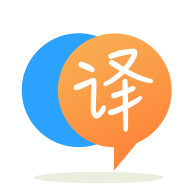
[英]how to console.log() longitudes and latitudes that are stored in mongoDB via mongoose
[英]how to check number of documents insert in MongoDB with Mongoose in console.log
我剛開始學習如何使用 MongoDB 和 Mongoose,所以我嘗試在 MongoDB 中插入一些文檔在本地運行,這就是我只使用 MongoDB 時的做法
const { MongoClient } = require("mongodb");
const uri = "mongodb://localhost:27017";
const client = new MongoClient(uri);
async function run() {
const dbName = client.db("fruitsDB");
try {
await client.connect();
// I insert the function to insert the docs here
await insertMultiDocs(dbName, docs);
// Establish and verify connection
await client.db("admin").command({ ping: 1 });
console.log("Connected successfully to server");
} finally {
await client.close();
}
}
run().catch(console.dir);
// below is the docs I want to insert and the function I use like the MongoDB's
// documentation.
const docs = [
{ name: "Ananas", rating: 7, review: "nice fruit"},
{ name: "Prune", rating: 8, review: "Kinda good"},
{ name: "Peach", rating: 7, review: "taste great"},
{ name: "Strawberry", rating: 9, review: "great fruit"}
];
async function insertMultiDocs (client, newList) {
try {
const insertManyresult = await client.collection("fruits").insertMany(newList);
let ids = insertManyresult.insertedIds;
console.log(`${insertManyresult.insertedCount} documents were inserted.`);
for (let id of Object.values(ids)) {
console.log(`Inserted a document with id ${id}`);
}
} catch(e) {
console.log(`A MongoBulkWriteException occurred, but there are successfully processed documents.`);
let ids = e.result.result.insertedIds;
for (let id of Object.values(ids)) {
console.log(`Processed a document with id ${id._id}`);
}
console.log(`Number of documents inserted: ${e.result.result.nInserted}`);
}
}
然后我得到這個console.log:
4 documents were inserted.
Inserted a document with id 63051aeb6b883a87e46ea895
Inserted a document with id 63051aeb6b883a87e46ea896
Inserted a document with id 63051aeb6b883a87e46ea897
Inserted a document with id 63051aeb6b883a87e46ea898
Connected successfully to server
現在我想嘗試對 ZCCADCDEDB567ABAE643E15DCF0974E503Z 做同樣的事情:
const mongoose = require("mongoose");
const { Schema } = mongoose;
main().catch(err => console.log(err));
async function main() {
try {
await mongoose.connect("mongodb://localhost:27017/fruitsDB");
const fruitSchema = new Schema({
name : String,
rating : Number,
review : String
});
const Fruit = mongoose.model("Fruit", fruitSchema);
// I insert the docs here...
Fruit.insertMany(docs)
} catch (error) {
console.log(error);
}
}
它可以工作,但是有沒有辦法在 Mongoose 中實現 console.log 並像在 MongoDB 驅動器上那樣循環插入每個文檔?
insertMany
返回在驗證后添加到數據庫的文檔(如果設置了任何驗證)。
你可以在它上面加上.length
來得到這樣的值
const insertManyresult = await Fruit.insertMany(docs);
console.log(`${insertManyresult.length} documents were inserted.`);
因為您可以訪問所有文件。 您可以執行任何選擇的操作(循環遍歷每個文檔、獲取長度等)
在此處閱讀有關insertMany
的更多信息。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.