest();
hr.open("GET", "draws.json", true);
hr.setRequestHeader("Content-type", "application/json", true);
hr.onreadystatechange = function () {
if (hr.readyState == 4 && hr.status == 200) {
var data = JSON.parse(hr.responseText);
formatToTable(data);
} else {
document.getElementById("error_message").style.display = "block"
$("p#xhr").text(xhr.status);
$("p#msg").text(thrownError);
}
}
hr.send(null);
}
function formatToTable(data) {
var table = document.querySelector("#draws_table tbody");
for (var x = 0; x < len(data); x++) {
table.innerHTML = '<tr>' +
'<td>' + table.history[row_num][0].toString() + '</td>' +
'<td>' + table.history[row_num][1].toString() + '</td>' +
'<td>' + table.history[row_num][2][0].toString() + '</td>' +
'<td>' + table.history[row_num][2][1].toString() + '</td>' +
'<td>' + table.history[row_num][2][2].toString() + '</td>' +
'<td>' + table.history[row_num][2][3].toString() + '</td>' +
'<td>' + table.history[row_num][2][4].toString() + '</td>' +
'<td>' + table.history[row_num][2][5].toString() + '</td>' +
'<td>' + table.history[row_num][2][6].toString() + '</td>' +
'<td>' + table.history[row_num][2][7].toString() + '</td>' +
'<td>' + table.history[row_num][2][8].toString() + '</td>' +
'<td>' + table.history[row_num][2][9].toString() + '</td>' +
'<td>' + table.history[row_num][2][10].toString() + '</td>' +
'<td>' + table.history[row_num][2][11].toString() + '</td>' +
'<td>' + table.history[row_num][2][12].toString() + '</td>' +
'<td>' + table.history[row_num][2][13].toString() + '</td>' +
'<td>' + table.history[row_num][2][14].toString() + '</td>' +
'<td>' + table.history[row_num][2][15].toString() + '</td>' +
'<td>' + table.history[row_num][2][16].toString() + '</td>' +
'<td>' + table.history[row_num][2][17].toString() + '</td>' +
'<td>' + table.history[row_num][2][18].toString() + '</td>' +
'<td>' + table.history[row_num][2][19].toString() + '</td>' +
+ '</tr>' + table.innerHTML;
};
}
我還轉換了 .toString() 任何數字,認為這是問題所在,但它似乎根本不起作用,因為它不會將任何行插入表體。
請問有什么幫助嗎?
先感謝您!
PS 我發現這個主題正在尋找相同的東西(不知何故),但 json 文件不同並且沒有那么嵌套。 我從那里拿了一些代碼,但它似乎也不起作用,而且我只需要前 5 項而不是所有 json 文件。 只是這樣說是為了避免可能出現的重復警告,因為我已經閱讀了關於這個主題的所有可能的主題,即使它們在某種程度上相似,它們也有不同的 json 文件,並且他們要求一些其他的東西。
也許這就是你需要的?
show_json.js:
function ajaxGetJson() {
var hr = new XMLHttpRequest();
hr.open('GET', 'draws.json', true);
hr.setRequestHeader('Content-type', 'application/json', true);
hr.onload = function () {//==> CHANGED TO ONLOAD
if (hr.readyState == 4 && hr.status == 200) {
var data = JSON.parse(hr.responseText);
formatToTable(data.history); // PASSING THE HISTORY
} else {
document.getElementById('error_message').style.display = 'block';
$('p#xhr').text(xhr.status);
$('p#msg').text(xhr.thrownError);
}
};
hr.send(null);
}
function formatToTable(data) {
var table = document.querySelector('#draws_table tbody');
for (var x = 0; x < 5; x++) { //5 FIRST RECORDS OF THE JSON
let date = data[x][0];
let hour = data[x][1];
let htmlTR = '<tr>';
htmlTR += `<td>${date}</td>`;
htmlTR += `<td>${hour}</td>`;
//ADD ALL 20 NUMBERS
for (let i = 0; i < 20; i++) {
let NN = data[x][2][i];
htmlTR += `<td>${NN}</td>`;
}
htmlTR += '</tr>';
table.innerHTML += htmlTR;
}
}
索引.html:
<!DOCTYPE html>
<html>
<head>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<style src="style/main.css"></style>
<script src="scripts/show_json.js"></script>
</head>
<body>
<div id="error_message" style="display: none">
<br />
<br />
<p align="center">The following errors occurred:</p>
<br />
<p id="xhr" align="center"></p>
<p id="msg" align="center"></p>
</div>
<div class="container">
<div class="table-responsive">
<h1 align="center">CSV File to HTML Table Using AJAX jQuery</h1>
<br />
<br />
<table id="draws_table">
<thead>
<tr>
<th>Date</th>
<th>Hour</th>
<th>N1</th>
<th>N2</th>
<th>N3</th>
<th>N4</th>
<th>N5</th>
<th>N6</th>
<th>N7</th>
<th>N8</th>
<th>N9</th>
<th>N10</th>
<th>N11</th>
<th>N12</th>
<th>N13</th>
<th>N14</th>
<th>N15</th>
<th>N16</th>
<th>N17</th>
<th>N18</th>
<th>N19</th>
<th>N20</th>
</tr>
</thead>
<tbody></tbody>
</table>
</div>
</div>
<script type="text/javascript">
ajaxGetJson(); <!-- HAD TO CALL IT SOMEWHERE.. -->
</script>
</body>
</html>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.