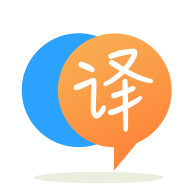
[英]Python Selenium - How to fix WebDriverException: Message: target frame detached
[英]Code throws random target frame detached in selenium/python
正如標題中所說,當嘗試在遍歷過濾器時抓取站點https://www.fragrantica.com/search/時,它會拋出 WebDriverException:消息:目標框架有時分離,有時不分離。扔掉它,它發生在隨機線而不是特定位置,所以我不知道如何解決它.. 有人建議我使用這條線:
WebDriverWait(driver, 20).until(EC.frame_to_be_available_and_switch_to_it((By.CSS_SELECTOR,"iframeCssSelector")))
但是如果它只是在整個代碼中隨機發生,它應該放在哪里。 這是我的代碼,它是提取過濾頁面具有的香水:
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.chrome.options import Options
from selenium.webdriver.common.by import By
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.support.ui import WebDriverWait
from selenium.common.exceptions import NoSuchElementException
from selenium.common.exceptions import ElementClickInterceptedException
import time
options = Options()
options.add_argument("--profile-directory=Default")
options.add_argument('--disable-blink-features=AutomationControlled')
options.add_argument("start-maximized")
options.add_argument("user-agent=Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/102.0.0.0 Safari/537.36")
driver = webdriver.Chrome(service = Service(executable_path='C:/Users/armon/Downloads/chromedriver_win32/chromedriver.exe'), options=options)
url = 'https://www.fragrantica.com/'
driver.get(url)
time.sleep(3)
perfumes_btn = driver.find_element(by=By.XPATH, value = '//*[@id="offCanvasLeft"]/ul/li[5]/a')
perfumes_btn.click()
search_btn = WebDriverWait(driver,20).until(EC.element_to_be_clickable((By.XPATH,'/html/body/div[2]/div[2]/div[2]/ul/li[5]/ul/li[1]/a')))
search_btn.click()
time.sleep(3)
Industry_more = '//*[@id="offCanvasLeftOverlap1"]/div/div/div[13]/div[2]/div/p/div/button'
Industry_dict = {'Industry_Fragrances' : '//*[@class="ais-RefinementList-checkbox" and @value="Fragrances"]',
'Industry_Cosmetics' : '//*[@class="ais-RefinementList-checkbox" and @value="Cosmetics"]',
'Industry_Fashion' : '//*[@class="ais-RefinementList-checkbox" and @value="Fashion"]',
'Industry_NaturalPerfumery': '//*[@class="ais-RefinementList-checkbox" and @value="Natural Perfumery"]',
'Industry_Celebrity' : '//*[@class="ais-RefinementList-checkbox" and @value="Celebrity"]',
'Industry_Accessories' : '//*[@class="ais-RefinementList-checkbox" and @value="Accessories"]',
'Industry_Jewelry': '//*[@class="ais-RefinementList-checkbox" and @value="Jewelry"]',
'Industry_Retailer': '//*[@class="ais-RefinementList-checkbox" and @value="Retailer"]',
'Industry_Lingerie': '//*[@class="ais-RefinementList-checkbox" and @value="Lingerie"]',
'Industry_NichePerfumes': '//*[@class="ais-RefinementList-checkbox" and @value="Niche Perfumes"]'
}
Gender_dict = {'Gender_Female' : '//*[@class="ais-RefinementList-checkbox" and @value="female"]',
'Gender_Unisex' : '//*[@class="ais-RefinementList-checkbox" and @value="unisex"]',
'Gender_Male' : '//*[@class="ais-RefinementList-checkbox" and @value="male"]'
}
Country_more = '//*[@id="offCanvasLeftOverlap1"]/div/div/div[10]/div[2]/div/p/div/button'
Country_dict = {'Country_USA' : '//*[@class="ais-RefinementList-checkbox" and @value="United States"]',
'Country_France' : '//*[@class="ais-RefinementList-checkbox" and @value="France"]',
'Country_Italy' : '//*[@class="ais-RefinementList-checkbox" and @value="Italy"]',
'Country_UK' : '//*[@class="ais-RefinementList-checkbox" and @value="United Kigdom"]',
'Country_Brazil' : '//*[@class="ais-RefinementList-checkbox" and @value="Brazil"]',
'Country_UAE' : '//*[@class="ais-RefinementList-checkbox" and @value="United Arab Emirates"]',
'Country_Russia': '//*[@class="ais-RefinementList-checkbox" and @value="Russia"]',
'Country_Spain' : '//*[@class="ais-RefinementList-checkbox" and @value="Spain"]',
'Country_Germany' : '//*[@class="ais-RefinementList-checkbox" and @value="Germany"]',
'Country_SaudiArabia' : '//*[@class="ais-RefinementList-checkbox" and @value="Saudi Arabia"]',
'Country_Sweden' : '//*[@class="ais-RefinementList-checkbox" and @value="Sweden"]',
'Country_Latvia' : '//*[@class="ais-RefinementList-checkbox" and @value="Latvia"]',
'Country_Poland' : '//*[@class="ais-RefinementList-checkbox" and @value="Poland"]',
'Country_Japan' : '//*[@class="ais-RefinementList-checkbox" and @value="Japan"]',
'Country_Netherlands' : '//*[@class="ais-RefinementList-checkbox" and @value="Netherlands"]',
'Country_Canada' : '//*[@class="ais-RefinementList-checkbox" and @value="Canada"]',
'Country_Australia' : '//*[@class="ais-RefinementList-checkbox" and @value="Australia"]',
'Country_Switzerland' : '//*[@class="ais-RefinementList-checkbox" and @value="Switzerland"]',
'Country_Argentina' : '//*[@class="ais-RefinementList-checkbox" and @value="Argentina"]',
'Country_Belgium' : '//*[@class="ais-RefinementList-checkbox" and @value="Belgium"]',
'Country_Pakistan' : '//*[@class="ais-RefinementList-checkbox" and @value="Pakistan"]',
'Country_Slovakia' : '//*[@class="ais-RefinementList-checkbox" and @value="Slovakia"]',
'Country_Austria' : '//*[@class="ais-RefinementList-checkbox" and @value="Austria"]',
'Country_Thailand' : '//*[@class="ais-RefinementList-checkbox" and @value="Thailand"]',
'Country_Philippines' : '//*[@class="ais-RefinementList-checkbox" and @value="Philippines"]',
'Country_SouthKorea' : '//*[@class="ais-RefinementList-checkbox" and @value="South Korea"]',
'Country_Belarus' : '//*[@class="ais-RefinementList-checkbox" and @value="Belarus"]',
'Country_Israel' : '//*[@class="ais-RefinementList-checkbox" and @value="Israel"]',
'Country_Korea' : '//*[@class="ais-RefinementList-checkbox" and @value="Korea"]',
'Country_Greece' : '//*[@class="ais-RefinementList-checkbox" and @value="Greece"]',
'Country_Denmark' : '//*[@class="ais-RefinementList-checkbox" and @value="Denmark"]',
'Country_Norway' : '//*[@class="ais-RefinementList-checkbox" and @value="Norway"]',
'Country_Portugal' : '//*[@class="ais-RefinementList-checkbox" and @value="Portugal"]',
'Country_Iceland' : '//*[@class="ais-RefinementList-checkbox" and @value="Iceland"]',
'Country_Newzealand' : '//*[@class="ais-RefinementList-checkbox" and @value="New Zealand"]',
'Country_Ukraine' : '//*[@class="ais-RefinementList-checkbox" and @value="Ukraine"]',
'Country_Romania' : '//*[@class="ais-RefinementList-checkbox" and @value="Romania"]'
}
start_year_xpath = '//*[@id="offCanvasLeftOverlap1"]/div/div/div[5]/div[2]/div/p/div/form/input[1]'
end_year_xpath = '//*[@id="offCanvasLeftOverlap1"]/div/div/div[5]/div[2]/div/p/div/form/input[2]'
load_more_button = '//*[@id="main-content"]/div[1]/div[1]/div/div/div/div[2]/div[1]/div/div[3]/div/div/div/div/div/button'
clear_filters_button = '//*[@id="offCanvasLeftOverlap1"]/div/div/div[1]/div[2]/p/div/button'
industry_load = WebDriverWait(driver,20).until(EC.element_to_be_clickable((By.XPATH, Industry_more)))
driver.execute_script("arguments[0].click();", industry_load)
country_load = WebDriverWait(driver,20).until(EC.element_to_be_clickable((By.XPATH, Country_more)))
driver.execute_script("arguments[0].click();", country_load)
def start_scraping():
elements = driver.find_elements(by = By.XPATH, value = '//*[@class="cell card fr-news-box"]')
if len(elements) == 0:
return []
elif len(elements) < 30:
return elements
else:
prev_count = len(elements)
loading = WebDriverWait(driver, 100).until(EC.element_to_be_clickable((By.XPATH, load_more_button)))
driver.execute_script("arguments[0].click();", loading)
time.sleep(2)
elements = driver.find_elements(by = By.XPATH, value = '//*[@class="cell card fr-news-box"]')
now_count = len(elements)
while now_count > prev_count:
loading = WebDriverWait(driver, 100).until(EC.element_to_be_clickable((By.XPATH, load_more_button)))
driver.execute_script("arguments[0].click();", loading)
time.sleep(2)
elements = driver.find_elements(by = By.XPATH, value = '//*[@class="cell card fr-news-box"]')
prev_count = now_count
now_count = len(elements)
return elements
all_links = []
# def scrape():
# links = []
# try:
# loadingButton = WebDriverWait(driver,100).until(EC.element_to_be_clickable((By.XPATH,load_more_button)))
# maxPerfumes = 1000;
# cond = True
# while loadingButton:
# time.sleep(3)
# driver.execute_script("arguments[0].click();", loadingButton)
# loadingButton = WebDriverWait(driver,100).until(EC.element_to_be_clickable((By.XPATH,load_more_button)))
# loadElems = driver.find_elements(by = By.XPATH, value = '//*[@class="cell card fr-news-box"]')
# if len(loadElems)>0:
# loadingButton = WebDriverWait(driver,100).until(EC.element_to_be_clickable((By.XPATH,load_more_button)))
# else:
# cond = False
# break
# if len(loadElems) >= maxPerfumes:
# break
# if cond :
# card_sections = [] # list of card sections (image section and fragrance home page section)
# sections = driver.find_elements(by=By.CLASS_NAME, value="card-section")
# for section in sections:
# card_sections.append(section)
# home_pages = card_sections[1::2] # Extracting fragrance home page link section from all sections (image section and fragrance home page section)
# links = [] # list of final links when extracted.
# inc = 0 # Increment value to get each card_seection
# # Looping through to get all links
# for link in home_pages:
# link = home_pages[inc] # Getting the each div section
# link = link.find_elements(by=By.TAG_NAME, value = "p")
# link = link[0] # Selecting first paragraph (the one housing the link) element out of two
# link = link.find_elements(by=By.TAG_NAME, value = "a")
# link = link[0].get_attribute("href") # Extracting link from list
# links.append(link)
# inc +=1
# WebDriverWait(driver, 20).until(EC.element_to_be_clickable((By.XPATH,clear_filters_button))).click()
# all_links = all_links+links
# except:
# pass
all_cards = []
def filter():
for industry in Industry_dict.values():
for gender in Gender_dict.values():
for country in Country_dict.values():
for year in range(1920, 2023):
industry_selected = WebDriverWait(driver, 100).until(EC.element_to_be_clickable((By.XPATH, industry)))
driver.execute_script("arguments[0].click();", industry_selected)
time.sleep(2)
gender_selected = WebDriverWait(driver, 100).until(EC.element_to_be_clickable((By.XPATH, gender)))
driver.execute_script("arguments[0].click();", gender_selected)
time.sleep(2)
try:
country_selected = WebDriverWait(driver, 100).until(EC.element_to_be_clickable((By.XPATH, country)))
driver.execute_script("arguments[0].click();", country_selected)
time.sleep(2)
except NoSuchElementException:
break
start = WebDriverWait(driver, 100).until(EC.element_to_be_clickable((By.XPATH, start_year_xpath)))
start.clear()
driver.execute_script("arguments[0].click();", start)
start.send_keys(year)
time.sleep(1)
end = WebDriverWait(driver, 100).until(EC.element_to_be_clickable((By.XPATH, end_year_xpath)))
end.clear()
driver.execute_script("arguments[0].click();", end)
end.send_keys(year)
time.sleep(2)
# start of new code
all_cards.append(start_scraping())
print(len(all_cards)) # to keep track.
# end of new code
clear = WebDriverWait(driver, 50).until(EC.element_to_be_clickable((By.XPATH, clear_filters_button)))
driver.execute_script("arguments[0].click();", clear)
filter()
print(len(all_cards))
關於如何解決這個問題的任何建議? TIA
Chrome 99 bugs.chromium Issue 4048: target frame detached
中引入了一個問題。 見評論#53
狀態:已修復(原為:已分配)
ChromeDriver 103 中修復了框架分離問題
您使用的是版本 102,請更新您的瀏覽器和驅動程序版本。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.