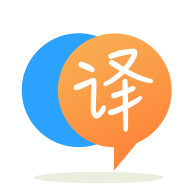
[英]How to replace a React component with another one when its state is changed
[英]How to replace react component with another when event triggered in that component
所以,我(Total react 初學者)有這個 React 組件。
import './cryptography.css';
import {useRef} from 'react';
import useInterval from 'react-useinterval';
import { useState } from 'react';
const DisplayTimer = () => {
const [time, setTime] = useState(0);
useInterval(() => {
setTime(time + 1);
}
, 1000);
const hours = Math.floor(time / 3600);
const minutes = Math.floor((time % 3600) / 60);
const seconds = time % 60;
return (
<div className="timer">
<h3>Timer</h3>
<p>{hours.toString().padStart(2, '0')}:{minutes.toString().padStart(2, '0')}:{seconds.toString().padStart(2, '0')}</p>
</div>
)
}
const CryptoOne = () => {
const inputRef = useRef();
function verifyAnswer() {
if (inputRef.current.value === "c6e24b99a8054ab24dd0323530b80819") {
alert("Correct!");
}
else {
alert("Wrong!");
}
}
return (
<div className="crypto-one">
<h3>Level One</h3>
<p>Convert this plaintext into an MD5 hash - "RollingStonesGatherNoMoss"</p>
<input ref={inputRef} type="text" id="one-answer" name="one-answer" />
<button onClick={verifyAnswer}>Submit</button>
</div>
)
}
const CryptoTwo = () => {
const inputRef = useRef();
function verifyAnswer() {
if (inputRef.current.value === "IaMaHackerManHaHa") {
alert("Correct!");
}
else {
alert("Wrong!");
}
}
return (
<div className="crypto-two">
<h3>Level Two</h3>
<p>Decode Caesar cipher into plaintext - "FxJxExzhboJxkExEx"</p>
<input ref={inputRef} type="text" id="two-answer" name="two-answer" />
<button onClick={verifyAnswer}>Submit</button>
</div>
)
}
const CryptoThree = () => {
const inputRef = useRef();
function verifyAnswer() {
let input = inputRef.current.value;
let answer = "SHA256".toLowerCase();
if (input === answer) {
alert("Correct!, completed the exercise");
}
else {
alert("Wrong!");
}
}
return (
<div className="crypto-three">
<h3>Level Three</h3>
<p>Identify the type of the hash (Type only the hash type, with no spaces) - "e3b0c44298fc1c149afbf4c8996fb92427ae41e4649b934ca495991b7852b855"</p>
<input ref={inputRef} type="text" id="three-answer" name="three-answer" />
<button onClick={verifyAnswer}>Submit</button>
</div>
)
}
const Cryptography = () => {
const [active, setActive] = useState("crypto-one");
return (
<div className="cryptography">
<h1>Cryptography</h1>
<DisplayTimer />
<div className="crypto-container">
<CryptoOne />
</div>
</div>
)
}
export { Cryptography };
在這里,我有這個密碼功能,它會顯示一個問題,如果它是正確的,那個問題將被另一個問題代替。 我有 3 個單獨的函數 CryptoOne、CryptoTwo 和 CryptoThree,它們將顯示在函數 Cryptography 中。 這意味着如果 CryptoOne 問題的答案是正確的,那么它將被 CryptoTwo 替換,依此類推。 所以我的問題是我該怎么做?
提前致謝。
您可以在 Cryptography 組件中維護 activeIndex 狀態。 並且有一個 changeSlide 方法,每次答案正確時, activeSlideIndex
都會增加 1。 如下所示
const Cryptography = () => {
const [active, setActive] = useState("crypto-one");
const [activeIndex, setActiveIndex] = useState(1);
const incrementIndex = () => {
setActiveIndex(prevIndex => prevIndex + 1)
}
return (
<div className="cryptography">
<h1>Cryptography</h1>
<DisplayTimer />
<div className="crypto-container">
{activeSlideIndex === 1 && <CryptoOne changeIndex={incrementIndex} />}
{activeSlideIndex === 2 && <CryptoTwo changeIndex={incrementIndex} />}
{activeSlideIndex === 3 && <CryptoThree />}
</div>
</div>
)
}
您可以將此changeSlide()
方法作為道具傳遞給每個組件。 並在答案正確時調用它
const CryptoOne = ({changeIndex}) => {
const inputRef = useRef();
function verifyAnswer() {
if (inputRef.current.value === "c6e24b99a8054ab24dd0323530b80819") {
alert("Correct!");
changeIndex()
}
else {
alert("Wrong!");
}
}
return (
<div className="crypto-one">
<h3>Level One</h3>
<p>Convert this plaintext into an MD5 hash - "RollingStonesGatherNoMoss"</p>
<input ref={inputRef} type="text" id="one-answer" name="one-answer" />
<button onClick={verifyAnswer}>Submit</button>
</div>
)
}
const CryptoTwo = ({changeIndex}) => {
const inputRef = useRef();
function verifyAnswer() {
if (inputRef.current.value === "IaMaHackerManHaHa") {
alert("Correct!");
changeIndex()
}
else {
alert("Wrong!");
}
}
return (
<div className="crypto-two">
<h3>Level Two</h3>
<p>Decode Caesar cipher into plaintext - "FxJxExzhboJxkExEx"</p>
<input ref={inputRef} type="text" id="two-answer" name="two-answer" />
<button onClick={verifyAnswer}>Submit</button>
</div>
)
}
const CryptoThree = () => {
const inputRef = useRef();
function verifyAnswer() {
let input = inputRef.current.value;
let answer = "SHA256".toLowerCase();
if (input === answer) {
alert("Correct!, completed the exercise");
}
else {
alert("Wrong!");
}
}
return (
<div className="crypto-three">
<h3>Level Three</h3>
<p>Identify the type of the hash (Type only the hash type, with no spaces) - "e3b0c44298fc1c149afbf4c8996fb92427ae41e4649b934ca495991b7852b855"</p>
<input ref={inputRef} type="text" id="three-answer" name="three-answer" />
<button onClick={verifyAnswer}>Submit</button>
</div>
)
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.