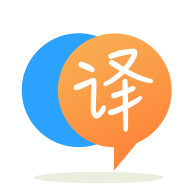
[英]Error working with structs and pointer arrays: incompatible types in assignment
[英]Incompatible pointer assignment error in C when implementing a stack with structs
我希望下面的詳細信息有所幫助,但總而言之......我正在嘗試使用結構來實現堆棧數據結構。 我在分配時只收到一個關於不兼容指針類型的錯誤,但是它們都是指向我的結構的單個指針。 但是,我確實有一個我無法理解的問題,我嘗試分配的指針類型(如下所示)的類型為'StackNode * (*)(int)'
,我似乎無法理解為什么,它應該只是StackNode *
。
有人有答案嗎? 謝謝你。
我給出的錯誤是...
Problem1.c:63:14: warning: assignment to ‘StackNode *’ from incompatible pointer type ‘StackNode * (*)(int)’ [-Wincompatible-pointer-types]
63 | root = newNode;//inserting at the front of the stack, so make sure to set our root node to point to newNode
我遇到問題的代碼塊是這個 function:
//This is a function that will create a new StackNode structure given some data and return pointer to the node
StackNode* newNode(int data){
StackNode* newNode = (StackNode*)malloc(sizeof(StackNode)); //allocate memory for the newly made stacknode
newNode->data = data; //set the data
newNode->next = NULL; //set the next node to null
return newNode;
}
我寫的完整代碼如下:
#include <stdio.h>
#include <stdlib.h>
#include <limits.h>
//type definition for each node in the queue
typedef struct StackNode{
int data;
struct StackNode* next;
}StackNode;
//function prototype definitions
int isEmpty(StackNode*);
int peek(StackNode*);
int pop(StackNode*);
void push(StackNode*, int);
StackNode* newNode(int data);
int main(){
int userInput = 0;
int validInput = 1;
StackNode *root = NULL;
while(validInput){
printf("Please enter a value to push into the stack");
scanf("%d", &userInput);
if(userInput>=0){
push(root, userInput);
printf("%d pushed to stack\n", userInput);//print the data we to the stack
}else{
break;
}
}
while(root!=NULL){
printf("%d was popped from the stack\n", root->data);
}
return 0;
}
int isEmpty(StackNode* root){
return !root; //NULL evaluates to false, so this returns whether it isnt null or not
}
//pushes a new node to the top of the stack by taking in data and using our function newNode to make a new node from the data.
//takes in a pointer to the root node since we want to adjust the stack.
void push(StackNode* root, int data){
StackNode* node = newNode(data); //create a new node from our data.
node->next = root;//insert at the front of the stack, so save the node root is pointing at and make newNode point there
root = newNode;//inserting at the front of the stack, so make sure to set our root node to point to newNode
}
//removes the first node and returns the value stored by it, makes sure to free the root node.
//It takes in a pointer to the root node since we want to free the space taken by the root node.
int pop(StackNode *root){
if(isEmpty(root)){
return INT_MIN; //return smallest possible integer since root doesnt exist
}
StackNode* temp = root; //sets a temporary node equal to the root node in order to free it after we are done
int poppedData = temp->data;//get the data from the root node
root = root->next;//set the root node to the next node in line
free(temp); //since we we no longer want the root node in memory, free it by freeing our temp node.
return poppedData; //return the data the root is storing.
}
//show the data stored at the top of the stack without removing the node itself, takes in pointer to root node
int peek(StackNode *root){
if(isEmpty(root)){
return INT_MIN; //return smallest possible integer since root doesnt exist
}
return root->data; //return the data the root is storing.
}
//This is a function that will create a new StackNode structure given some data and return pointer to the node
StackNode* newNode(int data){
StackNode* newNode = (StackNode*)malloc(sizeof(StackNode)); //allocate memory for the newly made stacknode
newNode->data = data; //set the data
newNode->next = NULL; //set the next node to null
return newNode;
}
在push
root = newNode;
newNode
是一個函數! 你可能是說
root=node;
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.