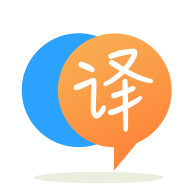
[英]Loop over an Array of Objects and display only object property at a time in HTML using Javascript
[英]Display Only 3 Objects at the time
我有一個報價生成器,我正在嘗試實現當時顯示特定數量對象的功能。 我試過使用 map,但它返回它不是 function。 現在我可以生成一個隨機報價,但我希望可以選擇顯示一個特定的數字。 任何幫助是極大的贊賞。 這是有效的方法,但我評論了試用 map。
應用程序.js
import { useState, useEffect } from "react";
import Footer from "./Components/Footer/Footer";
import Quote from "./Components/Quote/Quote";
import "./App.css"
import { data } from "./Components/Data/Data";
import { characterData } from "./Components/Data/CharacterData"
import CharacterBios from "./Components/CharacterBios/CharacterBios";
import Header from "./Components/Header/Header";
function App() {
const [quote, setQuote] = useState();
const [isLoading, setIsLoading] = useState(true);
const randomise = () => {
const randomNumber = Math.floor(Math.random() * data.length);
setQuote(data[randomNumber]);
};
//math.floor makes it a whole number, and the equation above goes through the data at random
useEffect(() => {
randomise();
setIsLoading(false);
}, []);
return (
<div className="App">
<Header />
<div className="main">
<h1>Quote Generator</h1>
{isLoading ? <p>Quote now loading...</p> : <Quote data={quote} />}
<button onClick={randomise}>Generate Quote</button>
<CharacterBios characterData={characterData} />
<Footer />
</div>
</div>
);
}
export default App;
報價單.jsx
import React from 'react'
const Quote = ({data}) => {
return (
<div className='container quote-section'>
<div className="row">
{data.slice(0).map((item,index)=> (
<div className="col-xl-4 col-lg-4 col-md-6 col-sm-12" key={index}>
<div className="marked-content-card">
<p><span className="name">{item.name}</span></p>
<p>{item.quote}</p>
</div>
</div>
))}
</div>
{/* <blockquote> {
data.quote
}
<p>-{
data.name
}</p>
</blockquote> */}
</div>
)
}
export default Quote;
以下是您可以執行的操作:
tempData = [...data]
tempQuotes
splice()
使用隨機索引從tempQuotes
中引用push
入tempQuotes
...
因為splice
返回一個數組tempData
中的剩余引號再次迭代 import { useState, useEffect } from "react"; const data = [ "Another one bites the dust", "Sweet dreams are made of this", "Never gonna give you up", "Highway to the danger zone", "Let the bodies hit the floor", "Hey now, I'm an allstar", "Hello darkness my old friend" ]; function App() { const [quotes, setQuotes] = useState([]); const [isLoading, setIsLoading] = useState(true); const numberOfQuotes = 3; const randomise = () => { const tempData = [...data]; const tempQuotes = []; for (let i = 0; i < numberOfQuotes; i++) { const randomNumber = Math.floor(Math.random() * tempData.length); tempQuotes.push(...tempData.splice(randomNumber, 1)); } setQuotes(tempQuotes); }; useEffect(() => { randomise(); setIsLoading(false); }, []); const quoteEls = quotes.map((quote) => <li class="quote">{quote}</li>); return ( <div className="App"> <div className="main"> <h1>Quote Generator</h1> {isLoading? <p>Quote now loading...</p>: quoteEls} <button onClick={randomise}>Generate Quote</button> </div> </div> ); } export default App;
如果data
很大,不想每次都克隆到 memory,可以:
Array.from()
一個索引數組,即[0,1,2,3]
splice
索引map
陣列到您的數據下面的 function 僅用於演示,但如果您決定這樣做,將很容易集成到您的代碼中。
const data = [ "Another one bites the dust", "Sweet dreams are made of this", "Never gonna give you up", "Highway to the danger zone", "Let the bodies hit the floor", "Hey now, I'm an allstar", "Hello darkness my old friend" ]; const numberOfQuotes = 3 function randomize() { const quoteIndices = [] const indices = Array.from({length: data.length}, (a,i) => i) // Make array of indices for (let i = 0; i < numberOfQuotes; i++){ let randomIndex = Math.floor(Math.random() * indices.length); quoteIndices.push(...indices.splice(randomIndex, 1)) } return quoteIndices.map(index => data[index]) } console.log(randomize())
因為data[randomNumber]
似乎是 object 而不是數組,所以會導致 Quote 出現問題。 jsx 因為你把它當作一個數組。
使用 lodash 的shuffle
function 來隨機化你的數組。
是的, .map
不是 function 因為數據數組包含quote
根據您在Quote
組件中的使用,並且您在數據數組中獲取錯誤值的原因是因為您正在設置data
數組。 即setQuote(data[randomNumber]);
因為randomNumber
給出了 integer。 您需要修改您的randomise
function 以在您的quote
state 中存儲一些隨機報價:
const randomise = () => {
const indices = new Set()
const N = 3 // specifies number of random items
while(true){
const randomNumber = Math.floor(Math.random()*data.length)
indices.add(randomNumber)
if(indices.size>=N) break;
}
let quotesArr = []
for(i in indices){
quotesArr.push(data[i])
}
setQuote(quotesArr);
};
上面的代碼不斷生成隨機數,直到你有N
個不同的隨機數,一旦你有了,你只需要在這些索引處選擇data
中的對象,然后設置引號。
然后,您還必須更新您的退貨聲明,特別是您使用Quote component
的部分:
<>{quote.map(q => <Quote data={q} />)}</>
您的問題基本上歸結為選擇所需數量的隨機(非重復)報價。 因此,您不必在App
state 中使用單引號,而是必須跟蹤引號數組(您仍將在useEffect()
中填充該數組)。
該概念的蒸餾實現可能如下所示:
const { render } = ReactDOM, { useState, useEffect } = React, root = document.getElementById('root') const quotesData = [ {id: 0, name: 'me', quote: 'oranges are cool'}, {id: 1, name: 'him', quote: 'bananas are sweet'}, {id: 2, name: 'someone', quote: 'apples can be soar'}, {id: 3, name: 'anyone', quote: 'I hate broccoli'}, {id: 4, name: 'me', quote: 'pinapples are juicy'} ] const randomQuoteMachine = (srcData, numberOfQuotes = 0) => { const quotes = [] while(quotes.length < numberOfQuotes){ const randomQuote = srcData[0|Math.random()*srcData.length] quotes.every(({id}) => id.== randomQuote.id) && quotes.push(randomQuote) } return quotes } const Quote = ({quote}) => { return ( <div> <h3>{quote.name}</h3> <p>{quote,quote}</p> </div> ) } const App = () => { const [ quotesList, setQuotesList ] = useState([]) useEffect(() => { const quotes = randomQuoteMachine(quotesData, 3) setQuotesList(quotes) }. []) return..quotesList.length && ( <div> { quotesList.map((quote) => <Quote {,:.{quote, key: quote.id}} />) } </div> ) } render( <App />, root )
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/16.12.0/umd/react.production.min.js"></script><script src="https://cdnjs.cloudflare.com/ajax/libs/react-dom/16.11.0/umd/react-dom.production.min.js"></script><div id="root"></div>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.