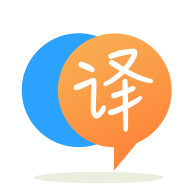
[英]Re-run JavasScript <script> code without refreshing the page
[英]Re-run javascript on a page without refreshing/reloading the entire page
我正在使用 JavaScript 生成一些隨機數。 然后根據用戶輸入檢查這些隨機數,並顯示正確/不正確的結果。 然后我有一個按鈕可以刷新頁面以生成新的隨機值。
我想知道是否有更好的方法可以重新運行 JavaScript 而無需再次重新加載整個頁面。
//Generates a pair of very peculiar two digit random numbers
function getRandomInt(min, max) {
min = Math.ceil(min);
max = Math.floor(max);
return Math.floor(Math.random() \* (max - min + 1)) + min;
}
let a = getRandomInt(2,9);
let b = getRandomInt(0,8);
let d = getRandomInt(1,a-1);
let e = getRandomInt(b+1,9);
let ab ="" + a + b;
document.getElementById("AB").innerHTML = ab;
let de ="" + d + e;
document.getElementById("DE").innerHTML = de;
// finds their difference
let result = ab-de;
document.getElementById("R").innerHTML = result;
//checks the user input against correct result and displays a correct/incorrect message
function myFunction() {
let x = document.getElementById("numb").value;
let text;
if (x == result) {
text = "Correct";
} else {
text = "incorrect";
}
document.getElementById("demo").innerHTML = text;
}
現在我希望新值在按下按鈕時出現。 一種方法是簡單地刷新我當前正在做的頁面。 我想知道是否有更好的方法來做到這一點。 我嘗試將整個腳本封裝在 function 中並使用按鈕調用它。 它有效,但由於某種原因它破壞了結果檢查功能。
PS:除了我在構建這個小項目時獲得的經驗外,我在 HTML/JS 方面沒有任何經驗。 非常感謝任何幫助。
您已經接近將所有內容放入另一個 function 的想法,但還不夠。 與其重新運行所有內容,另一個 function 只生成新數字並更新 UI 應該可以解決問題。
// Generates a pair of very peculiar two digit random numbers
function getRandomInt(min, max) {
min = Math.ceil(min);
max = Math.floor(max);
return Math.floor(Math.random() * (max - min + 1)) + min;
}
// Generate a new set of numbers
function getNewIntsToCheck() {
const a = getRandomInt(2,9);
const b = getRandomInt(0,8);
const d = getRandomInt(1,a-1);
const e = getRandomInt(b+1,9);
const ab ="" + a + b;
const de ="" + d + e;
const result = ab-de;
return { ab, de, result }
}
// Set in parent scope for access in myFunction
// There are other ways to do this, but this should be easy to understand since
// you don't have much experience with JS :)
let res = ""
// Update the UI on the click of the refresh button
function onRefreshButtonClick() {
const { ab, de, result } = getNewIntsToCheck()
// Set res from parent scope
res = result
document.getElementById("R").innerHTML = result;
document.getElementById("AB").innerHTML = ab;
document.getElementById("DE").innerHTML = de;
}
// Check current numbers in UI against user input and display message
function myFunction() {
let x = document.getElementById("numb").value;
let text;
// Check against res from parent scope, which was set in
// onRefreshButtonClick
if (x == res) {
text = "Correct";
} else {
text = "incorrect";
}
document.getElementById("demo").innerHTML = text;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.