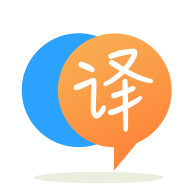
[英]How to cast, in C++, a byte vector (std::vector<uint8_t>) into different uint32_t, uint16_t and uint8_t variables
[英]C++ vector of uint8_t to vector of uint32_t
std::vector<uint8_t> vector1 = { 1, 2, 3, 4 };
我想將上面的向量轉換為 uint32_t 版本。 我試着做:
std::vector<uint32_t> vector2(vector1.begin(), vector2.end());
但是這段代碼在 32 位版本中返回相同的數組,所以內容仍然是 { 1, 2, 3, 4 }。
我期望得到的是一個包含單個值(在本例中)為 0x01020304 的數組。
有什么方法可以在不使用 for 循環的情況下實現這一目標?
謝謝你。
編輯:
我的解決方案,不知道這是否是一個好習慣,但從我對向量的了解來看,它應該是完全有效的:
std::vector<uint32_t> vector2((uint32_t*)vector1.data(), (uint32_t*)(vector1.data() + vector1.size()*sizeof(uint8_t)));
為簡單起見,我建議使用循環來執行此操作
#include <cstdint>
#include <exception>
#include <vector>
std::uint32_t
combine(std::uint8_t a,
std::uint8_t b,
std::uint8_t c,
std::uint8_t d)
{
return (std::uint32_t(a) << 24) |
(std::uint32_t(b) << 16) |
(std::uint32_t(c) << 8) |
std::uint32_t(d);
}
std::vector<std::uint32_t>
combine_vector(const std::vector<std::uint8_t>& items)
{
if (items.size() % 4 != 0)
throw std::exception();
std::vector<std::uint32_t> ret;
ret.reserve(items.size() / 4);
for (std::size_t i = 0; i < items.size(); i += 4) {
ret.push_back(
combine(items[i + 0],
items[i + 1],
items[i + 2],
items[i + 3]));
}
return ret;
}
我懷疑您需要實現自定義迭代器類型才能在不使用原始循環的情況下執行此操作。
我做了這樣一個迭代器
#include <iterator>
class combine_iterator
{
public:
using value_type = std::uint32_t;
using reference = const value_type&;
using pointer = const value_type*;
using difference_type = std::ptrdiff_t;
using iterator_category = std::input_iterator_tag;
combine_iterator(const std::uint8_t* data, std::size_t count) :
m_data(data)
{
if (count % 4 != 0)
throw std::exception();
if (count > 0)
++*this;
}
reference
operator*() const
{
return m_cur_val;
}
pointer
operator->() const
{
return &m_cur_val;
}
friend combine_iterator&
operator++(combine_iterator& rhs)
{
std::uint8_t a = *(rhs.m_data++);
std::uint8_t b = *(rhs.m_data++);
std::uint8_t c = *(rhs.m_data++);
std::uint8_t d = *(rhs.m_data++);
rhs.m_cur_val = combine(a, b, c, d);
return rhs;
}
friend combine_iterator
operator++(combine_iterator& lhs, int)
{
auto cp = lhs;
++lhs;
return cp;
}
friend bool
operator!=(const combine_iterator& lhs, const combine_iterator& rhs)
{
return (lhs.m_data != rhs.m_data);
}
private:
const std::uint8_t* m_data;
std::uint32_t m_cur_val;
};
int main()
{
std::vector<std::uint8_t> data = { 1, 2, 3, 4, 5, 6, 7, 8 };
std::vector<std::uint32_t> res(
combine_iterator(data.data(), data.size()),
combine_iterator(data.data() + data.size(), 0));
}
迭代器至少包含一個錯誤。 我將這些錯誤作為教育課程留下來,為什么使用循環通常比實現自定義迭代器更容易糾正。 理想情況下,僅當我們創建需要它的容器時才應創建自定義迭代器,否則創建自定義迭代器的心理開銷是合理的。
您可以通過使用 range-v3 庫或 C++20 std::ranges 來使用范圍。
std::vector<uint8_t> vector1 = { 1, 2, 3, 4, 5, 6, 7, 8 };
std::vector<uint32_t> vec2 =
vector1
| view::chunk(4)
| views::transform(
[](auto&& range){
return accumulate(
range,
static_cast<uint32_t>(0),
[](auto acc, auto i)
{
return acc << 8 | i;
}
);
})
| to<std::vector>();
vec2
將包含 {0x1020304, 0x5060708}。
在godbolt上看到它: https://godbolt.org/z/6z6ehfKbq
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.