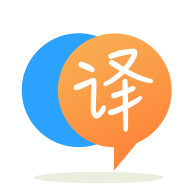
[英]Retrofit Post Request Error - java.lang.IllegalStateException: Expected BEGIN_OBJECT but was STRING at line 1 column 1 path $
[英]E/ERROR :: java.lang.IllegalStateException: Expected BEGIN_OBJECT but was STRING at line 1 column 2 path $
這是我第一次在這里提問,很抱歉我的英語不好。 每次我執行 POST 時都會收到此錯誤,而且我無法進行其他活動。 我不知道如何解決它。
E/ERROR :: java.lang.IllegalStateException: Expected BEGIN_OBJECT but was STRING at line 1 column 2 path $
這是我的模特
public class Experience implements Serializable {
@SerializedName("id")
@Expose
private String id;
@SerializedName("idKandidat")
@Expose
private String idKandidat;
@SerializedName("companyName")
@Expose
private String companyName;
@SerializedName("monthJoin")
@Expose
private Integer monthJoin;
@SerializedName("yearJoin")
@Expose
private Integer yearJoin;
@SerializedName("stillWorking")
@Expose
private Boolean stillWorking;
@SerializedName("monthResign")
@Expose
private Integer monthResign;
@SerializedName("yearResign")
@Expose
private Integer yearResign;
@SerializedName("posisionAs")
@Expose
private String posisionAs;
@SerializedName("descriptionJob")
@Expose
private String descriptionJob;
public Experience() {
}
public Experience(String id, String idKandidat, String companyName, Integer monthJoin, Integer yearJoin, Boolean stillWorking, Integer monthResign, Integer yearResign, String posisionAs, String descriptionJob) {
super();
this.id = id;
this.idKandidat = idKandidat;
this.companyName = companyName;
this.monthJoin = monthJoin;
this.yearJoin = yearJoin;
this.stillWorking = stillWorking;
this.monthResign = monthResign;
this.yearResign = yearResign;
this.posisionAs = posisionAs;
this.descriptionJob = descriptionJob;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public Experience withId(String id) {
this.id = id;
return this;
}
public String getIdKandidat() {
return idKandidat;
}
public void setIdKandidat(String idKandidat) {
this.idKandidat = idKandidat;
}
public Experience withIdKandidat(String idKandidat) {
this.idKandidat = idKandidat;
return this;
}
public String getCompanyName() {
return companyName;
}
public void setCompanyName(String companyName) {
this.companyName = companyName;
}
public Experience withCompanyName(String companyName) {
this.companyName = companyName;
return this;
}
public Integer getMonthJoin() {
return monthJoin;
}
public void setMonthJoin(Integer monthJoin) {
this.monthJoin = monthJoin;
}
public Experience withMonthJoin(Integer monthJoin) {
this.monthJoin = monthJoin;
return this;
}
public Integer getYearJoin() {
return yearJoin;
}
public void setYearJoin(Integer yearJoin) {
this.yearJoin = yearJoin;
}
public Experience withYearJoin(Integer yearJoin) {
this.yearJoin = yearJoin;
return this;
}
public Boolean getStillWorking() {
return stillWorking;
}
public void setStillWorking(Boolean stillWorking) {
this.stillWorking = stillWorking;
}
public Experience withStillWorking(Boolean stillWorking) {
this.stillWorking = stillWorking;
return this;
}
public Integer getMonthResign() {
return monthResign;
}
public void setMonthResign(Integer monthResign) {
this.monthResign = monthResign;
}
public Experience withMonthResign(Integer monthResign) {
this.monthResign = monthResign;
return this;
}
public Integer getYearResign() {
return yearResign;
}
public void setYearResign(Integer yearResign) {
this.yearResign = yearResign;
}
public Experience withYearResign(Integer yearResign) {
this.yearResign = yearResign;
return this;
}
public String getPosisionAs() {
return posisionAs;
}
public void setPosisionAs(String posisionAs) {
this.posisionAs = posisionAs;
}
public Experience withPosisionAs(String posisionAs) {
this.posisionAs = posisionAs;
return this;
}
public String getDescriptionJob() {
return descriptionJob;
}
public void setDescriptionJob(String descriptionJob) {
this.descriptionJob = descriptionJob;
}
public Experience withDescriptionJob(String descriptionJob) {
this.descriptionJob = descriptionJob;
return this;
}
}
這是我的服務
public interface ExperienceService {
@GET("DetailExperience/get")
Call<List<Experience>> getDetailEmergencies();
@GET("DetailExperience/getDetailExperience")
Call<List<Experience>> getDetailExperience();
@GET("DetailExperience/getDetailExperience/{id}")
Call<List<Experience>> getDetailExperience(@Path("id") String id);
@GET("DetailExperience/getSelectedExperience/{id}")
Call<List<Experience>> getSelectedExperience(@Path("id") String id);
@GET("DetailExperience/getById/{id}")
Call<List<Experience>> getById();
@Headers({"Accept: application/json"})
@POST("DetailExperience/save")
Call<Experience> SaveDetailExperience(@Body Experience experience);
@GET("DetailExperience/deleted/{id}")
Call<Experience> deleteDetailExperience(@Path("id") String id);
}
這是我的改造
public class APIUtils {
private APIUtils(){
}
public static final String API_URL = "http://192.168.100.7:2424/api/";
public static ExperienceService getExperienceService(){
return RetrofitClient.getClient(API_URL).create(ExperienceService.class);
}
}
public class RetrofitClient {
private static Retrofit retrofit = null;
public static Retrofit getClient(String url){
HttpLoggingInterceptor interceptor = new HttpLoggingInterceptor();
interceptor.setLevel(HttpLoggingInterceptor.Level.BODY);
OkHttpClient client = new OkHttpClient.Builder().addInterceptor(interceptor).build();
if (retrofit == null){
retrofit = new Retrofit.Builder().baseUrl(url)
.addConverterFactory(GsonConverterFactory.create())
.client(client)
.build();
}
return retrofit;
}
}
這是我的活動
package com.example.rekrutmenreka;
import androidx.appcompat.app.AppCompatActivity;
import androidx.appcompat.widget.SwitchCompat;
import android.app.DatePickerDialog;
import android.content.Intent;
import android.icu.text.Transliterator;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.CompoundButton;
import android.widget.DatePicker;
import android.widget.EditText;
import android.widget.ProgressBar;
import android.widget.Toast;
import com.example.rekrutmenreka.adapter.ExperienceAdapter;
import com.example.rekrutmenreka.model.Experience;
import com.example.rekrutmenreka.remote.APIUtils;
import com.example.rekrutmenreka.remote.ExperienceService;
import com.google.android.material.textfield.TextInputLayout;
import org.json.JSONObject;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.List;
import okhttp3.OkHttpClient;
import okhttp3.logging.HttpLoggingInterceptor;
import retrofit2.Call;
import retrofit2.Callback;
import retrofit2.Response;
public class SelectedExperienceActivity extends AppCompatActivity {
ExperienceService experienceService;
String IdKandidat,IdExperience,CompanyName,PosisionAs,DescriptionJob, MonthJoin,YearJoin,MonthResign,YearResign;
EditText edtIdKandidat,edtCompanyName,edtPosisionAs,edtDescriptionJob,edtMonthJoin,edtYearJoin,edtMonthResign,edtYearResign;
Boolean EdtStillWorking;
DatePickerDialog datePickerDialog;
TextInputLayout txtLayoutEditExperience6, txtLayoutEditExperience7;
SwitchCompat swEdtStillWorking;
Button btnUpdateExperience;
ProgressBar pbDataExperience;
private int mYear,mMonth,mDay;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_selected_experience);
HttpLoggingInterceptor interceptor = new HttpLoggingInterceptor();
interceptor.setLevel(HttpLoggingInterceptor.Level.BODY);
OkHttpClient okClient = new OkHttpClient.Builder().addInterceptor(interceptor).build();
Intent intent = getIntent();
IdExperience = intent.getStringExtra("IdExperience");
pbDataExperience = findViewById(R.id.pbEditDataExperience);
pbDataExperience.setVisibility(View.VISIBLE);
edtIdKandidat = findViewById(R.id.edtIdKandidat);
edtCompanyName = findViewById(R.id.edtCompanyName);
edtPosisionAs = findViewById(R.id.edtPosisionAs);
edtDescriptionJob = findViewById(R.id.edtDescriptionJob);
txtLayoutEditExperience6 = findViewById(R.id.txtLayoutEditExperience6);
txtLayoutEditExperience7 = findViewById(R.id.txtLayoutEditExperience7);
edtMonthJoin = findViewById(R.id.edtMonthJoin);
edtYearJoin = findViewById(R.id.edtYearJoin);
edtMonthResign = findViewById(R.id.edtMonthResign);
edtYearResign = findViewById(R.id.edtYearResign);
swEdtStillWorking = findViewById(R.id.swEdtStillWorking);
loadData();
edtMonthJoin.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
final Calendar c = Calendar.getInstance();
mYear = c.get(Calendar.YEAR); // current year
mMonth = c.get(Calendar.MONTH); // current month
mDay = c.get(Calendar.DAY_OF_MONTH); // current day
// date picker dialog
datePickerDialog = new DatePickerDialog(SelectedExperienceActivity.this,
new DatePickerDialog.OnDateSetListener() {
@Override
public void onDateSet(DatePicker view, int year,
int monthOfYear, int dayOfMonth) {
// set day of month , month and year value in the edit text
/*addMonthJoin.setText(dayOfMonth + "/"
+ (monthOfYear + 1) + "/" + year);*/
edtMonthJoin.setText(String.valueOf(monthOfYear + 1));
edtYearJoin.setText(String.valueOf(year));
}
}, mYear, mMonth, mDay);
datePickerDialog.show();
}
});
edtYearJoin.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
final Calendar c = Calendar.getInstance();
mYear = c.get(Calendar.YEAR); // current year
mMonth = c.get(Calendar.MONTH); // current month
mDay = c.get(Calendar.DAY_OF_MONTH); // current day
// date picker dialog
datePickerDialog = new DatePickerDialog(SelectedExperienceActivity.this,
new DatePickerDialog.OnDateSetListener() {
@Override
public void onDateSet(DatePicker view, int year,
int monthOfYear, int dayOfMonth) {
// set day of month , month and year value in the edit text
/*addMonthJoin.setText(dayOfMonth + "/"
+ (monthOfYear + 1) + "/" + year);*/
edtMonthJoin.setText(String.valueOf(monthOfYear + 1));
edtYearJoin.setText(String.valueOf(year));
}
}, mYear, mMonth, mDay);
datePickerDialog.show();
}
});
edtMonthResign.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
final Calendar c = Calendar.getInstance();
mYear = c.get(Calendar.YEAR); // current year
mMonth = c.get(Calendar.MONTH); // current month
mDay = c.get(Calendar.DAY_OF_MONTH); // current day
// date picker dialog
datePickerDialog = new DatePickerDialog(SelectedExperienceActivity.this,
new DatePickerDialog.OnDateSetListener() {
@Override
public void onDateSet(DatePicker view, int year,
int monthOfYear, int dayOfMonth) {
// set day of month , month and year value in the edit text
/*addMonthJoin.setText(dayOfMonth + "/"
+ (monthOfYear + 1) + "/" + year);*/
edtMonthResign.setText(String.valueOf(monthOfYear + 1));
edtYearResign.setText(String.valueOf(year));
}
}, mYear, mMonth, mDay);
datePickerDialog.show();
}
});
edtYearResign.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
final Calendar c = Calendar.getInstance();
mYear = c.get(Calendar.YEAR); // current year
mMonth = c.get(Calendar.MONTH); // current month
mDay = c.get(Calendar.DAY_OF_MONTH); // current day
// date picker dialog
datePickerDialog = new DatePickerDialog(SelectedExperienceActivity.this,
new DatePickerDialog.OnDateSetListener() {
@Override
public void onDateSet(DatePicker view, int year,
int monthOfYear, int dayOfMonth) {
// set day of month , month and year value in the edit text
/*addMonthJoin.setText(dayOfMonth + "/"
+ (monthOfYear + 1) + "/" + year);*/
edtMonthResign.setText(String.valueOf(monthOfYear + 1));
edtYearResign.setText(String.valueOf(year));
}
}, mYear, mMonth, mDay);
datePickerDialog.show();
}
});
swEdtStillWorking.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
if (!isChecked){
txtLayoutEditExperience6.setVisibility(View.VISIBLE);
txtLayoutEditExperience7.setVisibility(View.VISIBLE);
}else{
txtLayoutEditExperience6.setVisibility(View.INVISIBLE);
txtLayoutEditExperience7.setVisibility(View.INVISIBLE);
}
}
});
btnUpdateExperience = findViewById(R.id.btnUpdateExperience);
btnUpdateExperience.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Experience experience = new Experience();
IdKandidat = edtIdKandidat.getText().toString();
CompanyName = edtCompanyName.getText().toString();
PosisionAs = edtPosisionAs.getText().toString();
DescriptionJob = edtDescriptionJob.getText().toString();
MonthJoin = edtMonthJoin.getText().toString();
YearJoin = edtYearJoin.getText().toString();
EdtStillWorking = swEdtStillWorking.isChecked();
MonthResign = edtMonthResign.getText().toString();
YearResign = edtYearResign.getText().toString();
if (CompanyName.trim().equals("")){
edtCompanyName.setError("Tidak Boleh Kosong");
}else if(PosisionAs.trim().equals("")){
edtPosisionAs.setError("Tidak Boleh Kosong");
}else if(DescriptionJob.trim().equals("")){
edtDescriptionJob.setError("Tidak Boleh Kosong");
}else if(MonthJoin.trim().equals("")){
edtMonthJoin.setError("Tidak Boleh Kosong");
}else if(YearJoin.trim().equals("")){
edtYearJoin.setError("Tidak Boleh Kosong");
}else if(!EdtStillWorking){
if (MonthResign.trim().equals("")){
edtMonthResign.setError("Tidak Boleh Kosong");
}
if (YearResign.trim().equals("")){
edtYearResign.setError("Tidak Boleh Kosong");
}
}
experience.setId(IdExperience);
experience.setIdKandidat(IdKandidat);
experience.setCompanyName(CompanyName);
experience.setMonthJoin(Integer.parseInt(MonthJoin));
experience.setYearJoin(Integer.parseInt(YearJoin));
experience.setStillWorking(EdtStillWorking);
if (EdtStillWorking){
experience.setMonthResign(0);
experience.setYearResign(0);
}else{
experience.setMonthResign(Integer.parseInt(MonthResign));
experience.setYearResign(Integer.parseInt(YearResign));
}
experience.setPosisionAs(PosisionAs);
experience.setDescriptionJob(DescriptionJob);
experienceService = APIUtils.getExperienceService();
Call<Experience> call = experienceService.SaveDetailExperience(experience);
call.enqueue(new Callback<Experience>() {
@Override
public void onResponse(Call<Experience> call, Response<Experience> response) {
if (response.isSuccessful()){
Toast.makeText(SelectedExperienceActivity.this, "Data Berhasil Disimpan", Toast.LENGTH_LONG).show();
Intent intent = new Intent(SelectedExperienceActivity.this, DetailExperienceActivity.class);
startActivity(intent);
}
}
@Override
public void onFailure(Call<Experience> call, Throwable t) {
Log.e("ERROR : ", t.getMessage());
}
});
/*insertData(experience);*/
}
});
}
private void loadData() {
experienceService = APIUtils.getExperienceService();
Call<List<Experience>> call = experienceService.getSelectedExperience(IdExperience);
call.enqueue(new Callback<List<Experience>>() {
@Override
public void onResponse(Call<List<Experience>> call, Response<List<Experience>> response) {
if (response.isSuccessful()){
List<Experience> listEdit = response.body();
edtIdKandidat.setText(listEdit.get(0).getIdKandidat());
edtCompanyName.setText(listEdit.get(0).getCompanyName());
edtPosisionAs.setText(listEdit.get(0).getPosisionAs());
edtDescriptionJob.setText(listEdit.get(0).getDescriptionJob());
edtMonthJoin.setText(Integer.toString(listEdit.get(0).getMonthJoin()));
edtYearJoin.setText(Integer.toString(listEdit.get(0).getYearJoin()));
swEdtStillWorking.setChecked(listEdit.get(0).getStillWorking());
edtMonthResign.setText(Integer.toString(listEdit.get(0).getMonthResign()));
edtYearResign.setText(Integer.toString(listEdit.get(0).getYearResign()));
pbDataExperience.setVisibility(View.INVISIBLE);
}
}
@Override
public void onFailure(Call<List<Experience>> call, Throwable t) {
Log.e("ERROR : ", t.getMessage());
pbDataExperience.setVisibility(View.INVISIBLE);
}
});
}
}
我嘗試通過 POSTMAN 發送,結果成功。
這是日志記錄
2022-12-14 21:40:19.843 552-692/system_process D/InputDispatcher: Waiting to send key to Window{cfe608e u0 com.example.rekrutmenreka/com.example.rekrutmenreka.SelectedExperienceActivity} because there are unprocessed events that may cause focus to change
2022-12-14 21:40:20.191 552-692/system_process D/InputDispatcher: Waiting to send key to Window{cfe608e u0 com.example.rekrutmenreka/com.example.rekrutmenreka.SelectedExperienceActivity} because there are unprocessed events that may cause focus to change
2022-12-14 21:40:21.250 552-692/system_process D/InputDispatcher: Waiting to send key to Window{cfe608e u0 com.example.rekrutmenreka/com.example.rekrutmenreka.SelectedExperienceActivity} because there are unprocessed events that may cause focus to change
2022-12-14 21:40:21.371 6662-6720/com.example.rekrutmenreka D/OkHttp: --> POST http://192.168.100.7:2424/api/DetailExperience/save
2022-12-14 21:40:21.371 6662-6720/com.example.rekrutmenreka D/OkHttp: Content-Type: application/json; charset=UTF-8
2022-12-14 21:40:21.372 6662-6720/com.example.rekrutmenreka D/OkHttp: Content-Length: 289
2022-12-14 21:40:21.372 6662-6720/com.example.rekrutmenreka D/OkHttp: Accept: application/json
2022-12-14 21:40:21.377 6662-6720/com.example.rekrutmenreka D/OkHttp: {"companyName":"PT Mencari Cinta Sejati","descriptionJob":"Belajar Coding","id":"1063860e-e213-4d13-8f41-132e87558ffd","idKandidat":"29be4f5e-50a2-4bdd-b929-c9e796c6b7c3","monthJoin":12,"monthResign":12,"posisionAs":"Staff Marketing","stillWorking":false,"yearJoin":2020,"yearResign":2022}
2022-12-14 21:40:21.377 6662-6720/com.example.rekrutmenreka D/OkHttp: --> END POST (289-byte body)
2022-12-14 21:40:21.479 6662-6720/com.example.rekrutmenreka D/OkHttp: <-- 200 OK http://192.168.100.7:2424/api/DetailExperience/save (101ms)
2022-12-14 21:40:21.480 6662-6720/com.example.rekrutmenreka D/OkHttp: Transfer-Encoding: chunked
2022-12-14 21:40:21.480 6662-6720/com.example.rekrutmenreka D/OkHttp: Content-Type: application/json; charset=utf-8
2022-12-14 21:40:21.480 6662-6720/com.example.rekrutmenreka D/OkHttp: Server: Microsoft-IIS/10.0
2022-12-14 21:40:21.480 6662-6720/com.example.rekrutmenreka D/OkHttp: X-Powered-By: ASP.NET
2022-12-14 21:40:21.480 6662-6720/com.example.rekrutmenreka D/OkHttp: Date: Wed, 14 Dec 2022 14:40:22 GMT
2022-12-14 21:40:21.484 6662-6720/com.example.rekrutmenreka D/OkHttp: "Data Success"
2022-12-14 21:40:21.484 6662-6720/com.example.rekrutmenreka D/OkHttp: <-- END HTTP (14-byte body)
2022-12-14 21:40:21.489 6662-6662/com.example.rekrutmenreka E/ERROR :: java.lang.IllegalStateException: Expected BEGIN_OBJECT but was STRING at line 1 column 2 path $
顯然,對DetailExperience/save
的 HTTP 請求不會返回 JSON 對象,這是預期的:
public void onResponse(Call<Experience> call, Response<Experience> response)
這期望 POST 請求返回一個 JSON,其中包含 Experience 對象的數據。
但是日志消息說,端點返回一個帶引號的字符串:
2022-12-14 21:40:21.484 6662-6720/com.example.rekrutmenreka D/OkHttp: "Data Success"
可能的修復可能是:
public void onResponse(Call<String> call, Response<String> response)
這是一個 json 序列化/反序列化問題,值是對象,但反序列化是字符串。
Data Success
是一個字符串,不是一個對象,你需要改變接收類型或者改變后端返回值不要返回一個
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.