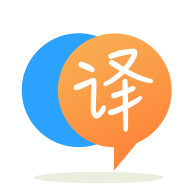
[英]Program producing a bus error when reading in using scanf - C Program
[英]Bus error while simply integer scanf sequence in C program
如果 C 中以下格式的輸入為 -1,我必須采用 integer 輸入並結束 while 循環:
111 1 1
111 1 1
111 1 1
-1
我的代碼在主 function(代碼結尾)中的第 3 次 scanf 后出現總線錯誤。 我知道這是關於空白的,但我不知道要解決它。 如果它很重要,我正在使用 M1 在 MacBook 上進行測試。 順便說一句 print("break")s 僅用於測試,我不想進行任何垃圾打印或掃描。
#include <stdio.h>
#include <stdlib.h>
struct employee{
int ID;
int freeAt;
int totalTime;
struct employee *next;
};
struct customer{
int ID;
int startTime;
int processTime;
int waitingTime;
int helper;
struct customer *front;
struct customer *rear;
struct customer *next;
};
typedef struct employee employee;
typedef struct customer customer;
void new_employer(employee *top, int id){ //Insert employees by id
employee *ptr;
ptr = (employee*)malloc(sizeof(employee));
ptr->ID = id;
ptr->freeAt = 0;
ptr->totalTime = 0;
if (top == NULL){
ptr->next = NULL;
top = ptr;
}
else{
ptr->next = top;
top = ptr;
}
}
void help_customer(customer *c, employee *top){ //Match customer with suitable employee
employee *ptr;
ptr = (employee*)malloc(sizeof(employee));
ptr = top;
if (top==NULL){
c->startTime = c->startTime + 1;
c->waitingTime++;
help_customer(c,ptr);
}
if(c->startTime>=top->freeAt){
c->helper = top->ID;
top->freeAt = c->startTime + c->processTime;
top->totalTime = top->totalTime + c->processTime;
}
else{
help_customer(c, top->next);
}
}
void new_customer(customer *c, employee *top, int id, int start, int process){ //New customer
customer *ptr;
ptr = (customer*)malloc(sizeof(customer));
ptr->ID = id;
ptr->startTime = start;
ptr->processTime = process;
ptr->waitingTime = 0;
help_customer(ptr,top);
if (c->front == NULL){
c->front = ptr;
c->rear = ptr;
c->next = NULL;
}
else{
c->rear->next = ptr;
c->rear = ptr;
c->rear->next = NULL;
}
}
void customerStats(customer *c){ //Printing customer stats at the end of the transactions
customer *ptr;
ptr = (customer*)malloc(sizeof(customer));
ptr = c->front;
if(ptr == NULL){
printf("\nQUEUE IS EMPTY");
}
else{
printf("\n");
while (ptr!=c->rear){
printf("%d ", ptr->ID);
printf("%d ", ptr->helper);
printf("%d ", ptr->startTime);
printf("%d ", ptr->processTime);
printf("%d\n", ptr->waitingTime);
ptr = ptr->next;
}
printf("%d ", ptr->ID);
printf("%d ", ptr->helper);
printf("%d ", ptr->startTime);
printf("%d ", ptr->processTime);
printf("%d\n", ptr->waitingTime);
}
}
void employeeStats(employee *top){ //Printing employee stats at the end of the transactions
employee *ptr;
ptr = (employee*)malloc(sizeof(employee));
ptr = top;
if (top==NULL){
printf("\nSTACK IS EMPTY");
}
else{
while (ptr!=NULL){
printf("%d ", ptr->ID);
printf("%d\n", ptr->totalTime);
ptr = ptr->next;
}
}
}
int main(){
employee *e;
for(int i=1;i>7;i++){
new_employer(e,i);
}
customer *c;
int id;
int start;
int process;
while(1){ //********I GET BUS ERROR HERE ON 3RD SCANF**************
scanf("%d", &id);
printf("break1");
if (id==-1){
break;
}
scanf("%d", &start);
printf("break2");
scanf("%d", &process);
printf("break3");
new_customer(c,e,id,start,process);
}
customerStats(c);
employeeStats(e);
}
這是終端:
111
break11
break21
zsh: bus error ./main
如果您一開始就編寫最少的代碼,並在添加更多代碼之前讓它工作,這會有所幫助。
這是一個部分更正,顯示了為什么您會出現未定義的行為(崩潰)。 閱讀每一行並了解它與您的代碼有何不同以及有何不同。 一旦理解了這一點,您就可以(謹慎地,一步一步地)一次再添加一個元素,並在朝着目標前進的過程中進行測試、測試、測試。
typedef struct employee {
int ID;
int freeAt;
int totalTime;
struct employee *next;
} employee_t;
employee_t *new_employeEEEE( employee_t *top, int id ) { //Insert employees by id
employee_t *ptr = (employee_t*)calloc( 1, sizeof(*ptr) ); // use calloc()
// Omitting check of calloc failing
ptr->ID = id;
ptr->next = top; // reverse sequence, but at least it works...
return ptr;
}
int main() {
employee_t *e = NULL;
// for( int i=1; i > 7;i++ ) !!!!
for( int i = 1; i < 7; i++ )
e = new_employeEEEE( e, i );
i = 1;
for( employee *p = e; p; p = p->next )
printf( "emp #%d has id: %d\n", i++, p->ID );
return 0;
}
Output:
emp #1 has id: 6
emp #2 has id: 5
emp #3 has id: 4
emp #4 has id: 3
emp #5 has id: 2
emp #6 has id: 1
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.