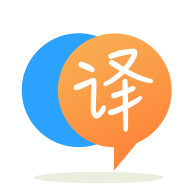
[英]Dust js How to check whether the condition is true at least once in a loop
[英]Check if at least one condition is true in react js
我有一個 object tableRows 數組。
tableRows = [
{
purchase_sales:1000,
yearFirstRemAmount: 1456,
capitalServicePurchase:123234,
otherServicePurchase: 12323,
otherStuffPurchase: 8903,
capitaStuffPurchase: 1200,
currentYearServiceSell: 47856,
currentYearStuffSell: 100000,
yearLastRemAmount: 20000
}
{
purchase_sales:23430,
yearFirstRemAmount: 12500,
capitalServicePurchase: 1000010,
otherServicePurchase: 12360,
otherStuffPurchase: 12300,
capitaStuffPurchase: 12000,
currentYearServiceSell: 123123,
currentYearStuffSell: 12111,
yearLastRemAmount: 13120
}
]
如何檢查每個索引的 9 個密鑰對值中的至少一個是否大於或等於 100000。
下面的代碼不起作用:
const handleValidation = (index)=>{
if(tableRows[index].purchase_sales<100000 || tableRows[index].yearFirstRemAmount<100000 || tableRows[index].capitalServicePurchase<100000 || tableRows[index].otherServicePurchase<100000 || tableRows[index].otherStuffPurchase<100000 || tableRows[index].capitaStuffPurchase<100000 || tableRows[index].currentYearServiceSell<100000 || tableRows[index].currentYearStuffSell<100000 || tableRows[index].yearLastRemAmount<100000 ){
alert("'At least one amount should be greater than or equal to 100000!!!")
}
}
有沒有更好更簡潔的方法來實現這一點?
您可以結合使用map
和some
:
const threshold = 1000000;
const yourAnswer = tableRows.map(Object.values).some(value => value >= threshold);
const handleValidation = (index)=>{
if (Object.values(tableRows[index]).some(value => value < 100000)) {
alert("'At least one amount should be greater than or equal to 100000!!!")
}
}
您可以使用filter
方法過濾數組, Object.values
每個 object 的鍵值,以及some
檢查是否存在等於或大於100000
的鍵
const tableRows = [ { purchase_sales:1000, yearFirstRemAmount: 1456, capitalServicePurchase:123234, otherServicePurchase: 12323, otherStuffPurchase: 8903, capitaStuffPurchase: 1200, currentYearServiceSell: 47856, currentYearStuffSell: 100000, yearLastRemAmount: 20000 }, { purchase_sales:23430, yearFirstRemAmount: 12500, capitalServicePurchase: 1000010, otherServicePurchase: 12360, otherStuffPurchase: 12300, capitaStuffPurchase: 12000, currentYearServiceSell: 123123, currentYearStuffSell: 12111, yearLastRemAmount: 13120 } ] const rows = tableRows.filter(row => { return Object.values(row).some(value => value >= 100000) }) console.log(rows)
如果您已經知道tableRows[index]
的所有條目都是要檢查的數字,則可以使用Object.prototype.values
和Array.prototype.some
(這也是短路)
const tableRows=[{purchase_sales:1000,yearFirstRemAmount:1456,capitalServicePurchase:123234,otherServicePurchase:12323,otherStuffPurchase:8903,capitaStuffPurchase:1200,currentYearServiceSell:47856,currentYearStuffSell:100000,yearLastRemAmount:20000},{purchase_sales:23430,yearFirstRemAmount:12500,capitalServicePurchase:1000010,otherServicePurchase:12360,otherStuffPurchase:12300,capitaStuffPurchase:12000,currentYearServiceSell:123123,currentYearStuffSell:12111,yearLastRemAmount:13120},{purchase_sales:0,yearFirstRemAmount:0,capitalServicePurchase:0,otherServicePurchase:0,otherStuffPurchase:0,capitaStuffPurchase:0,currentYearServiceSell:0,currentYearStuffSell:0,yearLastRemAmount:0}]; const handleValidation = (index) => { if (.Object.values(tableRows[index]).some((v) => v >= 100000)) { alert("'At least one amount should be greater than or equal to 100000,;! for index " + index) } } tableRows.forEach((_row, idx) => handleValidation(idx));
如其他答案中所述,您可以將 object 轉換為數組或將其值轉換為數組,然后在數組上使用some
方法。
但是如果您的 object 可能有其他鍵並且您想檢查特定屬性:
const keys = [
'purchase_sales',
'yearFirstRemAmount',
'capitalServicePurchase',
'otherServicePurchase',
'otherStuffPurchase',
'capitaStuffPurchase',
'currentYearServiceSell',
'currentYearStuffSell',
'yearLastRemAmount'
];
function validate (obj) {
for (key of keys) {
if(obj[key] >= 100000) {
// Do something here.
// For instance: return true;
}
}
// There is no property with a value greater or equal to 100000.
// Do something here.
// For instance: return false;
}
const isValid = tableRows.map(validate).every(Boolean)
const tableRows = [
{
purchase_sales:1000,
yearFirstRemAmount: 1456,
capitalServicePurchase:123234,
otherServicePurchase: 12323,
otherStuffPurchase: 8903,
capitaStuffPurchase: 1200,
currentYearServiceSell: 47856,
currentYearStuffSell: 100000,
yearLastRemAmount: 20000
},
{
purchase_sales:23430,
yearFirstRemAmount: 12500,
capitalServicePurchase: 1000010,
otherServicePurchase: 12360,
otherStuffPurchase: 12300,
capitaStuffPurchase: 12000,
currentYearServiceSell: 123123,
currentYearStuffSell: 12111,
yearLastRemAmount: 13120
}
];
const handleValidation = (index) => {
const found = Object.values(tableRows[index]).some((x) => x > 100000);
if(!found)
{
alert("'At least one amount should be greater than or equal to 100000!!!");
}
console.log(index,found)
}
tableRows.forEach((item, index) => handleValidation(index));
const tableRows = [
{
purchase_sales:1000,
yearFirstRemAmount: 1456,
capitalServicePurchase:123234,
otherServicePurchase: 12323,
otherStuffPurchase: 8903,
capitaStuffPurchase: 1200,
currentYearServiceSell: 47856,
currentYearStuffSell: 100000,
yearLastRemAmount: 20000
},
{
purchase_sales:23430,
yearFirstRemAmount: 12500,
capitalServicePurchase: 1000010,
otherServicePurchase: 12360,
otherStuffPurchase: 12300,
capitaStuffPurchase: 12000,
currentYearServiceSell: 123123,
currentYearStuffSell: 12111,
yearLastRemAmount: 13120
}
];
let data = tableRows.filter((row)=>{
return Object.values(row).map(value => value >= 100000)
});
console.log(data);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.