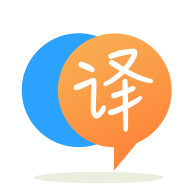
[英]How can I query all records with timestamp older than 30 days using cURL?
[英]How can I tell if a file is older than 30 minutes from /bin/sh?
如何編寫腳本來確定 /bin/sh 中的文件是否超過 30 分鍾?
不幸的是,系統中不存在stat
命令。 這是一個舊的 Unix 系統, http://en.wikipedia.org/wiki/Interactive_Unix
不幸的是,系統上沒有安裝 Perl,客戶也不想安裝它,也沒有別的。
這是使用find
的一種方法。
if test "`find file -mmin +30`"
如果find
問題的文件包含空格或特殊字符,則必須引用find
命令。
以下以秒為單位為您提供文件年齡:
echo $(( `date +%s` - `stat -L --format %Y $filename` ))
這意味着這應該為超過 30 分鍾的文件提供真/假值 (1/0):
echo $(( (`date +%s` - `stat -L --format %Y $filename`) > (30*60) ))
30*60
60——一分鍾60秒,不要預先計算,讓CPU為你做事!
如果您正在編寫 sh 腳本,最有用的方法是將test
與已經提到的 stat 技巧一起使用:
if [ `stat --format=%Y $file` -le $(( `date +%s` - 1800 )) ]; then
do stuff with your 30-minutes-old $file
fi
請注意, [
是test
的符號鏈接(或等效的); 請參閱man test
,但請記住, test
和[
也是 bash 內置函數,因此行為可能略有不同。 (另請注意[[
bash 復合命令)。
好吧,沒有統計數據和殘缺的發現。 這是您的替代方案:
編譯GNU coreutils以獲得不錯的 find(以及許多其他方便的命令)。 您可能已經將它作為 gfind 使用了。
如果-r
有效,也許您可以使用date
來獲取文件修改時間?
(`date +%s` - `date -r $file +%s`) > (30*60)
或者,使用-nt
比較來選擇哪個文件較新,麻煩的是制作一個修改時間為 30 分鍾過去的文件。 touch
通常可以做到這一點,但所有的賭注都是可用的。
touch -d '30 minutes ago' 30_minutes_ago
if [ your_file -ot 30_minutes_ago ]; then
...do stuff...
fi
最后,看看 Perl 是否可用,而不是糾結於誰知道 shell 實用程序的版本。
use File::stat;
print "Yes" if (time - stat("yourfile")->mtime) > 60*30;
對於像我這樣不喜歡倒勾的人,根據@slebetman 的回答:
echo $(( $(date +%s) - $(stat -L --format %Y $filename) > (30*60) ))
您可以通過與您創建的時間戳為 30 分鍾前的參考文件進行比較來完成此操作。
首先通過輸入創建您的比較文件
touch -t YYYYMMDDhhmm.ss /tmp/thirty_minutes_ago
用三十分鍾前的值替換時間戳。 您可以使用 Perl 中的一個簡單的單行代碼自動執行此步驟。
然后使用 find 的 newer 運算符通過否定搜索運算符來匹配較舊的文件
find . \! -newer /tmp/thirty_minutes_ago -print
超過 30 分鍾是什么意思:修改超過 30 分鍾,或創建超過 30 分鍾? 希望是前者,因為到目前為止的答案對於該解釋是正確的。 在后一種情況下,您會遇到問題,因為 unix 文件系統不跟蹤文件的創建時間。 ( ctime
文件屬性記錄inode內容上次更改的時間,即發生了chmod
或chown
事情)。
如果你真的需要知道文件是否是在 30 分鍾前創建的,你要么必須使用find
東西重復掃描文件系統的相關部分,或者使用一些平台相關的東西,比如 linux 的inotify 。
這是我對find
的變化:
if [ `find cache/nodes.csv -mmin +10 | egrep '.*'` ]
Find 除非失敗,否則總是返回狀態碼0
; 但是, egrep
返回1
是沒有找到匹配項`。 因此,如果該文件早於 10 分鍾,則此組合將通過。
試試看:
touch /tmp/foo; sleep 61;
find /tmp/foo -mmin +1 | egrep '.*'; echo $?
find /tmp/foo -mmin +10 | egrep '.*'; echo $?
應該在文件路徑后打印 0 和 1。
我的功能使用這個:
## Usage: if isFileOlderThanMinutes "$NODES_FILE_RAW" $NODES_INFO_EXPIRY; then ...
function isFileOlderThanMinutes {
if [ "" == "$1" ] ; then serr "isFileOlderThanMinutes() usage: isFileOlderThanMinutes <file> <minutes>"; exit; fi
if [ "" == "$2" ] ; then serr "isFileOlderThanMinutes() usage: isFileOlderThanMinutes <file> <minutes>"; exit; fi
## Does not exist -> "older"
if [ ! -f "$1" ] ; then return 0; fi
## The file older than $2 is found...
find "$1" -mmin +$2 | egrep '.*' > /dev/null 2>&1;
if [ $? == 0 ] ; then return 0; fi ## So it is older.
return 1; ## Else it not older.
}
#!/usr/bin/ksh
## this script creates a new timer file every minute and renames all the previously created timer files and then executes whatever script you need which can now use the timer files to compare against with a find. The script is designed to always be running on the server. The first time the script is executed it will remove the timer files and it will take an hour to rebuild them (assuming you want 60 minutes of timer files)
set -x
# if the server is rebooted for any reason or this scripts stops we must rebuild the timer files from scratch
find /yourpath/timer -type f -exec rm {} \;
while [ 1 ]
do
COUNTER=60
COUNTER2=60
cd /yourpath/timer
while [ COUNTER -gt 1 ]
do
COUNTER2=`expr $COUNTER - 1`
echo COUNTER=$COUNTER
echo COUNTER2=$COUNTER2
if [ -f timer-minutes-$COUNTER2 ]
then
mv timer-minutes-$COUNTER2 timer-minutes-$COUNTER
COUNTER=`expr $COUNTER - 1`
else
touch timer-minutes-$COUNTER2
fi
done
touch timer-minutes-1
sleep 60
#this will check to see if the files have been fully updated after a server restart
COUNT=`find . ! -newer timer-minutes-30 -type f | wc -l | awk '{print $1}'`
if [ $COUNT -eq 1 ]
then
# execute whatever scripts at this point
fi
done
myfile.txt 的當前時間和上次修改時間之間的差異(以秒為單位):
echo $(($(date +%s)-$(stat -c "%Y" myfile.txt)))
您還可以在命令stat -c
使用%X
或%Z
來獲取上次訪問或上次狀態更改之間的差異,檢查是否返回 0!
%X time of last access, seconds since Epoch
%Y time of last data modification, seconds since Epoch
%Z time of last status change, seconds since Epoch
測試:
if [ $(($(date +%s)-$(stat -c "%Y" myfile.txt))) -lt 600 ] ; then echo younger than 600 sec ; else echo older than 600 sec ; fi
if [[ "$(date --rfc-3339=ns -r /tmp/targetFile)" < "$(date --rfc-3339=ns --date '90 minutes ago')" ]] ; then echo "older"; fi
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.