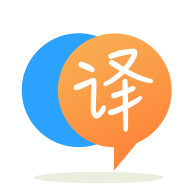
[英]How to force hibernate to do join while using @OneToOne mapping with @MapsId
[英]How can I join 3 entities in a table using JPA Hibernate without using @Mapsid
[this is my DB design](https://i.stack.imgur.com/7ckz4.png)
[Users entitie](https://i.stack.imgur.com/8mMfR.png)
[Roles entitie](https://i.stack.imgur.com/ACYNB.png)
[Project entitie](https://i.stack.imgur.com/ZJXKC.png)
Users 和 Has 之間的關系不需要是單向的
使用這個復合鍵類,我們可以創建實體類,它模擬連接表:
@Entity
class CourseRating {
@EmbeddedId
CourseRatingKey id;
@ManyToOne
@MapsId("studentId")
@JoinColumn(name = "student_id")
Student student;
@ManyToOne
@MapsId("courseId")
@JoinColumn(name = "course_id")
Course course;
int rating;
// standard constructors, getters, and setters
}
This code is very similar to a regular entity implementation. However, we have some key differences:
We used @EmbeddedId to mark the primary key, which is an instance of the CourseRatingKey class.
We marked the student and course fields with @MapsId.
@MapsId means that we tie those fields to a part of the key, and they're the foreign keys of a many-to-one relationship. We need it because, as we mentioned, we can't have entities in the composite key.
After this, we can configure the inverse references in the Student and Course entities as before:
class Student {
// ...
@OneToMany(mappedBy = "student")
Set<CourseRating> ratings;
// ...
}
class Course {
// ...
@OneToMany(mappedBy = "course")
Set<CourseRating> ratings;
// ...
}
請注意,還有一種使用復合鍵的替代方法:@IdClass 注釋。
3.4. 更多特征 我們將與 Student 和 Course 類的關系配置為 @ManyToOne。 我們可以這樣做是因為使用新實體,我們在結構上將多對多關系分解為兩個多對一關系。
為什么我們能夠做到這一點? 如果我們仔細檢查前一個案例中的表,我們可以看到它包含兩個多對一關系。 換句話說,RDBMS 中沒有任何多對多關系。 我們將使用連接表創建的結構稱為多對多關系,因為這就是我們建模的對象。
此外,如果我們談論多對多關系會更清楚,因為這是我們的意圖。 同時,連接表只是一個實現細節; 我們並不真正關心它。
此外,此解決方案還有一個我們尚未提及的附加功能。 簡單的多對多解決方案在兩個實體之間創建關系。 因此,我們無法將關系擴展到更多實體。 但是我們在這個解決方案中沒有這個限制:我們可以對任意數量的實體類型之間的關系進行建模。
例如,當多個教師可以教授一門課程時,學生可以評價特定教師教授特定課程的方式。 這樣一來,評級將成為三個實體之間的關系:學生、課程和教師。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.