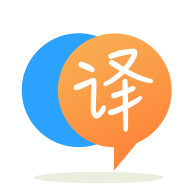
[英]Recursively walk through an array/object with render functions with javascript
[英]How to walk through the object/array in javascript by layers
我將嵌套數據 object 轉換為 arrays,以便 UI 庫顯示數據之間的關系。
原來的
// assume that all object key is unique
{
"top":{
"test":{
"hello":"123"
},
"test2":{
"bye":"123"
"other":{
...
...
...
}
}
}
}
首選結果
[
{
id:"top",
parent: null,
},
{
id:"test",
parent: "top",
},
{
id:"hello",
parent: "test",
},
{
id:"test2",
parent: "top",
},
]
為此,我編寫如下代碼:
const test = []
const iterate = (obj, parent = null) => {
Object.keys(obj).forEach(key => {
const id = typeof obj[key] === 'object' ? key : obj[key]
const loopObj = {
id,
parent
}
test.push(loopObj)
if (typeof obj[key] === 'object') {
iterate(obj[key], id)
}
})
}
iterate(data)
console.log(test) // Done!!
有用。 但是,我錯過了一件重要的事情,圖書館需要原始數據中的層,以確定類型/ function 做什么。
// The key name maybe duplicated in different layer
{
"top":{ // Layer 1
"test":{ // Layer 2
"hello":"123", // Layer 3
"test":"123" // Layer 3
// Maybe many many layers...
}
}
}
[
{
id:"top",
display:"0-top",
parent: null,
layer: 0
},
{
id: "1-top-test", // To prevent duplicated id, `${layer}-${parentDisplay}-${display}`
display:"test",
parent: "0-top",
parentDisplay: "top",
layer: 1
},
{
id: "3-test-test", // To prevent duplicated id,`${layer}-${parentDisplay}-${display}`
display:"test",
parent: "2-top-test",
parentDisplay: "test",
layer: 3
}
]
編輯display
或者id
格式很簡單,就是編輯function並添加字段,但是不知道如何輕松獲取圖層。
我嘗試在外部添加let count = 0
並在iterate
調用 function 時執行count++
。但我意識到當檢測到 object 時它會命中,而不是分層。
原始數據可能很大,所以我覺得編輯原始數據結構或者在test[]
每次循環中搜索父 id 可能都不是一個好的解決方案。
有什么解決方案可以做到這一點嗎?
只需將當前深度作為參數添加到每個遞歸調用(以及父名稱)上。
const input = { "top":{ "test":{ "hello":"123" }, "test2":{ "bye":"123", "other":{ } } } }; const iterate = (obj, result = [], layer = 0, parentId = null, parentDisplay = '') => { Object.entries(obj).forEach(([key, value]) => { const id = `${layer}-${key}`; result.push({ id, display: key, parentId, parentDisplay, layer, }); if (typeof value === 'object') { iterate(value, result, layer + 1, id, key); } }); return result; } console.log(iterate(input));
也就是說,如果在同一級別存在兩個具有不同祖父對象但其父對象使用相同鍵的對象,則您所需的方法仍然會產生重復條目,例如:
const input = {
"top1":{
"test":{
"hello":"123"
},
},
"top2": {
"test": {
"hello":"123"
}
}
};
const input = { "top1":{ "test":{ "hello":"123" }, }, "top2": { "test": { "hello":"123" } } }; const iterate = (obj, result = [], layer = 0, parentId = null, parentDisplay = '') => { Object.entries(obj).forEach(([key, value]) => { const id = `${layer}-${key}`; result.push({ id, display: key, parentId, parentDisplay, layer, }); if (typeof value === 'object') { iterate(value, result, layer + 1, id, key); } }); return result; } console.log(iterate(input));
如果這是一個問題,請考慮傳遞訪問屬性所需的整個訪問器字符串 - 例如top1.test.hello
和top2.test.hello
,它們保證是唯一的。
const input = { "top1":{ "test":{ "hello":"123" }, }, "top2": { "test": { "hello":"123" } } }; const iterate = (obj, result = [], parentAccessor = '') => { Object.entries(obj).forEach(([key, value]) => { const accessor = `${parentAccessor}${parentAccessor? '.': ''}${key}`; result.push({ id: key, accessor, }); if (typeof value === 'object') { iterate(value, result, accessor); } }); return result; } console.log(iterate(input));
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.