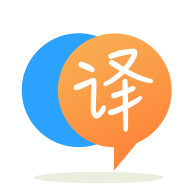
[英]error in Flutter : Unhandled Exception: type 'Null' is not a subtype of type 'String'
[英]Error:flutter Unhandled Exception: type 'Null' is not a subtype of type 'String'
我很久以前就遇到了這個問題試圖解決這個問題我在 inte.net 看到了很多地方但是無法解決這個問題從 API 從 controller class 調用中獲取信息。由於這種錯誤我看到了文檔 flutter 可能是因為 flutter package 這也是一個 dart package 我得到了 SDK 3.0.0 這里的代碼請有人幫助我實現這個項目非常感謝:
這是 model class:
class Product {
int? _totalSize;
int? _typeId;
int? _offset;
late List<ProductModel> _products;
List<ProductModel> get products => _products;
Product({required totalSize, required typeId, required offset, required products}){
this._totalSize = totalSize;
this._typeId = typeId;
this._offset = offset;
this._products = products;
}
factory Product.fromJson(Map<String, dynamic> json) {
var list = json['products'] as List;
List<ProductModel> productsList = list.map((e) => ProductModel.fromJson(e as Map<String, dynamic>)).toList();
return Product(
totalSize : json['total_size'] as int,
typeId : json['type_id'] as int,
offset : json['offset'] as int,
products: productsList
);
}
}
class ProductModel {
int? id;
String? name;
String? description;
int? price;
int? stars;
String? img;
String? location;
String? createdAt;
String? updatedAt;
int? typeId;
ProductModel(
{required this.id,
required this.name,
required this.description,
required this.price,
required this.stars,
required this.img,
required this.location,
required this.createdAt,
required this.updatedAt,
required this.typeId});
factory ProductModel.fromJson(Map<String, dynamic> json) {
return ProductModel(
id: json['id'] as int,
name: json['name'] as String,
description: json['description'] as String,
price: json['price'] as int,
stars: json['stars'] as int,
img: json['img'] as String,
location: json['location'] as String,
createdAt: json['createdAt'] as String,
updatedAt: json['updatedAt'] as String,
typeId: json['typeId'] as int
);
}
}
這是 controller class:
import 'dart:convert';
import 'package:get/get.dart';
import 'package:licores_app/data/repository/category_product_repo.dart';
import '../models/products_model.dart';
class CategoryProductController extends GetxController {
final CategoryProductRepo categoryProductRepo;
CategoryProductController({required this.categoryProductRepo});
List<dynamic> _categoryProductList = [];
// Get Method from Product Model
List<dynamic> get CategoryProductList => _categoryProductList;
// Response from repo product method
Future<void> getCategoryProductList() async {
Response response = await categoryProductRepo.getCategoryProductList();
print('got the products'); // This is right to this point
if (response.statusCode == 200) {
// Init to null not to repeat
_categoryProductList = [];
_categoryProductList.addAll(Product
.fromJson(jsonDecode(response.body))
.products); // --> Here the
// Unhandled Exception: type 'Null' is not a subtype of type 'String'
print(_categoryProductList);
update();
} else { // I added the curly braces but took off because I was
// debugging the problem still persist!
print('not able to get product list json');
}
}
}
這是錯誤:
[ERROR:flutter/lib/ui/ui_dart_state.cc(198)] Unhandled Exception: type 'Null' is not a subtype of type 'String'
E/flutter ( 6243): #0 CategoryProductController.getCategoryProductList (package:licores_app/controllers/category_product_controller.dart:27:72)
E/flutter ( 6243): <asynchronous suspension>
您正在接受 null 數據,因此您不需要強制使用as
。
你可以像這樣格式化
name: json['name']
factory ProductModel.fromJson(Map<String, dynamic> json) {
return ProductModel(
id: int.tryParse("${json['id']}"),
name: json['name'],
description: json['description'],
price: int.tryParse("${json['price']}"),
stars: json['stars'],
img: json['img'],
location: json['location'],
createdAt: json['createdAt'],
updatedAt: json['updatedAt'],
typeId: int.tryParse("${json['typeId']}"));
}
嘗試這個:
factory ProductModel.fromJson(Map<String, dynamic> json) {
return ProductModel(
id: json['id'] as int,
name: json['name'] as String ?? '',
description: json['description'] as String ?? '',
price: json['price'] as int ?? '',
stars: json['stars'] as int ?? '',
img: json['img'] as String ?? '',
location: json['location'] as String ?? '',
createdAt: json['createdAt'] as String ?? '',
updatedAt: json['updatedAt'] as String ?? '',
typeId: json['typeId'] as int ?? 0
);
}
好的,將其添加到您的代碼中:
if (response.statusCode == 200) {
// Init to null not to repeat
_categoryProductList = [];
//Added lines:
//----------------------------
print('response.body is ${response.body}');
print('of type: ${response.body.runtimeType}');
print('jsonDecode(response.body) is ${jsonDecode(response.body)}');
print('of type: ${jsonDecode(response.body).runtimeType}');
print('Product.fromJson(jsonDecode(response.body)) is ${Product.fromJson(jsonDecode(response.body))}');
print('of type: ${Product.fromJson(jsonDecode(response.body)).runtimeType}');
print('Product.fromJson(jsonDecode(response.body).products is ${Product.fromJson(jsonDecode(response.body)).products}');
print('of type: ${Product.fromJson(jsonDecode(response.body)).products.runtimeType}');
//----------------------------
_categoryProductList.addAll(Product
.fromJson(jsonDecode(response.body))
.products); // --> Here the
// Unhandled Exception: type 'Null' is not a subtype of type 'String'
print(_categoryProductList);
update();
} else { // I added the curly braces but took off because I was
// debugging the problem still persist!
print('not able to get product list json');
}
告訴我它打印了什么!
First you make all fields in ProductModel class is optional (null acceptable).
then in constructor you have remove required work because you make it as option field, and in factory constructor there is no required an additional type casting like as Int, as String, just remove it.
After this you must varified all key in factory return class ( constructor of production class ). Simply i mean check your all key of json and verify further your all data type with json you received from Api or somewhere else.
Hope this will helpfull.
您需要打印出 response.body 並將其添加到此處,然后很明顯哪個字段需要是可選的。
像這樣的聲明:
img: json['img'] as String,
當 json object 沒有定義鍵 'img' 時,將產生錯誤消息 type 'Null' is not a subtype of type 'String'
所以你正在解析的鍵之一是未定義的。
為了弄清楚是哪一個,我們需要知道響應到底是什么樣的。
然后從服務器中刪除對可選字段的所有“as String”強制轉換。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.