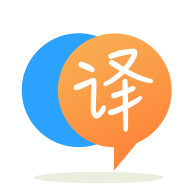
[英]How to use (variable of type gorm.io/gorm.DB) as github.com/jinzhu/gorm.DB?
[英]cannot use mockDB (variable of type *MockDB) as *gorm.DB value in struct literal
我創建了一個 get function 用於從 postgres 數據庫中獲取練習。 我寫了模擬測試,但是我從結構中得到了這個錯誤,我該如何修復它?
我使用了 Handler struct 它有 *gorm.DB 結構。
錯誤:
不能使用 mockDB(*MockDB 類型的變量)作為結構文字中的 *gorm.DB 值
// router
package exercises
import (
"github.com/gin-gonic/gin"
"gorm.io/gorm"
)
type Handlers struct {
DB *gorm.DB
}
func RegisterRoutes(router *gin.Engine, db *gorm.DB) {
h := &Handlers{
DB: db,
}
routes := router.Group("/exercises")
routes.POST("/", h.AddExercise)
routes.GET("/", h.GetExercises)
routes.GET("/:id", h.GetExercise)
routes.PUT("/:id", h.UpdateExercise)
routes.DELETE("/:id", h.DeleteExercise)
}
// test
package exercises
import (
"net/http"
"net/http/httptest"
"testing"
"github.com/gin-gonic/gin"
"github.com/kayraberktuncer/sports-planner/pkg/common/models"
"github.com/stretchr/testify/mock"
"gorm.io/gorm"
)
type MockDB struct {
mock.Mock
}
func (m *MockDB) Find(value interface{}) *gorm.DB {
args := m.Called(value)
return args.Get(0).(*gorm.DB)
}
func (m *MockDB) Error() error {
args := m.Called()
return args.Error(0)
}
func TestGetExercises(t *testing.T) {
// Setup mock DB
mockDB := new(MockDB)
mockDB.On("Find", &[]models.Exercise{}).Return(mockDB).Once()
// Setup Gin router
router := gin.New()
router.GET("/", func(c *gin.Context) {
handlers := &Handlers{DB: mockDB} // error
handlers.GetExercises(c)
})
// Perform request
w := httptest.NewRecorder()
req, _ := http.NewRequest("GET", "/", nil)
router.ServeHTTP(w, req)
// Assert response
if w.Code != http.StatusOK {
t.Errorf("Expected status code %d, got %d", http.StatusOK, w.Code)
}
// Assert mock DB was called correctly
mockDB.AssertExpectations(t)
}
我想用我的處理程序結構進行模擬測試
MockDB
和 gorm 的DB
是兩個不同的結構,不能互換使用。 如果它們實現相同的接口,它們可以在同一個地方使用。 例如:
// router
package exercises
import (
"github.com/gin-gonic/gin"
"gorm.io/gorm"
)
// this interface will be implemented by gorm.DB struct
type Store interface {
Create(value interface{}) *gorm.DB
First(out interface{}, where ...interface{}) *gorm.DB
Model(value interface{}) *gorm.DB
Delete(value interface{}, where ...interface{}) *gorm.DB
Find(out interface{}, where ...interface{}) *gorm.DB
DB() *sql.DB
Raw(sql string, values ...interface{}) *gorm.DB
Exec(sql string, values ...interface{}) *gorm.DB
Where(query interface{}, args ...interface{}) *gorm.DB
//other method signatures
}
type Handlers struct {
DB Store
}
func RegisterRoutes(router *gin.Engine, db Store) {
h := &Handlers{
DB: db,
}
routes := router.Group("/exercises")
routes.POST("/", h.AddExercise)
routes.GET("/", h.GetExercises)
routes.GET("/:id", h.GetExercise)
routes.PUT("/:id", h.UpdateExercise)
routes.DELETE("/:id", h.DeleteExercise)
}
現在,您可以將*gorm.DB
傳遞給代碼中的RegisterRoutes
function。 對於測試,如果MockDB
結構實現了Store
接口中的所有方法,則可以使用它。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.