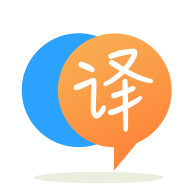
[英]Getting error in Apollo Express GraphQL: Error: Schema must contain uniquely named types but contains multiple types named "DateTime"
[英]Getting error in Express GraphQL: Schema must contain uniquely named types but contains multiple types named "String"
所以我試圖使用 GraphQL 創建一個 Pinterest 克隆,但我遇到了這個錯誤。
項目結構看起來像這樣
有 2 個模型,User 和 Pins
引腳型號
const mongoose = require('mongoose');
const pinSchema = new mongoose.Schema({
title: {
type: String,
required: [true, 'Title is Required'],
unique: [true, 'Title should be unique'],
},
imageUrl: {
type: String,
required: [true, 'Image URL is Required'],
},
description: {
type: String,
},
link: {
type: String,
},
userId: {
type: mongoose.Schema.Types.ObjectId, // to store which user
required: true,
},
createdAt: {
type: Date,
default: Date.now(),
},
});
const Pin = mongoose.model('Pin', pinSchema);
module.exports = Pin;
用戶模型
const mongoose = require('mongoose');
const userSchema = new mongoose.Schema({
name: {
type: String,
required: [true, 'Name is Required'],
},
userName: {
type: String,
required: [true, 'Username is Required'],
unique: [true, 'Username should be unique'],
},
email: {
type: String,
required: [true, 'Email is Required'],
unique: [true, 'Email should be unique'],
},
password: {
type: String,
required: [true, 'Password is Required'],
},
createdPins: {
type: [mongoose.Schema.Types.ObjectId], // store all pins created by this user
},
savedPins: {
type: [mongoose.Schema.Types.ObjectId], // store all pins saved by this user
},
});
const User = mongoose.model('User', userSchema);
module.exports = User;
Graphql 引腳類型
const { GraphQLObjectType, GraphQLID, GraphQLString } = require('graphql');
const User = require('../models/UserModel');
const PinType = new GraphQLObjectType({
name: 'Pin',
fields: () => ({
id: { type: GraphQLID },
title: { type: GraphQLString },
imageUrl: { type: GraphQLString },
description: { type: GraphQLString },
link: { type: GraphQLString },
user: {
type: UserType,
resolve(parent, args) {
return User.findById(parent.userId);
},
},
createdAt: { type: String },
}),
});
module.exports = PinType;
const UserType = require('./UserSchema');
Graphql 用戶類型
const {
GraphQLObjectType,
GraphQLID,
GraphQLString,
GraphQLList,
} = require('graphql');
const UserType = new GraphQLObjectType({
name: 'User',
fields: () => ({
id: { type: GraphQLID },
name: { type: GraphQLString },
userName: { type: GraphQLString },
createdPins: { type: new GraphQLList(PinType) },
savedPins: { type: new GraphQLList(PinType) },
}),
});
module.exports = UserType;
const PinType = require('./PinSchema');
查詢和變更:schema.js 文件
const {
GraphQLObjectType,
GraphQLList,
GraphQLID,
GraphQLSchema,
GraphQLNonNull,
GraphQLString,
} = require('graphql');
const User = require('../models/UserModel');
const Pin = require('../models/PinModel');
const UserType = require('./UserSchema');
const PinType = require('./PinSchema');
// Query
const RootQuery = new GraphQLObjectType({
name: 'RootQuery',
fields: {
// Get all Users
users: {
type: new GraphQLList(UserType),
resolve(parent, args) {
return User.find();
},
},
// Get a Single User
user: {
type: UserType,
args: { id: { type: GraphQLID } },
resolve(parent, args) {
return User.findById(args.id);
},
},
// Get all Pins
pins: {
type: new GraphQLList(PinType),
resolve(parent, args) {
return Pin.find();
},
},
// Get a Single Pin
pin: {
type: PinType,
args: { id: { type: GraphQLID } },
resolve(parent, args) {
return Pin.findById(args.id);
},
},
},
});
// Mutation
const Mutation = new GraphQLObjectType({
name: 'Mutation',
fields: {
// Create User
createUser: {
type: UserType,
args: {
name: { type: new GraphQLNonNull(GraphQLString) },
userName: { type: new GraphQLNonNull(GraphQLString) },
email: { type: new GraphQLNonNull(GraphQLString) },
password: { type: new GraphQLNonNull(GraphQLString) },
},
resolve(parent, args) {
return User.create({
name: args.name,
userName: args.userName,
email: args.email,
password: args.password,
});
},
},
// Delete User
deleteUser: {
type: UserType,
args: {
id: { type: new GraphQLNonNull(GraphQLID) },
},
resolve(parent, args) {
// delete all pins created by this user
Pin.find({ userId: args.id }).then((pins) => {
pins.forEach((pin) => {
pin.remove();
});
});
return User.findByIdAndRemove(args.id);
},
},
// Create a Pin
createPin: {
type: PinType,
args: {
title: { type: new GraphQLNonNull(GraphQLString) },
imageUrl: { type: new GraphQLNonNull(GraphQLString) },
description: { type: GraphQLString },
link: { type: GraphQLString },
userId: { type: new GraphQLNonNull(GraphQLID) },
},
resolve(parent, args) {
return Pin.create({
title: args.title,
imageUrl: args.imageUrl,
description: args.description,
link: args.link,
userId: args.userId,
});
},
},
// Update a Pin
updatePin: {
type: PinType,
args: {
id: { type: new GraphQLNonNull(GraphQLID) },
title: { type: GraphQLString },
imageUrl: { type: GraphQLString },
description: { type: GraphQLString },
link: { type: GraphQLString },
},
resolve(parent, args) {
return Pin.findByIdAndUpdate(
args.id,
{
$set: {
title: args.title,
imageUrl: args.imageUrl,
description: args.description,
link: args.link,
},
},
{ new: true }
);
},
},
// Delete a Pin
deletePin: {
type: PinType,
args: {
id: { type: new GraphQLNonNull(GraphQLID) },
},
resolve(parent, args) {
// remove this pin from the createdPins of the user
User.updateMany(
{},
{
$pullAll: {
createdPins: [args.id],
},
}
);
// delete this pin
return Pin.findByIdAndRemove(args.id);
},
},
},
});
const schema = new GraphQLSchema({
query: RootQuery,
mutation: Mutation,
});
module.exports = schema;
收到此錯誤
錯誤:架構必須包含唯一命名的類型,但包含多個名為“String”的類型。 在新的 GraphQLSchema (D:\Projects\Pinterest Clone\server\node_modules\graphql\type\schema.js:219:15) 在 Object.<anonymous> (D:\Projects\Pinterest Clone\server\schemas\schema.js :159:16) 在 Module._compile (node:internal/modules/cjs/loader:1149:14) 在 Module._extensions..js (node:internal/modules/cjs/loader:1203:10) 在 Module.load (node:internal/modules/cjs/loader:1027:32) 在 Module._load (node:internal/modules/cjs/loader:868:12) 在 Module.require (node:internal/modules/cjs/loader:1051 :19) at require (node:internal/modules/cjs/helpers:103:18) at Object.<anonymous> (D:\Projects\Pinterest Clone\server\index.js:6:16) at Module._compile (節點:內部/模塊/cjs/加載器:1149:14)
節點.js v18.10.0
試圖搜索這個,發現很多人都面臨類似的問題,但我無法解決。
發現了錯誤。 它在 Graphql PinType 文件中。 在定義架構時,我在導致錯誤的“createdAt”字段中使用了“String”而不是“GraphqlString”。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.