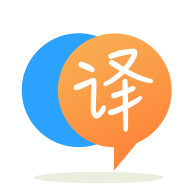
[英]How to add dropdown selected value to sharepoint lookup column in sharepoint list
[英]How to add a check icon with MUI or Tailwind CSS to all the selected value in the dropdown list?
我想在值旁邊添加一個小的復選/勾選圖標,例如:操作 ✓ 如果用戶在 TopicList 下拉列表中選擇操作。 TopicList 是一個 class 組件,用於從數據庫中獲取數據,其中包括:Operations、Array、Repetition、Function、Selection。 如果用戶選擇 2 個不同的值,則例如:操作 ✓ 數組 ✓ 重復 Function 選擇。 如何修改此代碼以解決此問題? 這是我希望用戶選擇 Array 時的樣子的圖像。
import axios from "axios";
import React, { useState } from "react";
import TopicList from "../Components/topicList";
import CheckIcon from '@mui/icons-material/Check';
function CreateEvent(success, message) {
const navigate = useNavigate();
const [selectedValues, setSelectedValues] = useState([]); // array to store selected values
const getDataFromTopicList = (val) => {
if (val !== "" && !selectedValues.includes(val)) {
setSelectedValues([...selectedValues, val]);
}
};
const handleSubmit = (event) => {
event.preventDefault();
console.log(selectedValues); // selectedValues array contains all selected values from all TopicList components
axios({
method: "POST",
url: BASE_URL + "events/submitEvent",
data: {
topicId: selectedValues,
},
headers: { "Content-Type": "application/json" },
})
.then((response) => {
if (response.status === 200) {
toast.success("Successfully Created", {
position: toast.POSITION.TOP_CENTER,
});
} else {
toast.error(response.data.message, {
position: toast.POSITION.TOP_CENTER,
});
}
})
.catch((err) => {
if (err.response) {
toast.error(err.response.data.message, {
position: toast.POSITION.TOP_CENTER,
});
} else {
toast.error("Failed to Create", {
position: toast.POSITION.TOP_CENTER,
});
}
});
};
return (
<div className="">
<div>
<form
onSubmit={handleSubmit}
>
<h1>
Create an Event
</h1>
<div>
<div>
<TopicList
selectedValues={selectedValues}
getDataFromTopicList={getDataFromTopicList}
/>
</div>
</div>
</form>
</div>
</div>
);
}
export default CreateEvent;
import axios from "axios";
import React from "react";
import CheckIcon from "@mui/icons-material/Check";
const BASE_URL = process.env.REACT_APP_BASE_URL;
export default class TopicList extends React.Component {
state = {
topics: [],
};
componentDidMount() {
axios.get(BASE_URL + `events/topic`).then((res) => {
const topics = res.data;
this.setState({ topics });
});
}
render() {
return (
<select required onChange={(val) => this.getCat(val.target.value)}>
{this.state.topics.map((topic) => (
<option value={topic.topicId}>
{topic.topic}
{this.props.selectedValues.includes(topic.topicId) && <CheckIcon style={{ color: "green" }} />}
</option>
))}
</select>
);
}
}
似乎發布的代碼具有 MUI 但未在TopicList
中使用其組件。
這是一個使用 MUI Select
的基本示例,並在多個選定值上實現了所需的CheckIcon
。
以下示例的最小化演示: stackblitz
該示例為簡單起見省略了數據獲取部分,但它保留了相同的topics
state 並為TopicList
中的selectedValues
獲取道具。
selectedValues
的更新 function 也針對 MUI 組件進行了更改,但 state 值本身仍然是所選topicId
的數組,因此希望它可以連接起來以獲取數據,而無需對現有代碼進行大量重構。
TopicList
中 MUI 組件的導入:
import OutlinedInput from '@mui/material/OutlinedInput';
import InputLabel from '@mui/material/InputLabel';
import MenuItem from '@mui/material/MenuItem';
import FormControl from '@mui/material/FormControl';
import ListItemText from '@mui/material/ListItemText';
import ListItemIcon from '@mui/material/ListItemIcon';
import Select from '@mui/material/Select';
import CheckIcon from '@mui/icons-material/Check';
修改后的 TopicList 的TopicList
:
<FormControl sx={{ m: 3, width: 300 }}>
<InputLabel id="topic-form-input-label">Topic</InputLabel>
<Select
labelId="topic-form-label"
id="multiple-topic-select"
multiple
value={this.props.selectedValues}
onChange={this.props.onChange}
input={<OutlinedInput label="Topic" />}
renderValue={(selected) =>
selected
.map((topicId) => {
const selectedTopic = this.state.topics.find(
(item) => item.topicId === topicId
);
return selectedTopic ? selectedTopic.topic : topicId;
})
.join(", ")
}
>
{this.state.topics.map((topic) => {
const selected = this.props.selectedValues.includes(topic.topicId);
return (
<MenuItem key={topic.topicId} value={topic.topicId}>
{selected && (
<ListItemIcon>
<CheckIcon style={{ color: "green" }} />
</ListItemIcon>
)}
<ListItemText inset={!selected} primary={topic.topic} />
</MenuItem>
);
})}
</Select>
</FormControl>
從父組件傳遞的選定值:
const [selectedValues, setSelectedValues] = useState([]);
const handleChange = (event) => {
const {
target: { value },
} = event;
setSelectedValues(typeof value === "string" ? value.split(",") : value);
};
<TopicList
selectedValues={selectedValues}
onChange={handleChange}
/>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.