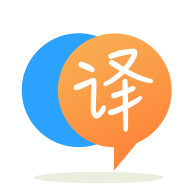
[英]How to exit a function with a return value without using "return" in c++
[英]how to return a value without exiting a function and storing it subsequently, in c++
在這里,我嘗試使用隨機數模擬來自證券交易所的數據饋送流,然后將其存儲在數組中。
#include <iostream>
#include <array>
#include <string.h>
#include <stdlib.h>
#include <stdio.h>
#include <math.h>
#include <vector>
#include <fstream>
#include <cstdlib>
#include <chrono>
#include <ctime>
#include <stdlib.h>
long int prevcntr=0;
using namespace std;
std::pair<long int, double>feedgenerator(long int len)
{
srand(time(0));
for(long int itr=1;itr<=len;itr++)
{
return {itr, (double)rand()/RAND_MAX};
//should continue evaluating the function without exiting
pause(0.001); //To allow some interval for 'makearray' to store it
}
}
template<size_t nn>
std::array<double, nn> makearray(long int cntr, double value, long int len)
{
std::array<double, nn> outarr; // should be able to pass the value of 'len' to 'nn'
long int itr=0;
begin:
while(cntr <= prevcntr)goto begin; // should wait until the next update
outarr[itr] = value;
prevcntr = cntr;
while(itr<len)
{
itr++;
goto begin; // control goes back to the beginning until all the elements of the array are filled with value
}
//should return the array after it is fully filled
return outarr;
}
int main()
{
double *p=new double[];
long int len = 100000;
*p = makearray(feedgenerator(len), len)
// I should be able to call these as nested functions as above
for(int i=0;i<len;i++)
cout<<*p[i]<<"\n";
return 0;
}
問題是如何在不退出feedgenerator
function 的情況下返回一個值。 如果我嘗試一次獲取所有值,那么它就不會模仿數據饋送。 數據饋送本質上是按順序更新相同的值。
為了存儲數據,正在使用makearray
(不應該使用vector ,因為它非常慢)。
總的來說, feedgenerator
應該使用遞增的計數器更新相同的值(在實際場景中,計數器將是時間,值將是價格等)並且makearray
應該存儲數據(除非我存儲數據,否則數據將是丟失,如來自證券交易所的數據饋送)以供后續分析。
在makearray
中,我想將數組的長度作為 function 的參數傳遞,以便它可以由另一個程序控制。 我不清楚如何做到這一點。
當前形式的代碼無法編譯。
問題是如何在不退出 feedgenerator function 的情況下返回一個值
因為您試圖模仿來自證券交易所的數據饋送 stream,所以我認為您應該考慮使用線程。 例子:
class FeedGenerator {
long int max_length;
std::deque<double> queue;
std::mutex mutex;
std::thread thread;
public:
FeedGenerator(long int len) : max_length(len) {
thread(&FeedGenerator::run, this);
}
// Get the latest `len` values
int getData(std::vector<double>& vec, int len) {
std::lock_guard<std::mutex>(mutex);
int nlen = std::min(len, queue.size());
vec.resize(nlen);
std::copy(queue.end() - nlen, queue.end(), vec.begin());
return nlen;
}
private:
void run() {
srand(time(0));
while (TRUE)
{
{
std::lock_guard<std::mutex>(mutex);
queue.push_back((double)rand()/RAND_MAX);
if (queue.size() >= len) {
queue.pop_front();
}
}
pause(0.001);
}
}
};
int main()
{
long int len = 100000;
FeedGenerator feedgenerator(len);
std::vector<double> p;
feedgenerator.getData(p, 10); // get the latest 10 values
for(int i=0;i<p.size();i++)
std::cout << p[i] << std::endl;
return 0;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.