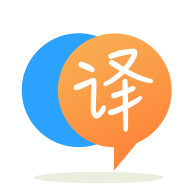
[英]Swapping an Objects value, with a value from another object. *Javascript*
[英]Getting max and min value from array of objects and returning another value associated with the same object. JavaScript
我在完成我正在參加的教育課程的任務時遇到問題,highestValShoe 和 lowestValShoe 函數返回的結果相同。
我已盡力而為,但我不明白我哪里出錯了。 我真的很感激一些指示。 謝謝!
//First I will establish an empty array to push() the shoes in later.
shoeArray = [];
//Now I will create a class for the shoes.
class Shoes {
constructor(name, productCode, quantity, valuePerItem) {
this.name = name;
this.productCode = productCode;
this.quantity = quantity;
this.valuePerItem = valuePerItem;
}
//This code will enable us to update the quantity.
updateQuantity(newQuantity) {
this.quantity = newQuantity;
}
}
//Now I will create 5 instances for the class.
converse = new Shoes("Converse", 010405, 3, 55.5);
adidas = new Shoes("Adidas", 030602, 5, 85.0);
nike = new Shoes("Nike", 052656, 2, 165.0);
vans = new Shoes("Vans", 745023, 6, 95.5);
fila = new Shoes("Fila", 034567, 3, 45.0);
//This will push all instances into the shoeArray.
shoeArray.push(converse, adidas, nike, vans, fila);
//This function will enable us to search for any shoe within the array.
function searchShoes(shoeName, shoeArray) {
for (i = 0; i < shoeArray.length; i++) {
if (shoeArray[i].name === shoeName) {
return shoeArray[i];
}
}
}
//This function will enable us to search for the lowest value shoe.
function lowestValShoe (shoeArray) {
for (i=0; i<shoeArray.length; i++){
lowestVal = Math.min(shoeArray[i].valuePerItem)
shoe = shoeArray[i].name
}
return shoe
}
//This function will enable us to search for the highest value shoe.
function highestValShoe (shoeArray){
for (i=0; i<shoeArray.length; i++){
highestVal = Math.max(shoeArray[i].valuePerItem)
shoe1 = shoeArray[i].name
}
return shoe1
}
我試圖返回最大值“Shoe”和最小值“Shoe”,當我測試 lowestValShoe function 時我認為它有效,但是當我將其轉換為最大值時,它返回相同的值。
我更改了其中一只鞋的數值,將另一只鞋設為最低值,然后意識到我的最低值 ValShoe function 也沒有按預期工作。
這兩個函數都返回 'Fila'
您需要存儲 object 而不是僅用於查找最小值或最大值鞋的名稱。
取第一項並從第二項迭代到結束。 然后檢查值是更大還是更小並拿走該項目。 稍后僅返回名稱。
function highestValShoe(shoeArray) {
let shoe = shoeArray[0];
for (let i = 1; i < shoeArray.length; i++) {
if (shoeArray[i].valuePerItem > shoe.valuePerItem) shoe = shoeArray[i];
}
return shoe.name;
}
順便說一句,請聲明所有變量。 如果沒有,你會得到全局的,這可能會導致有趣的結果。 並使用分號。 總是。
//Now I will create a class for the shoes. class Shoes { constructor(name, productCode, quantity, valuePerItem) { this.name = name; this.productCode = productCode; this.quantity = quantity; this.valuePerItem = valuePerItem; } //This code will enable us to update the quantity. updateQuantity(newQuantity) { this.quantity = newQuantity; } } //This function will enable us to search for any shoe within the array. function searchShoes(shoeName, shoeArray) { for (let i = 0; i < shoeArray.length; i++) { if (shoeArray[i].name === shoeName) return shoeArray[i]; } } //This function will enable us to search for the lowest value shoe. function lowestValShoe(shoeArray) { let shoe = shoeArray[0]; for (let i = 1; i < shoeArray.length; i++) { if (shoeArray[i].valuePerItem < shoe.valuePerItem) shoe = shoeArray[i]; } return shoe.name; } function highestValShoe(shoeArray) { let shoe = shoeArray[0]; for (let i = 1; i < shoeArray.length; i++) { if (shoeArray[i].valuePerItem > shoe.valuePerItem) shoe = shoeArray[i]; } return shoe.name; } //Now I will create 5 instances for the class. const shoeArray = [], converse = new Shoes("Converse", "010405", 3, 55.5), adidas = new Shoes("Adidas", "030602", 5, 85.0), nike = new Shoes("Nike", "052656", 2, 165.0), vans = new Shoes("Vans", "745023", 6, 95.5), fila = new Shoes("Fila", "034567", 3, 45.0); //This will push all instances into the shoeArray. shoeArray.push(converse, adidas, nike, vans, fila); console.log(lowestValShoe(shoeArray)); console.log(highestValShoe(shoeArray));
因為你需要重寫你的 min 和 max 函數,所以他們不會只使用 Math.min 和 Math.max 你可以使用Array.reduce
使它們成為一個班輪:
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/reduce
function lowestValShoe (shoeArray) {
return shoeArray.reduce((prev, curr) => (prev.valuePerItem < curr.valuePerItem ? prev : curr));
}
function highestValShoe (shoeArray){
return shoeArray.reduce((prev, curr) => (prev.valuePerItem > curr.valuePerItem ? prev : curr));
}
在這里你可以看到它分別返回Fila
和Nike
的最小值和最大值:
//First I will establish an empty array to push() the shoes in later. shoeArray = []; //Now I will create a class for the shoes. class Shoes { constructor(name, productCode, quantity, valuePerItem) { this.name = name; this.productCode = productCode; this.quantity = quantity; this.valuePerItem = valuePerItem; } //This code will enable us to update the quantity. updateQuantity(newQuantity) { this.quantity = newQuantity; } } //Now I will create 5 instances for the class. converse = new Shoes("Converse", 010405, 3, 55.5); adidas = new Shoes("Adidas", 030602, 5, 85.0); nike = new Shoes("Nike", 052656, 2, 165.0); vans = new Shoes("Vans", 745023, 6, 95.5); fila = new Shoes("Fila", 034567, 3, 45.0); //This will push all instances into the shoeArray. shoeArray.push(converse, adidas, nike, vans, fila); console.log(lowestValShoe(shoeArray)); console.log(highestValShoe(shoeArray)); function lowestValShoe(shoeArray) { return shoeArray.reduce((prev, curr) => (prev.valuePerItem < curr.valuePerItem? prev: curr)); } function highestValShoe(shoeArray){ return shoeArray.reduce((prev, curr) => (prev.valuePerItem > curr.valuePerItem? prev: curr)); }
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.