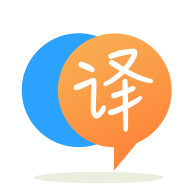
[英]How can I get the user input from a HTML form and store it in an existing JavaScript array
[英]How can I get input(they will input a link) from user and store it inside of a button and have it redirect?
import { useState } from 'react';
const AddNote = ({ handleAddNote }) => {
const [noteText, setNoteText] = useState('');
const [jobLink, setJobLink] = useState('');
const characterLimit = 200;
const handleDescChange = (event) => {
if (characterLimit - event.target.value.length >= 0) {
setNoteText(event.target.value);
}
};
const handleJobLinkChange = (event) => {
if (event.target.value.length >= 0) {
setJobLink(event.target.value);
console.log(event.target.value);
}
};
const handleSaveClick = () => {
if (noteText.trim().length > 0) {
handleAddNote(noteText);
setNoteText('');
setJobLink('');
}
};
return (
<div className='note new'>
<textarea
rows='8'
cols='10'
placeholder='Type to add a note...'
value={noteText}
onChange={handleDescChange}
></textarea>
<input style={{
paddingTop: "1.5%",
outline: "none",
color: "black",
marginRight: "0px",
marginLeft: "0px",
paddingRight: "0px",
backgroundColor: "white"
}}
className="form-control"
id="link"
name="link"
placeholder="Link to Job Posting"
value={jobLink}
type="link"
onChange={handleJobLinkChange}
/>
<div className='note-footer'>
<small>
{characterLimit - noteText.length} Remaining
</small>
<button className='save' onClick={handleSaveClick}>
Save
</button>
</div>
</div>
);
};
export default AddNote;
import { MdDeleteForever } from 'react-icons/md';
import AddNote from './AddNote';
const Note = ({ id, link, text, date, handleDeleteNote,}) => {
const go = e => {
link = "https://www.google.com";
e.preventDefault();
window.location.href = link;
}
return (
<div className='note'>
<span>{text}</span>
<div className='note-footer'>
<small>{date}</small>
<div className='note-btn'>
<button onClick={go} style={{ backgroundColor: "#001E49", borderRadius: "10px"
, borderColor: "none", margin: "auto", padding: "8px", marginLeft: "0px"
, marginRight: "0px", width: "fit-content"
, borderColor: "none"}}>Apply Here</button>
</div>
</div>
</div>
);
};
export default Note;
import Note from './Note';
import AddNote from './AddNote';
const NotesList = ({
notes,
handleAddNote,
handleDeleteNote,
}) => {
return (
<div className='notes-list'>
{notes.map((note) => (
<Note
id={note.id}
text={note.text}
date={note.date}
handleDeleteNote={handleDeleteNote}
/>
))}
<AddNote handleAddNote={handleAddNote} />
</div>
);
};
export default NotesList;
import React from 'react'
import './Internships.css'
import { useState, useEffect } from 'react';
import { nanoid } from 'nanoid';
import NotesList from '../components/NotesList';
import Search from '../components/Search';
import Header from '../components/Header';
const Internships = () => {
const [notes, setNotes] = useState([
]);
const [searchText, setSearchText] = useState('');
const [darkMode, setDarkMode] = useState(false);
useEffect(() => {
const savedNotes = JSON.parse(
localStorage.getItem('react-notes-app-data')
);
if (savedNotes) {
setNotes(savedNotes);
}
}, []);
useEffect(() => {
localStorage.setItem(
'react-notes-app-data',
JSON.stringify(notes)
);
}, [notes]);
const addNote = (text, link) => {
const date = new Date();
const newNote = {
id: nanoid(),
text: text,
date: date.toLocaleDateString(),
link: link,
};
const newNotes = [...notes, newNote];
setNotes(newNotes);
};
const deleteNote = (id) => {
const newNotes = notes.filter((note) => note.id !== id);
setNotes(newNotes);
};
return (
<div className={`${darkMode && 'dark-mode'}`}>
<div className='container'>
<Header />
<Search handleSearchNote={setSearchText} />
<NotesList
notes={notes.filter((note) =>
note.text.toLowerCase().includes(searchText)
)}
handleAddNote={addNote}
handleDeleteNote={deleteNote}
/>
</div>
</div>
);
};
export default Internships
對於 'link="www.google.com"'(它是一個占位符),該按鈕將我重定向到 google.com 但我希望有一個用戶輸入的網站並將我重定向到那里。 我無法將 joblink 傳遞到 note.js 並且當我檢查創建的按鈕上的元素時它沒有讀取它。 我不知道如何解決嘗試從用戶獲取輸入並將其傳遞給 note.js 的問題。
我對論壇上的代碼是如何寫的有點困惑,但似乎您可以在輸入中為目標值設置 state。 所以做類似的事情
const[site, setSite] = useState("https://www.google.com");
<input style={{
paddingTop: "1.5%",
outline: "none",
color: "black",
marginRight: "0px",
marginLeft: "0px",
paddingRight: "0px",
backgroundColor: "white"
}}
className="form-control"
id="link"
name="link"
placeholder="Link to Job Posting"
value={jobLink}
type="link"
onChange={() => setSite(e.target.value)}
/>
然后使用回調道具並將其發送到您的 Note 組件,然后將您的“go”鏈接替換為
link = site
如果這沒有意義,我很抱歉,哈哈。
據我了解,您擁有 AddNote 組件,用戶可以在其中生成新的職位發布,而此處保存的這些值在 Note 組件中是必需的
這里不僅狀態保存在不正確的組件中,而且當它們點擊保存(handleSaveClick)時,會發生這種情況:
setNoteText('');
setJobLink('');
我建議有一個 Notes 組件,包括一個 notes state 數組,您將在其中保存一個帶有 noteText 和 jobLink 的 json,然后是 map 將它們作為 props 組件傳遞。 這個 Notes 組件還可以包含 AddNote 組件,這樣您就可以通過 setNotes 鈎子並有效地處理動態職位發布:
const [notes, setNotes] = useState([])
{notes?.length > 0 && notes.map(elem => (<Note noteText={elem.noteText} jobLink={elem.jobLink} />))}
(最好將 'elem' 作為一個整體傳遞,也許你會向它添加新屬性並且道具數量會增加)並使用 useRef 鈎子:
const handleSaveClick = () => {
handleAddNote(prev => [...prev, {noteLink: noteLinkRef.current.value, jobLink: jobLinkRef.current.value}])
}
希望這有助於理解,如果您覺得我需要再擴展一點,請告訴我!
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.