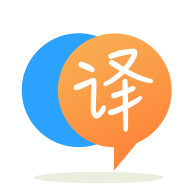
[英]How to map JSON object with java Pojo class ignoring parent class spring boot?
[英]How to map JSON array to Java class in Spring Boot
我正在嘗試從另一個 API 獲得響應,將其轉換為 Java 類,然后使用 Spring Boot 發送到前端 我有來自外部 API 的 JSON 響應,就像這樣
{
"status": {
"timestamp": "2023-01-31T14:06:45.210Z",
"error_code": 0,
"error_message": null,
},
"data": [
{
"id": 7982,
"name": "wc4qtz6py1i",
"tags": [
"40rcevshzab",
"3ja25pufu0z"
],
"quote": {
"USD": {
"price": 0.2,
},
"BTC": {
"price": 7159,
}
}
},
{
"id": 8742,
"name": "uhso98ca",
"tags": [
"84jsjsaesxx",
"sasdd5dda76"
],
"quote": {
"USD": {
"price": 6,
},
"BTC": {
"price": 1230,
}
}
}
]
}
我需要使用 Spring Boot 將所有這些轉換為類。 但是我應該如何組織呢? 最多的問題是關於“數據”數組。 現在我有這樣的東西。
@AllArgsConstructor
@NoArgsConstructor
@Getter
@Setter
public class MainDTO{
@JsonProperty("status")
private Status status;
@JsonProperty("data")
private Data data;
}
@AllArgsConstructor
@NoArgsConstructor
@Getter
@Setter
public class Data {
Coin[] coins;
}
@AllArgsConstructor
@NoArgsConstructor
@Getter
@Setter
public class Coin{
@JsonProperty("id")
private Integer id;
@JsonProperty("name")
private String name;
private Map<String, Coin> quote;
}
但它不起作用。 我有錯誤:
I have error
Tue Jan 31 16:13:45 EET 2023
There was an unexpected error (type=Internal Server Error, status=500).
Error while extracting response for type [class com.example.myCoolApp.entity.MainDTO] and content type [application/json;charset=utf-8]
org.springframework.web.client.RestClientException: Error while extracting response for type [class com.example.myCoolApp.entity.CryptoDTO] and content type [application/json;charset=utf-8]
您不需要 Data class 因為 json 中的數據字段不是 object - 它是一個對象數組,在您的例子中它是一個Coin對象數組。
改變它的下一種方式:
public class MainDTO{
@JsonProperty("status")
private Status status;
@JsonProperty("data")
private List<Coin> data;
}
值得一提的是,您的示例中的 JSON 由於有額外的逗號,因此不正確。 我認為正確的形式應該是這樣的:
{
"status": {
"timestamp": "2023-01-31T14:06:45.210Z",
"error_code": 0,
"error_message": null
},
"data": [
{
"id": 7982,
"name": "wc4qtz6py1i",
"tags": [
"40rcevshzab",
"3ja25pufu0z"
],
"quote": {
"USD": {
"price": 0.2
},
"BTC": {
"price": 7159
}
}
},
{
"id": 8742,
"name": "uhso98ca",
"tags": [
"84jsjsaesxx",
"sasdd5dda76"
],
"quote": {
"USD": {
"price": 6
},
"BTC": {
"price": 1230
}
}
}
]
}
至於課程,我會建議你下一個:
@AllArgsConstructor
@NoArgsConstructor
@Getter
@Setter
public class MainDTO {
@JsonProperty("status")
private Status status;
@JsonProperty("data")
private List<Coin> coins;
}
@AllArgsConstructor
@NoArgsConstructor
@Getter
@Setter
public class Status {
@JsonProperty("timestamp")
private String timestamp;
@JsonProperty("error_code")
private Integer errorCode;
@JsonProperty("error_message")
private String errorMessage;
}
@AllArgsConstructor
@NoArgsConstructor
@Getter
@Setter
public class Coin {
@JsonProperty("id")
private Integer id;
@JsonProperty("name")
private String name;
@JsonProperty("tags")
private List<String> tags;
@JsonProperty("quote")
private Map<String, Quote> quote;
}
@AllArgsConstructor
@NoArgsConstructor
@Getter
@Setter
public class Quote {
@JsonProperty("price")
private Double price;
}
JSON
響應中的“數據”數組是一個硬幣數組,因此MainDTO
class 中的硬幣屬性應該是List<Coin>
類型。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.