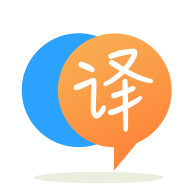
[英]Java: split array list into chunks where objects have an equal attribute value
[英]Find objects in a list where some attributes have equal values
給定一個對象列表(所有相同的類型),我如何確保它只包含某個屬性的每個值的一個元素,即使equals()可能因為檢查更多屬性而為這些元素返回false? 在代碼中:
private void example() {
List<SomeType> listWithDuplicates = new ArrayList<SomeType>();
/*
* create the "duplicate" objects. Note that both attributes passed to
* the constructor are used in equals(), though for the purpose of this
* question they are considered equal if the first argument was equal
*/
SomeType someObject1 = new SomeObject1("hello", "1");
SomeType someObject2 = new SomeObject1("hello", "2");
List<SomeType> listWithoutDuplicates = removeDuplicates(listWithDuplicates)
//listWithoutDuplicates should not contain someObject2
}
private List<SomeType> removeDuplicates(List<SomeType> listWithDuplicates) {
/*
* remove all but the first entry in the list where the first constructor-
* arg was the same
*/
}
可以使用Set作為中間占位符來查找Bozho建議的重復項。 這是一個示例removeDuplicates()
實現。
private List<SomeType> removeDuplicates(List<SomeType> listWithDuplicates) {
/* Set of all attributes seen so far */
Set<AttributeType> attributes = new HashSet<AttributeType>();
/* All confirmed duplicates go in here */
List duplicates = new ArrayList<SomeType>();
for(SomeType x : listWithDuplicates) {
if(attributes.contains(x.firstAttribute())) {
duplicates.add(x);
}
attributes.add(x.firstAttribute());
}
/* Clean list without any dups */
return listWithDuplicates.removeAll(duplicates);
}
也許HashMap可以像這樣使用:
private List<SomeType> removeDuplicates(List<SomeType> listWithDuplicates) {
/*
* remove all but the first entry in the list where the first constructor-
* arg was the same
*/
Iterator<SomeType> iter = listWithDuplicates.iterator();
Map<String, SomeType> map = new HashMap<String, SomeType>();
while(iter.hasnext()){
SomeType i = iter.next();
if(!map.containsKey(i.getAttribute())){
map.put(i.getAttribute(), i);
}
}
//At this point the map.values() is a collection of objects that are not duplicates.
}
如果equals()
是合適的,我可以推薦一些“標准”集合類/方法。 事實上,我認為你唯一的選擇就是
在首先檢查原始列表中的所有前面元素是否重復之后,將每個元素復制到另一個列表; 要么
從列表中刪除您在之前位置發現重復的任何元素。 對於列表內刪除,您最好使用LinkedList
,其中刪除不是那么昂貴。
在任何一種情況下,檢查重復項將是O(n ^ 2)操作,唉。
如果您要進行大量此類操作,則可能值得將列表元素包裝在另一個類中,該類根據您自己定義的條件返回哈希碼。
我會看一下像這樣的實現Comparator
接口。 如果您希望使用一個或兩個簡單的屬性進行比較,那么這非常簡單。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.