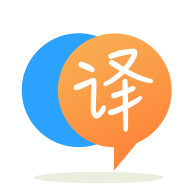
[英]How can I attach an event listener to an element that has itself been inserted with Javascript? (without jQuery)
[英]jQuery - How can I temporarily disable the onclick event listener after the event has been fired?
事件被觸發后,如何暫時禁用onclick事件監聽器(jQuery首選)?
例:
用戶單擊按鈕並在下面觸發此函數后,我想禁用onclick偵聽器,因此不會向我的django視圖發出相同的命令。
$(".btnRemove").click(function(){
$(this).attr("src", "/url/to/ajax-loader.gif");
$.ajax({
type: "GET",
url: "/url/to/django/view/to/remove/item/" + this.id,
dataType: "json",
success: function(returned_data){
$.each(returned_data, function(i, item){
// do stuff
});
}
});
非常感謝,
阿爾
有很多方法可以做到這一點。 例如:
$(".btnRemove").click(function() {
var $this = $(this);
if ($this.data("executing")) return;
$this
.data("executing", true)
.attr("src", "/url/to/ajax-loader.gif");
$.get("/url/to/django/view/to/remove/item/" + this.id, function(returnedData) {
// ... do your stuff ...
$this.removeData("executing");
});
});
要么
$(".btnRemove").click(handler);
function handler() {
var $this = $(this)
.off("click", handler)
.attr("src", "/url/to/ajax-loader.gif");
$.get("/url/to/django/view/to/remove/item/" + this.id, function(returnedData) {
// ... do your stuff ...
$this.click(handler);
});
}
我們還可以使用事件委派來獲得更清晰的代碼和更好的性能:
$(document).on("click", ".btnRemove:not(.unclickable)", function() {
var $this = $(this)
.addClass("unclickable")
.attr("src", "/url/to/ajax-loader.gif");
$.get("/url/to/django/view/to/remove/item/" + this.id, function(returnedData) {
// ... do your stuff ...
$this.removeClass("unclickable");
});
});
如果我們不需要在執行后重新啟用處理程序,那么我們可以使用.one()
方法。 它綁定僅執行一次的處理程序。 請參閱jQuery文檔: http : //api.jquery.com/one
您要在多長時間內禁用click事件偵聽器? 一種方法是使用jQuery的unbind
http://docs.jquery.com/Events/unbind取消綁定事件監聽器。
但最好的做法是不要將事件解除綁定只是為了以后重新綁定它。 請改用布爾值。
var active = true;
$(".btnRemove").click(function() {
if (!active) {
return;
}
active = false;
$(this).attr("src", "/url/to/ajax-loader.gif");
$.ajax({
type: "GET",
url: "/url/to/django/view/to/remove/item/" + this.id,
dataType: "json",
success: function(returned_data) {
active = true; // activate it again !
$.each(returned_data, function(i, item) {
// do stuff
});
}
});
});
編輯:為了安全起見,你還應該關心其他ajax完成例程(只有三個: success
, error
, complete
查看文檔 )或者其他active
可能保持錯誤。
為什么不禁用按鈕?您想要單獨禁用此列表器的任何特定原因? BTB,從您的代碼中,我看到您正在進行ajax調用。 所以你特別想阻止用戶,直到電話回來? 如果是,你可以試試一個jQuery插件blockUI
我會設置一個全局變量來跟蹤AJAX請求......
var myApp = {
ajax: null
}
然后有一點魔力來阻止同步請求......
// Fired when an AJAX request begins
$.ajaxStart(function() { myApp.ajax = 1 });
// Fired when an AJAX request completes
$.ajaxComplete(function() { myApp.ajax = null });
// Fired before an AJAX request begins
$.ajaxSend(function(e, xhr, opt) {
if(myApp.ajax != null) {
alert("A request is currently processing. Please wait.");
xhr.abort();
}
});
使用這種方法,您不必返回代碼並修改每個AJAX調用。 (我稱之為“附加”解決方案)
您可以根據布爾值在單擊內執行操作。 單擊它時,更改布爾值並使用setTimeout()在幾秒鍾后將其更改回來。 這將有效地限制用戶每隔幾秒鍾僅點擊一次按鈕。
var isEnabled = true;
$("a.clickMe").click(function(e){
e.preventDefault();
if (isEnabled == true) {
isEnabled = false; // disable future clicks for now
do_Something();
setTimeout(function(){
isEnabled = true;
}, 3000); // restore functionality after 3 seconds
}
});
我會使用一個類,例如'ajax-running'。 只有當被點擊的元素沒有'ajax-running'類時,才會執行click事件。 一旦你的ajax調用完成,你就可以刪除'ajax-running'類,這樣就可以再次點擊它。
$(".btnRemove").click(function(){
var $button = $(this);
var is_ajaxRunning = $button.hasClass('ajax-running');
if( !is_ajaxRunning ){
$.ajax({
...
success: function(returned_data) {
...
$button.removeClass('ajax-running');
});
};
}
});
我建議禁用該按鈕,然后在Ajax完成例程中重新啟用它(成功或失敗,請記住)。 如果您擔心瀏覽器不尊重您的禁用按鈕,您可以在按鈕上使用您自己的標志來支持它(例如,設置一個名為data-disabled
的屬性,使用data-
前綴作為良好做法並兼容HTML5 )。 但是,除非實際上遇到瀏覽器沒有禁用按鈕的問題,我可能會認為這已經足夠了。
var ajaxAction = function() {
var ele = $(this);
ele.unbind("click", ajaxAction);
ele.attr("src", "/url/to/ajax-loader.gif");
$.ajax({
type: "GET",
url: "/url/to/django/view/to/remove/item/" + this.id,
dataType: "json",
success: function(returned_data) {
$.each(returned_data, function(i, item) {
});
},
complete: function() {
ele.bind("click", ajaxAction);
}
});
}
$(".btnRemove").click(ajaxAction);
如果您通過ajax發布,則可以在單擊時禁用該按鈕,並在該過程完成后啟用它。 但如果您要回發,則不能只禁用該按鈕。 禁用該按鈕會導致服務器端單擊事件不會觸發。 所以只需在點擊時隱藏按鈕並顯示用戶友好的消息。 關於如何在回發時禁用asp.net按鈕的帖子有幫助。
你也可以隱藏按鈕(或包含div)
$(".btnRemove").click(function() {
$(this).hide();
// your code here...
})
如果你需要再次顯示它,你可以調用.show()。
另外,根據您的使用情況,您應該考慮使用加載覆蓋
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.