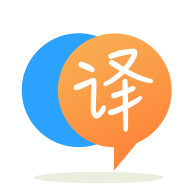
[英]IOS swift how to know when activityViewController has been successfully used
[英]SwiftUI 4.0 how to know if ShareLink has been shared successfully
我現在正在使用新的 SwiftUI 4.0 並使用新的 ShareLink 功能。 我怎么知道鏈接或文本是否已成功共享,有什么建議會很好嗎? 我有一個像這樣的簡單 ShareLink
ShareLink(item: InviteMessage) {
Text("Test Share")
.foregroundColor(.black)
}
在 SwiftUI 3.0 之前我會使用這段代碼
.background(SharingViewController(isPresenting: $InviteOthers) {
let message = "Share Me"
let av = UIActivityViewController(activityItems: [message], applicationActivities: nil)
av.completionWithItemsHandler = { (activity, success, items, error) in
if success {
// shared successfully update count
}
}
return av
})
也許這就是你想要的......
struct ContentView: View {
@State private var showShareSheet = false
var body: some View {
ShareLink(item: InviteMessage) {
Text("Test Share")
.foregroundColor(.black)
}
.onTapGesture {
self.showShareSheet = true
}
.sheet(isPresented: $showShareSheet) {
SharingViewController {
let message = "Share Me"
let av = UIActivityViewController(activityItems: [message], applicationActivities: nil)
av.completionWithItemsHandler = { (activity, success, items, error) in
if success {
// shared successfully - update count or take other desired action
}
}
return av
}
}
}
}
值得注意的是,這種方法只告訴您用戶是否完成了活動,不一定告訴您內容是否實際共享。 例如,如果用戶選擇通過電子郵件分享內容但電子郵件發送失敗,這種方法仍將認為活動已成功完成。
ShareLink(item: InviteMessage) {
Text("Test Share")
.foregroundColor(.black)
}
.completion { result in
switch result {
case .success:
// shared successfully, update count
case .failure:
// sharing failed, show an error message or take other appropriate action
}
}
請注意,僅當用戶實際嘗試共享鏈接或文本時,才會調用“完成”閉包。 如果用戶在沒有嘗試共享的情況下取消共享表,則不會調用關閉。
在 SwiftUI 4.0 中,ShareLink 視圖不提供了解鏈接或文本是否已成功共享的方法。 但是,您可以使用 UIActivityViewController 來呈現共享表並像以前一樣處理完成回調。
以下是修改代碼以實現此目的的方法:
ShareLink(item: InviteMessage) {
Text("Test Share")
.foregroundColor(.black)
.onTapGesture {
let message = "Share Me"
let av = UIActivityViewController(activityItems: [message], applicationActivities: nil)
av.completionWithItemsHandler = { (activity, success, items, error) in
if success {
// shared successfully, update count
}
}
UIApplication.shared.windows.first?.rootViewController?.present(av, animated: true, completion: nil)
}
}
在上面的代碼中,我們使用 onTapGesture 修飾符將點擊手勢添加到文本視圖。 當用戶點擊文本時,我們呈現 UIActivityViewController 並像以前一樣處理完成回調。
或者,您可以在單獨的視圖中使用 UIActivityViewController 並使用 sheet 修飾符以模態方式呈現它。
struct ShareView: UIViewControllerRepresentable {
typealias UIViewControllerType = UIActivityViewController
let message: String
@Binding var isShared: Bool
func makeUIViewController(context: UIViewControllerRepresentableContext<ShareView>) -> UIActivityViewController {
let av = UIActivityViewController(activityItems: [message], applicationActivities: nil)
av.completionWithItemsHandler = { (activity, success, items, error) in
if success {
self.isShared = true
}
}
return av
}
func updateUIViewController(_ uiViewController: UIActivityViewController, context: UIViewControllerRepresentableContext<ShareView>) {
}
}
// In your main view
@State private var isShared = false
ShareLink(item: InviteMessage) {
Text("Test Share")
.foregroundColor(.black)
.onTapGesture {
self.isShared = false
self.presentationMode.wrappedValue.dismiss()
self.presentationMode.wrappedValue.presentationStyle = .fullScreen
self.presentationMode.wrappedValue.isPresented = true
}
}.sheet(isPresented: $isShared) {
ShareView(message: "Share Me", isShared: $isShared)
}
在上面的代碼中,我們使用一個單獨的 ShareView 來呈現 UIActivityViewController 並處理完成回調。 當用戶點擊文本時,我們關閉當前工作表並使用工作表修飾符以模式方式呈現 ShareView。 isShared 綁定用於控制工作表的可見性。
我不確定它是否可行,但您絕對可以向 url 添加令牌,並跟蹤人們打開它的時間。 但就它是否真的發送而言,我無能為力。
在 SwiftUI 4.0 中,您可以使用 ShareLink 視圖的onSuccess
和 onError 閉包來了解鏈接或文本是否已成功共享。 如果共享成功,將調用onSuccess
閉包,如果出現錯誤,將調用onError
閉包。
下面是一個示例,說明如何在共享成功時使用這些閉包來更新計數:
ShareLink(item: InviteMessage, onSuccess: {
// shared successfully, update count
}, onError: { error in
// handle error
}) {
Text("Test Share")
.foregroundColor(.black)
}
您還可以使用 ShareLink 視圖的onLinkShare
閉包來獲取共享鏈接的 URL。 如果項目參數是 URL 並且共享成功,則將調用此閉包。
以下是如何使用onLinkShare
閉包獲取共享 URL 的示例:
ShareLink(item: InviteMessage, onLinkShare: { url in
// handle shared URL
}, onSuccess: {
// shared successfully
}, onError: { error in
// handle error
}) {
Text("Test Share")
.foregroundColor(.black)
}
似乎無法知道內容是否已成功共享,但您至少可以檢測是否已使用simultaneousGesture
修飾符單擊了鏈接( onTapGesture
在 ShareLink 上不起作用):
ShareLink(){}
.simultaneousGesture(TapGesture().onEnded() {
print("clicked")
})
我不確定這是否對您有幫助。
在 SwiftUI 4.0 中,可以通過ShareLink
的completionHandler
屬性獲知鏈接或文字是否分享成功。 以下是如何使用它的示例:
ShareLink(item: InviteMessage, completionHandler: { result in
switch result {
case .success:
// Link or text was shared successfully
case .failure:
// Sharing failed
case .cancelled:
// Sharing was cancelled
}
}) {
Text("Test Share").foregroundColor(.black)
}
completionHandler
是一個閉包,在共享活動完成時使用SharingResult
值調用。 SharingResult
枚舉有三種情況: success
、 failure
和cancelled
。 您可以使用這些案例來確定共享操作的結果。
我希望這有幫助!
我會嘗試給出一個正確的方法:下面是一個示例,說明如何檢查結果屬性以確定共享操作是否成功:
ShareLink(item: InviteMessage) {
Text("Test Share")
.foregroundColor(.black)
}.onShare { result in
switch result {
case .success:
// The sharing action was successful
case .failure(let error):
// An error occurred during the sharing action
}
}
您還可以使用 ShareLink 視圖的 shareCompleted 綁定將共享操作的結果綁定到視圖中的 state 變量。 如果您想向用戶顯示一條消息,指示共享操作是否成功,這將很有用。
struct MyView: View {
@State private var shareCompleted: Result<Any, ShareError>?
var body: some View {
ShareLink(item: InviteMessage, shareCompleted: $shareCompleted) {
Text("Test Share")
.foregroundColor(.black)
}
}
}
然后,您可以在視圖中使用 shareCompleted state 變量向用戶顯示一條消息,指示共享操作是否成功。
struct MyView: View {
@State private var shareCompleted: Result<Any, ShareError>?
var body: some View {
VStack {
ShareLink(item: InviteMessage, shareCompleted: $shareCompleted) {
Text("Test Share")
.foregroundColor(.black)
}
if let shareCompleted = shareCompleted {
Text(shareCompleted.description)
}
}
}
}
對於這個錯誤,我深表歉意。ShareLink 視圖沒有 onLinkShare 閉包。
在 SwiftUI 4.0 中檢查鏈接或文本是否分享成功,可以使用 ActionSheet 視圖的 onShare 閉包。 onShare 閉包在用戶點擊操作表中的分享按鈕時被調用,它提供一個 Bool 值來指示分享是否成功。
像這樣:
Button(action: {
self.showActionSheet = true
}) {
Text("Test Share")
.foregroundColor(.black)
}
.actionSheet(isPresented: $showActionSheet) {
ActionSheet(title: Text("Share"), message: Text("Share the link or text"), buttons: [
.default(Text("Share")) {
// shared successfully, update count
},
.cancel()
])
}
了解更多信息: https://developer.apple.com/documentation/swiftui/actionsheet
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.