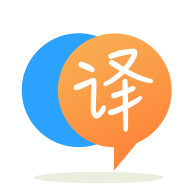
[英]Programatically closing currently displayed Java Swing JDialog box
[英]How do I get a reference to the currently displayed Java Swing JDialog box?
我正在使用基於Java Swing的框架,該框架使用Java Swing JDialog框顯示彈出消息。 我需要獲得它們的參考,以便以編程方式關閉它們。 該框架創建對話框,但不保留對它們的引用。 我可以參考當前顯示的框嗎?
謝謝。
嘗試這個 :
import java.awt.Window;
import javax.swing.JDialog;
public class Test {
public static void main(String[] args) {
JDialog d = new JDialog((Window)null,"Demo Dialog");
for (Window w : JDialog.getWindows()) {
if ( w instanceof JDialog) {
System.out.println(((JDialog)w).getTitle());
}
}
}
}
如果您對以下內容感到困惑:
(Window)null
強制轉換,只需嘗試在沒有它的情況下進行編譯:)
(順便說一句,將空窗口傳遞給構造函數會創建一個未擁有的對話框)
編輯:請注意,這將為您提供對所有對話框的引用,無論它們是否如代碼所示都是可見的。 要解決此問題,只需使用isVisible()查詢對話框是否可見
如果要獲取對最前面的JDialog的引用,則必須偵聽AWT事件並“實時”更新此引用並將其存儲在某個地方。
這是我在自己的Swing框架中所做的事情:
public class ActiveWindowHolder
{
public DefaultActiveWindowHolder()
{
_current = null;
Toolkit.getDefaultToolkit().addAWTEventListener(new AWTEventListener()
{
public void eventDispatched(AWTEvent e)
{
DefaultActiveWindowHolder.this.eventDispatched(e);
}
}, AWTEvent.WINDOW_EVENT_MASK);
}
public Window getActiveWindow()
{
return _current;
}
private void eventDispatched(AWTEvent e)
{
switch (e.getID())
{
case WindowEvent.WINDOW_ACTIVATED:
_current = ((WindowEvent) e).getWindow();
break;
case WindowEvent.WINDOW_DEACTIVATED:
_current = null;
break;
default:
// Do nothing (we are only interested in activation events)
break;
}
}
private Window _current;
}
只需確保在顯示任何組件之前在主線程中實例化ActiveWindowHandler
; ActiveWindowHandler.getActiveWindow()
起,每次對ActiveWindowHandler.getActiveWindow()
調用都將返回最前面的窗口( JFrame
JDialog
)。
您可以使用Window類上的getWindows()方法獲得對所有子窗口的引用。
public static Window[] getWindows()
Returns an array of all Windows, both owned and ownerless, created by this application. If called from an applet, the array includes only the Windows accessible by that applet.
請注意,我懷疑返回的窗口之一將是您的頂層框架/窗口。 您可以檢查窗口屬性(例如標題)以確定要關閉的對話框。
還有更具體的版本:getOwnedWindows()和getOwnerlessWindows()取決於您的需求。
我沒有辦法知道當前作為框架的子框架打開的內容-我只看到獲取父框架的方法。
您可以更改當前類以調用對話框嗎? 如果是這樣,您可以添加一個容器來跟蹤事物的打開和關閉,並添加一些訪問該容器的方法。
窗口w = KeyboardFocusManager.getCurrentKeyboardFocusManager()。getFocusedWindow();
然后,您可以檢查並將其投射到JDialog。
但是就像我在上一個問題中所說的那樣-您必須更改方法。
如果您顯式創建JDialog
而不是使用JOptionPane.showXXX
您將已經具有對該對話框的引用。 這絕對是我首選的方法; 例如
JDialog dlg = new JDialog(null, "My Dialog", false); // Create non-modal dialog.
dlg.setLayout(new BorderLayout());
dlg.add(myPanel, BorderLayout.CENTER);
dlg.pack();
dlg.setLocationRelativeTo(null);
dlg.setVisible(true);
您可以將對話框設置為模態對話框,這使您的生活變得非常輕松...
或者,在初始化JDialog時,請執行以下操作:
JDialog d = new JDialog();
d.addWindowListener(new WindowAdapter(){
public void windowClosed(WindowEvent e){
dialogClosedAlertFunction(); //goes wherever you want it to go
}
});
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.