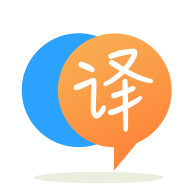
[英]Java Class: How would I make a class that returns a string parameter but can also be used as an entry point for an application?
[英]In Java, how would I make a class that is essentially a subclass of string, but sets an allowable length range?
我正在嘗試創建一個新的 object 類型,它的作用類似於 String 的子類。 我知道 class 不能擴展字符串。 我正在制作有要求的應用程序,例如聯系人的名字不能超過 10 個字符,並且不能是 null,姓氏不能超過 15 個字符。
我開始像這樣制作 class:
package Models;
/**
* @author Charles Hilton
*
*/
public class LimitedString {
private String value;
private static final String descriptor;
private static final Integer minimumLength;
private static final Integer maximumLength;
private static final boolean nullable;
public LimitedString(String value, String descriptor, Integer maximumLength, Integer minimumLength, boolean isNullable) throws Exception {
if (minimumLength < 0) {
throw new IllegalArgumentException("Minimum length cannot be less than 0");
}
if (minimumLength > maximumLength) {
throw new IllegalArgumentException("Minimum length cannot be greater than maximum length");
}
this.minimumLength = minimumLength;
this.maximumLength = maximumLength;
this.nullable = isNullable;
this.descriptor = descriptor; // i.e. descriptor = "Contact First Name"
this.setValue(value);
}
LimitedString(String descriptor, Integer maximumLength, Integer minimumLength, boolean isNullable) {
if (minimumLength < 0) {
throw new IllegalArgumentException("Minimum length cannot be less than 0");
}
if (minimumLength > maximumLength) {
throw new IllegalArgumentException("Minimum length cannot be greater than maximum length");
}
this.minimumLength = minimumLength;
this.maximumLength = maximumLength;
this.nullable = isNullable;
this.descriptor = descriptor; // i.e. descriptor = "Contact First Name"
this.setValue(value);
}
public String ToString() {
return this.value;
}
public String getValue() {
return value;
}
public void setValue(String value) {
if (value == null && !nullable) throw new IllegalArgumentException("The value cannot be null.");
if (value.length() < minimumLength || value.length() > maximumLength) {
throw new IllegalArgumentException(String.format("@s must be between %s character(s) and %s characters long.", descriptor, minimumLength, maximumLength));
}
this.value = value;
}
public static String getDescriptor() {
return descriptor;
}
public static Integer getMinimumlength() {
return minimumLength;
}
public static Integer getMaximumlength() {
return maximumLength;
}
public static boolean isNullable() {
return nullable;
}
}
然后像這樣在聯系人 class 中使用它:
/**
*
*/
package Models;
/**
* @author Charles Hilton
*
*/
public class Contact {
private LimitedString firstName = new LimitedString("First Name", 10, 1, false);
private LimitedString lastName = new LimitedString("Last Name", 15, 1, false);
private Contact() {};
public Contact(String lastName, String firstName) {
this.setLastName(lastName);
this.setFirstName(firstName);
}
public String getFirstName() {
return firstName.getValue();
}
public void setFirstName(String firstName) {
this.firstName.setValue(firstName);
}
public String getLastName() {
return lastName.getValue();
}
public void setLastName(String lastName) {
this.lastName.setValue(lastName);
}
}
我擔心它可以更好地優化。 對於初學者,在 LimitedString 中。 我制作了幾個字段 static 和 final,目的是在將更多聯系人添加到集合中時盡量減少 memory 的使用,我顯然無法按照我現在設置的方式進行操作。
CharSequence
要直接回答您的問題,我建議編寫一個實現CharSequence
接口的 class。 String
、 StringBuilder
和其他一些類實現該接口。
如果您希望能夠向 class 的現有 object 添加更多文本,您的 class 也應該實現Appendable
。
我想你的 class 會包含一個String
。 如果可追加,可能是一個StringBuilder
。
我認為不需要您的descriptor
字段。
與其攜帶可為nullable
的字段,不如考慮編寫兩個類。 一個是另一個的子類,一個可以容忍 null,另一個不能。
Jakarta Bean Validation是滿足 null 檢查和限制字段大小等需求的更好解決方案。 見規格頁; 版本 3是最新的。 請參閱項目頁面(有點過時)。
提供了一些驗證器。 您可以編寫自己的驗證器。
您可以在代碼中調用驗證器。 驗證器可以通過內置於JavaFX或內置於Vaadin Flow的用戶界面調用。
我相信提供的驗證器NotNull
和Size
會實現您的目標。 但NotBlank
優於NotNull
,因為它還檢查非空文本是否包含至少一個非空白字符。
@NotBlank
@Size( min=1 , max=10 )
private String firstName ;
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.