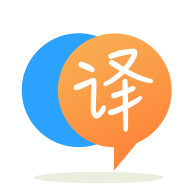
[英]How do I get an input from an Entry in Tkinter to be used in a function to be used in another window?
[英]How to get input from a tkinter entry box, to a variable in another function?
我正在 python 中編寫文件管理器。 功能之一是重命名文件。 我不想在終端中使用“input()”function 輸入文件名,而是要彈出一個輸入框,以便用戶可以在其中輸入文件名。
這是重命名文件的 function。
import os
import tkinter
from tkinter import filedialog
from tkinter import messagebox
def rename_file():
messagebox.showinfo(title="Rename File", message="Select the file you want to rename!")
renameFileSource = filedialog.askopenfilename()
if renameFileSource == "":
messagebox.showinfo(title="File Select Canceled", message="You have canceled the file selection!")
else:
try:
namePath = os.path.dirname(renameFileSource)
renameFileDestination = os.path.splitext(namePath)[1]
messagebox.showinfo(title="Rename File", message="Enter the new file name!")
entry_box()
if renameFileInput == "":
messagebox.showinfo(title="No input", message="You have not entered anything!")
else:
newPath = os.path.join(namePath, renameFileInput + renameFileDestination)
os.rename(renameFileSource, newPath)
messagebox.showinfo(title="File Renamed", message="The file has succesfully been renamed!")
except OSError as renameFileError:
messagebox.showerror(title="File Error", message="The following error has occured:\n\n"+ str(renameFileError) + "\n\nPlease try again!")
這是function,有我用tkinter制作的輸入框。 我希望這個 function 將輸入傳遞給 'rename_file()' function 中的變量 'renameFileInput'
def entry_box():
entryBox = tkinter.Tk()
def rename_input():
renameFileInput = entryInput.get()
entryBox.destroy()
tkinter.Label(entryBox, text="Enter data here: ").pack()
entryInput = tkinter.Entry(entryBox)
entryInput.pack()
button = tkinter.Button(entryBox, text="GO!", width=3, height=1, command=rename_input)
button.pack()
entryBox.mainloop()
這是具有我主要 GUI 的 function。
def gui_window():
guiWindow = tkinter.Tk()
tkinter.Label(guiWindow, text="Lucas' Files Manager\n", fg="green", font=("", 40, "normal", "underline")).pack()
tkinter.Button(guiWindow, text="Rename File", width=25, height=1, bg="blue", fg="red", font=("", "20", "bold"), command=rename_file).pack()
tkinter.Button(guiWindow, text="Exit Program", width=25, height=1, bg="blue", fg="red", font=("", "20", "bold"), command=guiWindow.destroy).pack()
guiWindow.mainloop()
gui_window()
當我運行如上所示的代碼時,我收到以下錯誤: NameError: name 'renameFileInput' is not defined。
我已將代碼上傳到我的整個文件管理器到 github: https://github.com/GierLucas/File-Manager
發生的情況是您的程序在創建變量renameFileInput
之前嘗試訪問它,因此引發了NameError
異常。 從您鏈接的代碼( 此處)中,您在 function rename_input
(位於entry_box
內部)中定義該變量,並嘗試在 function rename_file
中使用它。
問題是當 function 結束時, rename_input
中創建的變量被破壞,即它的 scope 結束時。 要獲得 rest 的代碼知道該值,您有幾個選擇。 建議return
該值,以便您可以將其傳輸到調用 function 的 scope。 另一種選擇是使該變量成為global
變量。 這意味着可以在代碼的每個部分中訪問變量。
要全球化 scope 中的變量,請在 function 的開頭鍵入global <variable>
,在您的情況下:
def entry_box():
entryBox = tkinter.Tk()
def callback():
global renameFileInput
renameFileInput = entryInput.get()
print(renameFileInput)
entryBox.destroy()
tkinter.Label(entryBox, text="Enter data here: ").pack()
entryInput = tkinter.Entry(entryBox)
entryInput.pack()
btn = tkinter.Button(entryBox, text="GO!", width=3, height=1, command=callback)
btn.pack()
entryBox.mainloop()
這應該可以解決您mainloop
的問題,盡管您的代碼有更多錯誤,從您在該方法中的主循環開始。 它不會讓您按預期保留應用程序。
要創建彈出窗口 windows,最好使用TopLevel
class。 請參閱此處的相關問題。
關於范圍
關於函數中的返回,請參見此處
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.