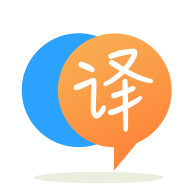
[英]Jackson XML with different element name and attributes under root tag
[英]How to use Jackson to map XML child entries with a root element that has attributes
假設我們有以下 XML 文件:
<?xml version="1.0" encoding="UTF-8" ?>
<car>
<name>Speedy VW Golf</name>
<tires>
<tire>
<value>Michelin FL</value>
</tire>
<tire>
<value>Michelin FR</value>
</tire>
<tire>
<value>Michelin BL</value>
</tire>
<tire>
<value>Michelin BR</value>
</tire>
</tires>
<component componentId="compId", serialId="lalal">
<frame frameId="1234-56789-0" vendor="Steel MC">
<item vendorName="Foo" country="Italy"/>
<item vendorName="Bar" country="Germany"/>
<item vendorName="Foobar" country="China"/>
</frame>
</component>
</car>
我怎樣才能 map 所有item
兒童? 以下Java代碼:
package com.acme.carsample;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.dataformat.xml.XmlMapper;
import com.fasterxml.jackson.dataformat.xml.annotation.JacksonXmlElementWrapper;
import com.fasterxml.jackson.dataformat.xml.annotation.JacksonXmlProperty;
import com.fasterxml.jackson.dataformat.xml.annotation.JacksonXmlRootElement;
import java.io.File;
import java.nio.file.Files;
import java.util.List;
public class CarReader {
@JsonIgnoreProperties(ignoreUnknown = true)
public static class Car {
private String name;
private List<Tire> tires;
private Component component;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public List<Tire> getTires() {
return tires;
}
public void setTires(List<Tire> tires) {
this.tires = tires;
}
public Component getComponent() {
return component;
}
public void setComponent(Component component) {
this.component = component;
}
}
@JsonIgnoreProperties(ignoreUnknown = true)
public static class Tire {
private String value;
public String getValue() {
return value;
}
public void setValue(String value) {
this.value = value;
}
}
@JsonIgnoreProperties(ignoreUnknown = true)
public static class Component {
private Frame frame;
public Frame getFrame() {
return frame;
}
public void setFrame(Frame frame) {
this.frame = frame;
}
}
@JsonIgnoreProperties(ignoreUnknown = true)
public static class Frame {
@JacksonXmlProperty(isAttribute = true)
private String frameId;
@JacksonXmlProperty(isAttribute = true)
private String vendor;
@JacksonXmlElementWrapper(useWrapping = false)
private List<Item> items;
public String getFrameId() {
return frameId;
}
public void setFrameId(String frameId) {
this.frameId = frameId;
}
public String getVendor() {
return vendor;
}
public void setVendor(String vendor) {
this.vendor = vendor;
}
public List<Item> getItems() {
return items;
}
public void setItems(List<Item> items) {
this.items = items;
}
}
@JsonIgnoreProperties(ignoreUnknown = true)
@JacksonXmlRootElement(localName = "item")
public static class Item {
@JacksonXmlProperty(isAttribute = true)
private String vendorName;
@JacksonXmlProperty(isAttribute = true)
private String country;
public String getVendorName() {
return vendorName;
}
public void setVendorName(String vendorName) {
this.vendorName = vendorName;
}
public String getCountry() {
return country;
}
public void setCountry(String country) {
this.country = country;
}
}
public static void main(String[] arguments) {
try {
// Deserialize the car object (XML to Java)
String data = new String(Files.readAllBytes(new File("Car.xml").toPath()));
XmlMapper xmlMapper = new XmlMapper();
Car car = xmlMapper.readValue(data, Car.class);
// Serialize the car object (Java to json)
ObjectMapper objectMapper = new ObjectMapper();
data = objectMapper.writerWithDefaultPrettyPrinter().writeValueAsString(car);
System.out.println(data);
} catch (Exception exception) {
System.err.println("An error occurred: " + exception.getMessage());
}
}
}
結果如下 JSON output ( item
子屬性未映射為列表,而是null
:
{
"name" : "Speedy VW Golf",
"tires" : [ {
"value" : "Michelin FL"
}, {
"value" : "Michelin FR"
}, {
"value" : "Michelin BL"
}, {
"value" : "Michelin BR"
} ],
"component" : {
"frame" : {
"frameId" : "1234-56789-0",
"vendor" : "Steel MC",
"items" : null
}
}
}
解決方案是將@JacksonXmlRootElement
class 注釋從Item
class 移動到Frame
中的字段變量items
:
@JacksonXmlProperty(localName = "item")
@JacksonXmlElementWrapper(useWrapping = false)
private List<Item> items;
之后正確讀取子項。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.