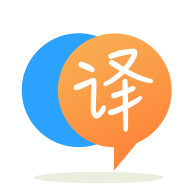
[英]How do I keep the active tab after page refresh with localStorage?
[英]How to keep active tab after page refresh with sessionStorage
我在 html / js 中有幾個選項卡,在每個選項卡中我都有一個帶有保存更改按鈕的表單,當我單擊保存更改按鈕時,頁面被刷新。 刷新頁面后,選項卡返回到第一項,而最后一次應該保持不變。
有沒有辦法做到這一點?
我在考慮localstorage,但我不知道該怎么做,有人能指出我正確的方法嗎?
function openCity(evt, cityName) { var i, tabcontent, tablinks; tabcontent = document.getElementsByClassName("tabcontent"); for (i = 0; i < tabcontent.length; i++) { tabcontent[i].style.display = "none"; } tablinks = document.getElementsByClassName("tablinks"); for (i = 0; i < tablinks.length; i++) { tablinks[i].className = tablinks[i].className.replace(" active", ""); } document.getElementById(cityName).style.display = "block"; evt.currentTarget.className += " active"; } // Get the element with id="defaultOpen" and click on it document.getElementById("defaultOpen").click();
/* Style the tab */.tab { float: left; border: 1px solid #ccc; background-color: #f1f1f1; width: 30%; height: 300px; } /* Style the buttons inside the tab */.tab button { display: block; background-color: inherit; color: black; padding: 22px 16px; width: 100%; border: none; outline: none; text-align: left; cursor: pointer; transition: 0.3s; font-size: 17px; } /* Change background color of buttons on hover */.tab button:hover { background-color: #ddd; } /* Create an active/current "tab button" class */.tab button.active { background-color: #ccc; } /* Style the tab content */.tabcontent { float: left; padding: 0px 12px; border: 1px solid #ccc; width: 70%; border-left: none; height: 300px; }
<div class="tab"> <button class="tablinks" onclick="openCity(event, 'London')" id="defaultOpen">London</button> <button class="tablinks" onclick="openCity(event, 'Paris')">Paris</button> <button class="tablinks" onclick="openCity(event, 'Tokyo')">Tokyo</button> </div> <div id="London" class="tabcontent"> <h3>London</h3> <p>London is the capital city of England.</p> </div> <div id="Paris" class="tabcontent"> <h3>Paris</h3> <p>Paris is the capital of France.</p> </div> <div id="Tokyo" class="tabcontent"> <h3>Tokyo</h3> <p>Tokyo is the capital of Japan.</p> </div>
觀察:根據您當前的代碼,看起來您在每次頁面加載時都在包含 id defaultOpen
的元素上觸發click()
事件。
解決方案:這個邏輯需要改變。 我們可以通過使用Web storage API
來實現此要求,方法是檢查本地存儲中是否有可用的currentTab
變量,如果可用,則加載該選項卡,否則運行此默認單擊事件邏輯。
現在的步驟是:
在 openCity() 方法中設置 localStorage 值。
localStorage.setItem('currentTab', cityName);
檢查本地存儲中是否存在任何currentTab
變量。
const currentTab = localStorage.getItem('currentTab');
現在添加以下條件。
if (currentTab) { document.getElementById(currentTab).click(); } else { document.getElementByName("defaultOpen").click(); }
現場演示(由於安全原因,window.localStorage 在下面的代碼片段中不起作用,但在本地或在 jsfiddle 中可以正常工作) :
function openCity(evt, cityName) { var i, tabcontent, tablinks; localStorage.setItem('currentTab', cityName); tabcontent = document.getElementsByClassName("tabcontent"); for (i = 0; i < tabcontent.length; i++) { tabcontent[i].style.display = "none"; } tablinks = document.getElementsByClassName("tablinks"); for (i = 0; i < tablinks.length; i++) { tablinks[i].className = tablinks[i].className.replace(" active", ""); } document.getElementsByClassName(cityName)[0].style.display = "block"; evt.currentTarget.className += " active"; } const currentTab = localStorage.getItem('currentTab'); if (currentTab) { document.getElementById(currentTab).click(); } else { document.getElementsByClassName("tablinks")[0].click(); }
/* Style the tab */.tab { float: left; border: 1px solid #ccc; background-color: #f1f1f1; width: 30%; height: 300px; } /* Style the buttons inside the tab */.tab button { display: block; background-color: inherit; color: black; padding: 22px 16px; width: 100%; border: none; outline: none; text-align: left; cursor: pointer; transition: 0.3s; font-size: 17px; } /* Change background color of buttons on hover */.tab button:hover { background-color: #ddd; } /* Create an active/current "tab button" class */.tab button.active { background-color: #ccc; } /* Style the tab content */.tabcontent { float: left; padding: 0px 12px; border: 1px solid #ccc; width: 70%; border-left: none; height: 300px; }
<div class="tab"> <button class="tablinks" id="London" onclick="openCity(event, 'London')">London</button> <button class="tablinks" id="Paris" onclick="openCity(event, 'Paris')">Paris</button> <button class="tablinks" id="Tokyo" onclick="openCity(event, 'Tokyo')">Tokyo</button> </div> <div class="tabcontent London"> <h3>London</h3> <p>London is the capital city of England.</p> </div> <div class="tabcontent Paris"> <h3>Paris</h3> <p>Paris is the capital of France.</p> </div> <div class="tabcontent Tokyo"> <h3>Tokyo</h3> <p>Tokyo is the capital of Japan.</p> </div>
你可以添加類似的東西
localStorage.setItem('currentCity', cityName);
到您的openCity()
function,它將當前標簽 ID 寫入 localStorage。 然后,在頁面加載時,從 localStorage 中讀取:
const defaultOpen = localStorage.getItem('currentCity') || 'London';
或者,您可以查看使用 URL 進行 state 管理,例如將currentCity
附加到 URL 並在加載時讀取它。
如果你真的想使用 localStorage (這可能不是最好的方法,正如所指出的,使用一些 hash 參數看起來“更好”),這是一個修改最少的提案,可以實現你想要的
1-調用openCity時將最后選擇的城市存儲在本地存儲中
openCity() {
...
window.localStorage.setItem('SAMPLE_SITE_CITY', cityName)
}
2-添加一些腳本以在頁面打開時執行:從本地存儲中讀取值; 找到相應的 DOM 元素並在其上觸發選擇(並處理沒有存儲任何內容的情況,只需像以前一樣選擇默認 select):
const previousCity = window.localStorage.getItem('SAMPLE_SITE_CITY');
if (previousCity) {
const xpath = `//button[text()='${previousCity}']`;
const elem = document.evaluate(xpath, document, null, XPathResult.FIRST_ORDERED_NODE_TYPE, null).singleNodeValue;
elem.click();
} else {
document.getElementById("defaultOpen").click();
}
注意:為您的按鈕添加一個“id”並將 id 存儲在本地存儲中將簡化最后一個選定元素的檢索。
您可以在此代碼筆上找到工作示例: https://codepen.io/aSH-uncover/pen/qBYaZOB
為此,您將需要使用本地存儲。
localStorage.setItem('currentCity', cityName);
頁面加載時,從本地存儲中獲取 cityName
const defaultOpen = localStorage.getItem('currentCity');
希望能幫助到你!
1 那個時候調用open city方法的時候我們需要把最后選擇的城市存入本地存儲
window.localStorage.setItem('CurrnetCityName', cityName);
2 頁面加載時從本地商店獲取當前城市名稱並添加
const defaultOpen = localStorage.getItem('CurrnetCityName');
希望對您有所幫助!
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.