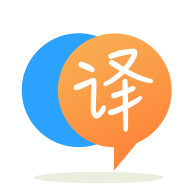
[英]Wondering how I can use a variable that is defined inside a def outside of the def and in the rest of my code
[英]how can I use a global variable in the def?
像這樣; 錯誤是num
未定義
我想使用回溯,但如何在函數中使用全局變量?
class Solution:
def removeNthFromEnd(self, head: Optional[ListNode], n: int) -> Optional[ListNode]:
num = n
def huisu(head):
if head == None:
return
else:
before = head
head = head.next
huisu(head)
if num == 0:
head.next = head.next.next
else:
num = num - 1
head = before
return head
huisu(head)
num
超出 scope。 您必須在您的內部 function 之前定義它:
class Solution:
def removeNthFromEnd(self, head: Optional[ListNode], n: int) -> Optional[ListNode]:
num = 0 # or some other default value
def huisu(head):
if head == None:
return
else:
before = head
head = head.next
huisu(head)
if num == 0:
head.next = head.next.next
else:
num = num - 1
head = before
return head
num = n
huisu(head)
您正在嘗試評估之前未聲明的內容...如果您甚至沒有聲明,我怎么知道 num 是否等於 0?
可能你錯過了 function 的一部分? 順便說一句,最好的解決方案是在 def 語句之外聲明它,這樣變量就可以在整個 function 中可見。
還記得給它分配正確的值,實際上從未使用過 num
class Solution:
def removeNthFromEnd(self, head: Optional[ListNode], n: int) -> Optional[ListNode]:
def huisu(head):
if head == None:
return
else:
before = head
head = head.next
huisu(head)
if num == 0: # here
head.next = head.next.next
else:
num = num - 1
head = before
return head
num = n
huisu(head)
你的意思:
class Solution:
def removeNthFromEnd(self, head: Optional[ListNode], n: int) -> Optional[ListNode]:
num = n # Added
def huisu(head):
if head == None:
return
else:
before = head
head = head.next
huisu(head)
if num == 0: # here
head.next = head.next.next
else:
num = num - 1
head = before
return head
num = n
huisu(head)
Python 具有三種類型的變量作用域:
scope 取決於變量的分配位置,而不是使用(讀取)的位置。
Function huisu
的賦值為 num,因此該變量被認為是 function huisu 的局部變量,即:
num = num - 1
由於之前沒有給它賦值,所以會發生錯誤。
兩種解決方案
解決方案 1
將 num 聲明為非本地
class Solution:
def removeNthFromEnd(self, head: Optional[ListNode], n: int) -> Optional[ListNode]:
num = n # Could assign num here
def huisu(head):
nonlocal num # this declaration uses num in outer function
if head == None:
return
else:
before = head
head = head.next
huisu(head)
if num == 0:
head.next = head.next.next
else:
num = num - 1
head = before
return head
num = n # Or here, doesn't matter
# What matters is assigned before function call
huisu(head)
解決方案 2
將 num 作為參數傳遞(更典型的解決方案)
class Solution:
def removeNthFromEnd(self, head: Optional[ListNode], n: int) -> Optional[ListNode]:
def huisu(head, num): # pass num as 2nd argument
if head == None:
return
else:
before = head
head = head.next
huisu(head)
if num == 0:
head.next = head.next.next
else:
num = num - 1
head = before
return head
huisu(head, n)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.