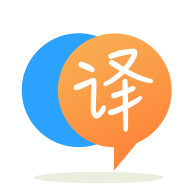
[英]How do I display text in a .txt file with an async function with jsonp?
[英]How to display text from async function
我想在個人資料頁面上顯示從異步 function 獲取的用戶名。 這是我在 user.js 上獲取用戶名的代碼。
//assume username is Peter
async function user_attr() {
try{
const { attributes } = await Auth.currentAuthenticatedUser();
console.log(JSON.stringify(attributes.username)); //output:Peter
} catch (e) {
console.log(e);
}
}
我想在 profile.js 上的文本中顯示它。
import './user.js'
<Text>{username}</Text>
由於 async function 無法返回值,我該怎么做才能顯示用戶名?
您可以使用.then.catch
方法。
在您的 function 中,您返回所需的值(在本例中為用戶名):
async function user_attr() {
try{
const { attributes } = await Auth.currentAuthenticatedUser();
const username = JSON.stringify(attributes.username);
return username;
} catch (e) {
console.log(e);
}
}
export default user_attr;
然后在要使用用戶名的文件中:
import user_attr from './user.js';
let username;
user_attr().then((res) => {
username = res;
console.log(username); //output:Peter
)};
為了能夠在 React 組件上顯示返回的用戶名,您必須在useState()
掛鈎中設置值。 為此,可以在useEffect()
掛鈎中調用 API。
const [userName, setUserName] = useState();
useEffect(() => {
Auth
.currentAuthenticatedUser()
.then((data) => {
setUserName(JSON.stringify(data.attributes.username));
})
.catch((err) => console.error(err));
}, []);
return (
...
<Text>{userName}</Text>
...
);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.