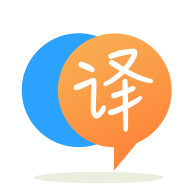
[英]How can I set a size for a Tkinter window using ```import Tkinter``` instead of ```from tkinter import *```?
[英]How can I "reset" the size of a Window on Tkinter when using Notebook?
我知道以前有人問過這個問題,但到目前為止我所看到的解決方案似乎都沒有奏效。
所以這是確切的情況:我正在構建一個具有 3 個不同選項卡的應用程序(使用 ttk.Notebook)。 3 個選項卡的初始內容不同:第二個是最小的,第三個是最大的。
現在問題來了(我正在使用網格,順便說一句):當我從第一個更改為第二個時,有一個額外的空白空間(因為第一個選項卡的內容比第二個選項卡的內容大一點,當我從第三個到第二個/第一個 go 時也會發生同樣的情況,因此在使用第三個選項卡並更改為另一個選項卡后,尺寸保持不變,就好像我仍然在第三個一樣。
我添加了一些我正在使用的代碼,但由於我不確定出了什么問題,所以我會讓您詢問您可能需要的任何額外代碼來幫助我找到解決方案。
# Tk modules
import tkinter as tk
from tkinter import ttk
# # define main()
def main():
app = App()
MainWindow(app)
app.mainloop()
# Frame for data input
class RegisterChooseType(ttk.Frame):
def __init__(self, container):
# Inheritance
super().__init__(container)
self.container = container
# Var for selection
self.selected_value = tk.IntVar()
# Options
self.options = {"padx": 10, "pady": 10}
# Doc types
self.doc_types = ("Incoming Bill", "Outgoing Bill", "Contract", "Other")
# Labels
self.barcode_label = ttk.Label(self, text="Barcode:").grid(
row=0, column=0, sticky="EW", **self.options
)
self.doc_type_label = ttk.Label(self, text="Document type:").grid(
row=0, column=2, sticky=tk.W, **self.options
)
# Entries
self.barcode_entry = ttk.Entry(self)
self.barcode_entry.grid(row=0, column=1, sticky="EW", **self.options)
self.grid()
def submit(self):
self.current_dict["func"](self, self.current_frame)
class DeleteTab(ttk.Frame):
def __init__(self, container):
# Inheritance
super().__init__(container)
# self.columnconfigure(0, weight=1)
# self.columnconfigure(1, weight=1)
self.options = {"padx": 10, "pady": 10}
## Type Barcode
# Label
self.barcode_label = ttk.Label(self, text="Barcode:").grid(
row=0, column=0, sticky="EW", **self.options
)
# Entries
self.barcode_entry = ttk.Entry(self)
self.barcode_entry.grid(row=0, column=1, sticky="EW", **self.options)
# self.pack(fill="x")
self.grid(sticky="W")
class ViewTab(ttk.Frame):
def __init__(self, container):
# Inheritance
super().__init__(container)
self.container = container
self.options = {"padx": 10, "pady": 10}
# Doc types
self.doc_types = ("Incoming Bill", "Outgoing Bill", "Contract", "Other")
## Type Barcode
# Labels
# Barcode
self.barcode_label = ttk.Label(self, text="Barcode:")
self.barcode_label.grid(row=0, column=0, sticky="EW", **self.options)
# Document type
self.doc_type_label = ttk.Label(self, text="Document Type:")
self.doc_type_label.grid(row=0, column=2, sticky="EW", **self.options)
# Entries
# Barcode
self.barcode_entry = ttk.Entry(self)
self.barcode_entry.grid(row=0, column=1, sticky="EW", **self.options)
# Document type
self.doc_type_comb = ttk.Combobox(self)
self.doc_type_comb["state"] = "readonly" # Doesn't allow new entries
self.doc_type_comb["values"] = self.doc_types
self.doc_type_comb.grid(
row=0, column=3, columnspan=3, sticky=tk.EW, **self.options
)
## View Button
self.viewSubmitButton = ttk.Button(
self, text="View", command=lambda: print("View")
)
self.viewSubmitButton.grid(
column=4,
row=self.grid_size()[0],
sticky="EW",
**self.options,
)
# New LabelFrame
self.deliver_view_LabelFrame = ttk.LabelFrame(self)
self.deliver_view_LabelFrame["text"] = "View Document"
self.deliver_view_LabelFrame.grid(
row=1,
columnspan=self.grid_size()[0],
column=0,
sticky="ew",
padx=10,
pady=10,
)
# Inside LabelFrame
##TreeView
self.dataDisplay = ttk.Treeview(self.deliver_view_LabelFrame, show="")
self.dataDisplay.pack(fill="x", **self.options)
self.grid(sticky="ews")
# Create window
class MainWindow:
def __init__(self, container):
self.container = container
## Create Parent
self.tab_parent = ttk.Notebook()
self.tab_parent.enable_traversal()
# Create tab frames
self.tab_register = ttk.Frame(self.tab_parent)
self.tab_view = ttk.Frame(self.tab_parent)
self.tab_delete = ttk.Frame(self.tab_parent)
# Adding the tabs to the main object (self.tab_parent)
self.tab_parent.add(self.tab_register, text="Register Documents")
self.tab_parent.add(self.tab_delete, text="Delete Documents")
self.tab_parent.add(self.tab_view, text="View Documents")
# Create empt variables
self.current_tab = None
self.last_tab = None
# Focus on barcode
# self.register.barcode_entry.focus()
self.tab_parent.bind("<<NotebookTabChanged>>", self.on_tab_change)
# Pack notebook
# self.tab_parent.pack(expand=True, fill="x", side="left")
self.tab_parent.grid(sticky="e")
## Triggers when changing tabs
def on_tab_change(self, event):
if self.current_tab != None:
self.current_tab.destroy()
# Get the current tab name
selected = event.widget.tab("current")["text"]
# Create frame depending on Tab chosen
if selected == "Register Documents":
self.current_tab = RegisterChooseType(self.tab_register)
self.clean_tabs()
self.last_tab = self.current_tab
elif selected == "Delete Documents":
self.current_tab = DeleteTab(self.tab_delete)
self.clean_tabs()
self.last_tab = self.current_tab
elif selected == "View Documents":
self.current_tab = ViewTab(self.tab_view)
self.clean_tabs()
self.last_tab = self.current_tab
self.current_tab.barcode_entry.focus()
def clean_tabs(self):
if self.last_tab != None:
# for widget in self.last_tab.winfo_children():
# # for widget in self.last_tab.grid_slaves():
# widget.destroy()
self.last_tab.destroy()
# ## INTERESTING
# self.last_tab.blank = ttk.Label(self.last_tab, text="1")
# self.last_tab.blank.grid()
# print(self.last_tab.grid_slaves()[0].cget("text"))
# self.container.geometry("1x1")
self.tab_parent.update()
# self.container.update()
# self.container.geometry("")
# self.container.update()
# Creatig App
class App(tk.Tk):
def __init__(self):
super().__init__()
self.geometry("")
self.title("iMPETU Document Handling v.0.1.0a")
self.resizable(0, 0)
if __name__ == "__main__":
main()
我嘗試在更改為另一個選項卡之前一一刪除當前選項卡的小部件,在更改為新選項卡后也執行相同操作(這就是為什么我有當前和最后一個選項卡的 2 個變量),我還嘗試留下一個空標簽(應該是 0 像素),但這會將所有內容向下推。
我出於好奇嘗試了退出,去圖標化,我還嘗試使用 self.container.update() 來更新主 window,還嘗試在 clean_tabs function 中將幾何形狀更改為“1x1”,然后在最后再次更改為“” on_tab_change ,看看這是否會迫使它調整大小,但這些都不起作用。
您可以在clean_tabs()
的開頭將所有框架( RegisterChooseType
、 DeleteTab
和ViewTab
實例的父級)的大小調整為 1x1:
def clean_tabs(self):
for tab in self.tab_parent.tabs():
# tab is the name of the frame
# so get the widget using .nametowidget()
# then call .config(width=1, height=1) to resize the frame to size 1x1
self.tab_parent.nametowidget(tab).config(width=1, height=1)
...
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.