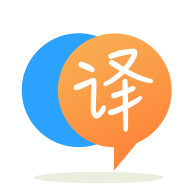
[英]Get specific value from array of objects [{“key”:“value”}, {“key”:“value”}]
[英]How to get an object from an array of objects with a specific value for a key?
例如,我們有以下對象數組
[
{ x_id: 3, name: abc, class: 3rd, subject: maths},
{ x_id: 33, name: sad, class: 4th, subject: maths},
{ x_id: 12, name: fds, class: 3rd, subject: phy},
{ x_id: 4, name: atgr, class: 10th, subject: sst},
]
x_id 4 的 Output
{ x_id: 4, name: atgr, class: 10th, subject: sst}
您可以使用 .find() 執行此操作,例如這是您的數組
const array = [
{ x_id: 3, name: 'abc', class: '3rd', subject: 'maths'},
{ x_id: 33, name: 'sad', class: '4th', subject: 'maths'},
{ x_id: 12, name: 'fds', class: '3rd', subject: 'phy'},
{ x_id: 4, name: 'atgr', class: '10th', subject: 'sst'},
];
使用此方法,您將迭代並在數組中找到您要查找的元素
var result = array.find(x => x.x_id === 4);
console.log(result); //returns { x_id: 4, name: 'atgr', class: '10th', subject: 'sst'}
var array1 = [
{ x_id: 3, name: "abc", class: "3rd", subject: "maths" },
{ x_id: 33, name: "sad", class: "4th", subject: "maths" },
{ x_id: 12, name: "fds", class: "3rd", subject: "phy" },
{ x_id: 4, name: "atgr", class: "10th", subject: "sst" },
{ x_id: 4, name: "atgr", class: "12th", subject: "phy" }
]
// returns the first element, that satisfies the provided testing function.
let element = array1.find(element => element.x_id === 4);
console.log(element);
// returns the all elements, that satisfies the provided testing function.
let results = array1.filter(element => element.x_id === 4);
console.log(results);
使用find()搜索滿足提供的 function ( element => element.x_id === 4 ) 的第一個元素
let element = array1.find(element => element.x_id === 4);
console.log(element);
返回
{ x_id: 4, name: 'atgr', class: '10th', subject: 'sst' }
使用filter()搜索滿足提供的 function ( element => element.x_id === 4 ) 的所有對象
let results = array1.filter(element => element.x_id === 4);
console.log(results);
返回
[
{ x_id: 4, name: 'atgr', class: '10th', subject: 'sst' },
{ x_id: 4, name: 'atgr', class: '12th', subject: 'phy' }
]
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.