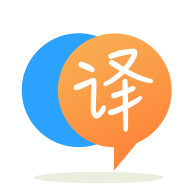
[英]Permission Denial: opening provider com.android.providers.media.MediaDocumentsProvider when RESTARTING the app
[英]Permission Denial: opening provider com.android.providers.contacts.ContactsProvider2 Read_Contacts and I don't have a write permission
我嘗試從 android 網站https://android-doc.github.io/training/contacts-provider/retrieve-names.html#Permissions構建檢索聯系人列表,並嘗試將其配置為 31 個目標 api。 我收到了這個錯誤
E / AndroidRuntime:致命異常:ModernAsyncTask#1進程:com.example.contactlistandroid,PID:15844 java.lang.RuntimeException:執行doInBackground()時發生錯誤原因:java.lang.SecurityException:權限拒絕:打開提供程序com .android.providers.contacts.ContactsProvider2 來自 ProcessRecord{b4e47ee 15844:com.example.contactlistandroid/u0a160} (pid=15844, uid=10160) 需要 android.permission.READ_CONTACTS 或 android.permission.WRITE_CONTACTS
這是我的主要活動
/*
* Copyright (C) 2013 The Android Open Source Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.example.contactlistandroid.ui;
import android.app.SearchManager;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import androidx.fragment.app.FragmentActivity;
import com.example.contactlistandroid.BuildConfig;
import com.example.contactlistandroid.R;
import com.example.contactlistandroid.util.Utils;
/**
* FragmentActivity to hold the main {@link ContactsListFragment}. On larger screen devices which
* can fit two panes also load {@link ContactDetailFragment}.
*/
public class ContactsListActivity extends FragmentActivity implements
ContactsListFragment.OnContactsInteractionListener {
// Defines a tag for identifying log entries
private static final String TAG = "ContactsListActivity";
private ContactDetailFragment mContactDetailFragment;
// If true, this is a larger screen device which fits two panes
private boolean isTwoPaneLayout;
// True if this activity instance is a search result view (used on pre-HC devices that load
// search results in a separate instance of the activity rather than loading results in-line
// as the query is typed.
private boolean isSearchResultView = false;
@Override
protected void onCreate(Bundle savedInstanceState) {
if (BuildConfig.DEBUG) {
Utils.enableStrictMode();
}
super.onCreate(savedInstanceState);
// Set main content view. On smaller screen devices this is a single pane view with one
// fragment. One larger screen devices this is a two pane view with two fragments.
setContentView(R.layout.activity_main);
// Check if two pane bool is set based on resource directories
isTwoPaneLayout = getResources().getBoolean(R.bool.has_two_panes);
// Check if this activity instance has been triggered as a result of a search query. This
// will only happen on pre-HC OS versions as from HC onward search is carried out using
// an ActionBar SearchView which carries out the search in-line without loading a new
// Activity.
if (Intent.ACTION_SEARCH.equals(getIntent().getAction())) {
// Fetch query from intent and notify the fragment that it should display search
// results instead of all contacts.
String searchQuery = getIntent().getStringExtra(SearchManager.QUERY);
ContactsListFragment mContactsListFragment = (ContactsListFragment)
getSupportFragmentManager().findFragmentById(R.id.contact_list);
// This flag notes that the Activity is doing a search, and so the result will be
// search results rather than all contacts. This prevents the Activity and Fragment
// from trying to a search on search results.
isSearchResultView = true;
mContactsListFragment.setSearchQuery(searchQuery);
// Set special title for search results
String title = getString(R.string.contacts_list_search_results_title, searchQuery);
setTitle(title);
}
if (isTwoPaneLayout) {
// If two pane layout, locate the contact detail fragment
mContactDetailFragment = (ContactDetailFragment)
getSupportFragmentManager().findFragmentById(R.id.contact_detail);
}
}
/**
* This interface callback lets the main contacts list fragment notify
* this activity that a contact has been selected.
*
* @param contactUri The contact Uri to the selected contact.
*/
@Override
public void onContactSelected(Uri contactUri) {
if (isTwoPaneLayout && mContactDetailFragment != null) {
// If two pane layout then update the detail fragment to show the selected contact
mContactDetailFragment.setContact(contactUri);
} else {
// Otherwise single pane layout, start a new ContactDetailActivity with
// the contact Uri
Intent intent = new Intent(this, ContactDetailActivity.class);
intent.setData(contactUri);
startActivity(intent);
}
}
/**
* This interface callback lets the main contacts list fragment notify
* this activity that a contact is no longer selected.
*/
@Override
public void onSelectionCleared() {
if (isTwoPaneLayout && mContactDetailFragment != null) {
mContactDetailFragment.setContact(null);
}
}
@Override
public boolean onSearchRequested() {
// Don't allow another search if this activity instance is already showing
// search results. Only used pre-HC.
return !isSearchResultView && super.onSearchRequested();
}
}
mainfest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
package="com.example.contactlistandroid">
<!--
<uses-permission android:name="android.permission.READ_CONTACTS" />
-->
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.ContactListAndroid"
tools:targetApi="31">
<activity
android:name=".ui.ContactsListActivity"
android:windowSoftInputMode="adjustResize"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
<!-- Add intent-filter for search intent action and specify searchable configuration
via meta-data tag. This allows this activity to receive search intents via the
system hooks. In this sample this is only used on older OS versions (pre-Honeycomb)
via the activity search dialog. See the Search API guide for more information:
http://developer.android.com/guide/topics/search/search-dialog.html -->
<intent-filter>
<action android:name="android.intent.action.SEARCH" />
</intent-filter>
<meta-data android:name="android.app.searchable"
android:resource="@xml/searchable_contacts" />
</activity>
<activity
android:name=".ui.ContactDetailActivity"
android:label="@string/activity_contact_detail"
android:parentActivityName=".ui.ContactsListActivity">
<!-- Define hierarchical parent of this activity, both via the system
parentActivityName attribute (added in API Level 16) and via meta-data annotation.
This allows use of the support library NavUtils class in a way that works over
all Android versions. See the "Tasks and Back Stack" guide for more information:
http://developer.android.com/guide/components/tasks-and-back-stack.html
-->
<meta-data android:name="android.support.PARENT_ACTIVITY"
android:value=".ui.ContactsListActivity" />
</activity>
</application>
</manifest>
感謝您的幫助
您需要請求許可並驗證它是否被我認為的廣播監聽器允許
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.